All you need to know you'll find here:
https://www.metatrader5.com/en/metaeditor/help/development/debug // Code debugging
https://www.mql5.com/en/articles/2041 // Error Handling and Logging in MQL5
https://www.mql5.com/en/articles/272 // Tracing, Debugging and Structural Analysis of Source Code
https://www.mql5.com/en/articles/35 // scrol down to: "Launching and Debuggin"
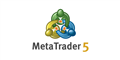
- www.metatrader5.com
I am new to programming, I wrote this program to place buystops and modify them at intervals if not triggered or close them after some time if triggered. Who can help me get it working?
This code contains dozens of errors - the first thing to do is use the compiler and fix all the syntax errors.
It helps if you compile regularly while typing to avoid ending up with a large number of errors at the end, as we see here.
I suggest you comment out sections of code so you can handle this in a bite-sized manner - all part of the journey in learning how to program

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am new to programming, I wrote this program to place buystops and modify them at intervals if not triggered or close them after some time if triggered. Who can help me get it working?