add a closing bracket at the end.
Also, use code styler, it really helps
results after using styler and adding a closing bracket. Errors exploded. Any
//+------------------------------------------------------------------+ //| Global variables | //+------------------------------------------------------------------+ // Scalping settings double ScalpThreshold = 0.0; double StopLoss = 0.0; double TakeProfit = 0.0; double Point = 0.0; // Trade tracking variables int buyTicket = 0; int sellTicket = 0; double openPrice = 0.0; double dailyProfit = 0.0; bool dailyProfitThresholdReached = false; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { // Reset the daily profit variable dailyProfit = 0.0; // Reset the daily profit threshold reached flag dailyProfitThresholdReached = false; // Return success return (INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { // Reset the daily profit variable dailyProfit = 0.0; // Reset the daily profit threshold reached flag dailyProfitThresholdReached = false; } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ int OnTick() { // Check if the daily profit threshold has been reached if(dailyProfitThresholdReached) { // If the daily profit threshold has been reached, close any open trades and return if(buyTicket > 0) { OrderClose(buyTicket, OrderLots(), Bid, 10, Violet); } if(sellTicket > 0) { OrderClose(sellTicket, OrderLots(), Ask, 10, Violet); } return; } // Set the default leverage for the trade int leverage = 100; // Check if the spread is less than the scalp threshold and there are no open orders if(SymbolInfoDouble(Symbol(), SYMBOL_SPREAD) < ScalpThreshold && buyTicket == 0 && sellTicket == 0) { // Open a buy order buyTicket = OrderSend(Symbol(), OP_BUY, 1.0, Ask, 10, 0, 0, "scalp-buy", leverage, 0, Green); // Set the stop loss and take profit for the buy order OrderModify(buyTicket, Ask - StopLoss * Point, Ask + TakeProfit * Point, 0, Blue); // Set the open price for the buy order openPrice = Ask; } // Check if there is a buy order open if(buyTicket > 0) { // Check if the order has hit the take profit or the market has moved in favor of the order if(OrderProfit() > 0 || Ask > openPrice + TrailingStop * Point) { // If either condition is true, close the order and reset the buy ticket variable OrderClose(buyTicket, OrderLots(), Bid, 10, Violet); buyTicket = 0; // Update the daily profit variable dailyProfit += OrderProfit(); // Check if the daily profit has reached or exceeded the threshold if(dailyProfit >= 50) { // If the daily profit has reached or exceeded the threshold, set the daily profit threshold reached flag to true // and return dailyProfitThresholdReached = true; return; } } } // Check if the spread is less than the scalp threshold and there are no open orders if(SymbolInfoDouble(Symbol(), SYMBOL_SPREAD) < ScalpThreshold && buyTicket == 0 && sellTicket == 0) { // Open a sell order sellTicket = OrderSend(Symbol(), OP_SELL, 1.0, Bid, 10, 0, 0, "scalp-sell", leverage, 0, Green); // Set the stop loss and take profit for the sell order OrderModify(sellTicket, Bid + StopLoss * Point, Bid - TakeProfit * Point, 0, Blue); // Set the open price for the sell order openPrice = Bid; } // Check if there is a sell order open if(sellTicket > 0) { // Check if the order has hit the take profit or the market has moved in favor of the order if(OrderProfit() > 0 || Bid < openPrice - TrailingStop * Point) { // If either condition is true, close the order and reset the sell ticket variable OrderClose(sellTicket, OrderLots(), Ask, 10, Violet); sellTicket = 0; // Update the daily profit variable dailyProfit += OrderProfit(); // Check if the daily profit has reached or exceeded the threshold if(dailyProfit >= 50) { // If the daily profit has reached or exceeded the threshold, set the daily profit threshold reached flag to true // and return dailyProfitThresholdReached = true; return; } } } //+------------------------------------------------------------------+
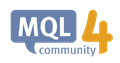
- docs.mql4.com
- 1193ped #: results after usin g styler and adding a closing bracket. Errors exploded. Any
Please edit your post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor -
You are missing the closing bracket for OnTick
-
Please edit your post and use the CODE button (or Alt+S)! (For large amounts of code, attach it.)
General rules and best pratices of the Forum. - General - MQL5 programming forum (2019)
Messages Editor -
You are missing the closing bracket for OnTick
apologies danke
i dont understand where t he missing bracket needs to be placed. Can you direct me?
bool dailyProfitThresholdReached = false; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { // Reset the daily profit variable dailyProfit = 0.0; // Reset the daily profit threshold reached flag dailyProfitThresholdReached = false; // Return success return (INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { // Reset the daily profit variable dailyProfit = 0.0; // Reset the daily profit threshold reached flag dailyProfitThresholdReached = false; } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ int OnTick() { // Check if the daily profit threshold has been reached if(dailyProfitThresholdReached) { // If the daily profit threshold has been reached, close any open trades and return if(buyTicket > 0) { OrderClose(buyTicket, OrderLots(), Bid, 10, Violet); } if(sellTicket > 0) { OrderClose(sellTicket, OrderLots(), Ask, 10, Violet); } return; } } } }
That is not what you originally posted.
The missing bracket was at the end of your original post.
no luck added it at end, and more error popped up. can you help me refactor. its my first EA. I am a below avg coding skills
Ive attached the text file
these are the errors with the bracket at the end. 78 in total. I am heartbroken,
without bracket at end , its just 2 errors, once i add bracket it turns into 78 errors. frustrating
without bracket at end , its just 2 errors, once i add bracket it turns into 78 errors. frustrating
-
Luck is not involved. Fix the first error, compile, repeat.
-
int OnTick()
Read the documentation. OnTick is a void function.
-
if(dailyProfitThresholdReached) {
Where is the closing brace?
-
Don't change the file extension. It is MQ5!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I don't understand these errors, I turned on highlighting brackets and I don't understand what is causing the two errors, so close yet so far away.
*******