I coded a function where it checks if a certain symbol has a hidden bullish or bearish divergence. It is filtered with a 200 EMA wherein it only looks for bullish hidden divergence if price is above EMA and looks for bearish hidden divergence if price is below EMA.
What do you think behind the logic of my codes? Is there a way to optimize it?
I think it's fine. 👍
If you want, you can create an enum and set the function return value to a type of that enum. This way, instead of checking for 0, 1, or 2, you can check for a programmer-friendly value.
Example:
enum HIDDEN_DIVERGENCE_TYPE { NO_HIDDEN_DIVERGENCE, HIDDEN_BULLISH_DIVERGENCE, HIDDEN_BEARISH_DIVERGENCE }; HIDDEN_DIVERGENCE_TYPE hiddenDivergence(int BarsShift) //BarsShift is the shift in bars { double currLowRSI = iRSI(NULL,0,14,0,iLowest(NULL,0,3,10,0)); //Current RSI (Lowest in the last 10 bars) double currHighRSI = iRSI(NULL,0,14,0,iHighest(NULL,0,3,10,0)); //Current RSI (Highest in the last 10 bars) double lowestRSI = iRSI(NULL,0,14,0,iLowest(NULL,0,1,BarsShift,0)); //Lowest RSI in last x Bars double highestRSI = iRSI(NULL,0,14,0,iHighest(NULL,0,2,BarsShift,0)); //Highest RSI in last x Bars double currPriceLow = iLow(NULL,0,iLowest(NULL,0,1,10,0)); //Lowest price in the last 10 bars double currPriceHigh = iHigh(NULL,0,iHighest(NULL,0,2,10,0)); //Highest price in the last 10 bars double lowestPrice = iLow(NULL,0,iLowest(NULL,0,1,BarsShift,0)); //Lowest price in last x Bars double highestPrice = iHigh(NULL,0,iHighest(NULL,0,2,BarsShift,0)); //Highest price in last x Bars double valueMA = iMA(NULL,0,200,0,1,0,0); //Value of the 200 EMA if(Ask > valueMA && currPriceLow > lowestPrice && lowestRSI > currLowRSI) { Print("Hidden Bullish Divergence"); return HIDDEN_BULLISH_DIVERGENCE; } else if(Ask < valueMA && currPriceHigh < highestPrice && highestRSI < currHighRSI) { Print("Hidden Bearish Divergence"); return HIDDEN_BEARISH_DIVERGENCE; } else { Print("No Divergence"); return NO_HIDDEN_DIVERGENCE; } }
Side note: your iHighest/iLowest and iMA calls check for the bar that's currently open. If this is what you want, that's fine, but it's worth noting as the results can change because the bar has yet to close.
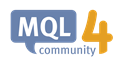
- docs.mql4.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I coded a function where it checks if a certain symbol has a hidden bullish or bearish divergence. It is filtered with a 200 EMA wherein it only looks for bullish hidden divergence if price is above EMA and looks for bearish hidden divergence if price is below EMA.
What do you think behind the logic of my codes? Is there a way to optimize it?