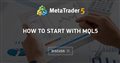
- 2021.12.30
- www.mql5.com
Yes, but you didn't provide the "iUniOsc" code used, or a link to your specific version.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problem
I provided the full code of the Indicator (but not the underlying sub routines). I get that the 3 buffers are osc (0), buy (1) and sell (2), but I cannot seem to find the right syntax in the EA to pick them up properly (as they show in the data window). In the data window, buy=osc for bullish signal, sell=osc for bearish signals, an are Null the rest of the time. When pulled into the EA, values never Nul out.
William
Appreciate the assist. The iUniOsc is too large for the forum. I did not think it was relevant, since the buffer calls are from the code above (Osc, Buy, Sell), however, as noted in the data window, buy=osc for bullish signal, sell=osc for bearish signals, an are Null the rest of the time. When pulled into the EA, values never Null out. I cannot seen to find the right syntax for the EA.
Thanks
Yes, but you didn't provide the "iUniOsc" code used, or a link to your specific version.
How To Ask Questions The Smart Way. (2004)
Be precise and informative about your problem

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Newbie here. I have an custom indicator that I cannot for the life of me, figure out how to access the Buy/Sell signals from an EA. Code for the indicator is below. I've tried every variation of CopyBuffer from the EA, and cannot seem to find the right syntax. Any assistance of what that CopyBuffer looks like to grab the Buy and Sell from below would be greatly appreciated!