- Colour code chart tabs
- Suggestions, comments, errors on the Signals service
- Know when a trade closes
No need to store it, just go through history and find it.
MT5: first select a position via CPositionInfo, directly, or by 'MT4Orders' library (2016)
MT4:
-
Do not assume history has only closed orders.
OrderType() == 6, 7 in the history pool? - MQL4 programming forum (2017) -
Do not assume history is ordered by date, it's not.
Could EA Really Live By Order_History Alone? (ubzen) - MQL4 programming forum (2012)
Taking the last profit and storing it in a variable | MQL4 - MQL4 programming forum #3 (2020.06.08) -
Total Profit is OrderProfit() + OrderSwap() + OrderCommission(). Some brokers don't use the Commission/Swap fields. Instead, they add balance entries. (Maybe related to Government required accounting/tax laws.)
"balance" orders in account history - Day Trading Techniques - MQL4 programming forum (2017)Broker History FXCM Commission - <TICKET>
Rollover - <TICKET>>R/O - 1,000 EUR/USD @0.52 #<ticket> N/A OANDA Balance update
Financing (Swap: One entry for all open orders.)
You'd want to start by defining "most recent" — is it "most recently opened" or "most recently closed"?
For "most recently closed", it'd be something like this:
// This is supposed to be a script (not an indicator or EA) void OnStart() { HistorySelect(0,TimeCurrent()); //Select all trading history; //some variables we'll need... long LastLossTime=0; long LastLossTicket=0; for(int i=0; i<HistoryDealsTotal(); i++) //Cycle through the history { ulong ticket=HistoryDealGetTicket(i); //IF: it's a loss and more recent than previous one found... if(HistoryDealGetDouble(ticket,DEAL_PROFIT)<0&&HistoryDealGetInteger(ticket,DEAL_TIME)>LastLossTime) { LastLossTime=HistoryDealGetInteger(ticket,DEAL_TIME); //update last found loss time LastLossTicket=HistoryDealGetInteger(ticket,DEAL_TICKET); //take note of the ticket number } } //Now that we've cycled through the history, did we find any losses? if(LastLossTime>0) { string LastLossSymbol=HistoryDealGetString(LastLossTicket,DEAL_SYMBOL); PrintFormat("Most recent loss incurred at %s in %s.",TimeToString(LastLossTime),LastLossSymbol); } else { Print("Most recent loss: none found!"); } }
Notes:
- this is a crude example I threw together in 10 minutes or so, not to be taken as an example of good and proper coding :D (for example, I'm getting DEAL_TIME twice)
- if your deal history contains separate commissions or charges (e.g. islamic account), you'll need to introduce additional filters to filter those out, see Deal properties for more info
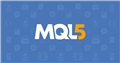
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use