I would like to add the volume of deals. Separated by market buy and market sell.
The problem: Apparently OnTick() is often not called!
Test: I placed a chart with this EA right next to the MT5 T&S. In the T&S you can see deals coming in that are not seen by the EA.
I did this during a time with low turnaround. 2 to 4 ticks a sek. for sure no performance problem.
What is going on here?
void OnTick()
{
static int tickCalls = 0;
static int tickCount = 0;
static int tickDeal = 0;
tickCalls += 1;
if(SymbolInfoTick(Symbol(),tick))
{
tickCount += 1;
if((tick.flags&TICK_FLAG_SELL)==TICK_FLAG_SELL || (tick.flags&TICK_FLAG_BUY)==TICK_FLAG_BUY)
tickDeal += 1;
}
Comment(" Calls: " + IntegerToString(tickCalls)+
" Count: " + IntegerToString(tickCount)+
" Deals: " + IntegerToString(tickDeal));
};
I haven't worked with this yet but try MqlBookInfo because the T&S data looks exactly like the Market Book Data.
Time and sales has nothing to do with the "book data".
You need to use CopyTicks() or CopyTicksRange().
a good hint and a place where i found the confirmation for my measurements. OnTick is not called for each incoming tick.
Docu for CopyTicks:
CopyTicks
'CopyTicks() can wait for the result for 45 seconds in Expert Advisors and scripts: as distinct from indicators, every Expert Advisor and script operate in a separate thread, and therefore can wait 45 seconds till the completion of synchronization. If the required amount of ticks fails to be synchronized during this time, CopyTicks() will return available ticks by timeout and will continue synchronization. OnTick() in Expert Advisor is not a handler of every tick, it only notifies an Expert Advisor about changes in the market. It can be a batch of changes: the terminal can simultaneously make a few ticks, but OnTick() will be called only once to notify the EA of the latest market state.'
Should I use CopyTicks() or SymbolInfoTick() for this?
Time and sales has nothing to do with the "book data".
You need to use CopyTicks() or CopyTicksRange().
I am just going to put this here:
struct MqlBookInfo { ENUM_BOOK_TYPE type; // Order type from ENUM_BOOK_TYPE enumeration double price; // Price long volume; // Volume double volume_real; // Volume with greater accuracy };
And maybe he can combine it with CopyTicks somehow.
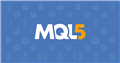
- www.mql5.com
Code to get every Deal (Time & Sale)
The question remains how to determine the number of ticks to be copied. While all FDAX traders were at lunch, I got max 4 deals on one OnTick call. Maybe I just take the number of deals found during the latest OnTick + 10 for the next call.
//+------------------------------------------------------------------+ //| Tick function | //+------------------------------------------------------------------+ void OnTick() { static int numDeals = 0; static long firstTimeMsc = 0; static long lastTimeMsc = 0; Print("------------------------------------ OnTick"); int tickCount = CopyTicks(Symbol(),tick,COPY_TICKS_TRADE,lastTimeMsc,10); // 10 = Number of ticks to be copied if(tickCount > 0) { for(int idx = 0; idx < tickCount; idx++) { if(firstTimeMsc == 0) firstTimeMsc = tick[idx].time_msc; // init if(tick[idx].time_msc > lastTimeMsc) { numDeals++; lastTimeMsc = tick[idx].time_msc; PrintFormat("%d. Deal after %d ms at %.1f Vol: %.0f",numDeals,tick[idx].time_msc-firstTimeMsc,tick[idx].last,tick[idx].volume); } } // Loop } };
Which gives you:
PJ 0 12:26:21.733 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
CM 0 12:26:21.739 DumpTicksOne (DDH22,M1) 1. Deal after 0 ms at 15238.0 Vol: 1
LD 0 12:26:21.739 DumpTicksOne (DDH22,M1) 2. Deal after 2711 ms at 15237.0 Vol: 1
RJ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 3. Deal after 163161 ms at 15231.0 Vol: 1
GQ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 4. Deal after 164853 ms at 15232.0 Vol: 7
PD 0 12:26:21.739 DumpTicksOne (DDH22,M1) 5. Deal after 164856 ms at 15233.0 Vol: 1
RJ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 6. Deal after 164859 ms at 15233.0 Vol: 1
DP 0 12:26:21.744 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HD 0 12:26:22.100 DumpTicksOne (DDH22,M1) 7. Deal after 164866 ms at 15233.0 Vol: 1
CJ 0 12:26:22.101 DumpTicksOne (DDH22,M1) 8. Deal after 164947 ms at 15232.0 Vol: 3
NP 0 12:26:22.101 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
OE 0 12:26:22.768 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
GJ 0 12:26:26.610 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
IO 0 12:26:26.928 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
DE 0 12:26:26.928 DumpTicksOne (DDH22,M1) 9. Deal after 170051 ms at 15232.0 Vol: 1
MJ 0 12:26:29.800 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
KO 0 12:26:30.145 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
PF 0 12:26:30.145 DumpTicksOne (DDH22,M1) 10. Deal after 173264 ms at 15233.0 Vol: 1
HI 0 12:26:33.127 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HN 0 12:26:33.336 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
ND 0 12:26:33.754 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HI 0 12:26:39.123 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
EL 0 12:26:39.123 DumpTicksOne (DDH22,M1) 11. Deal after 182244 ms at 15234.0 Vol: 1
DS 0 12:26:40.437 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
RJ 0 12:26:40.438 DumpTicksOne (DDH22,M1) 12. Deal after 183560 ms at 15235.0 Vol: 1
FM 0 12:26:40.455 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
JS 0 12:26:41.681 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
OH 0 12:26:41.701 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
PM 0 12:26:41.760 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
KR 0 12:26:42.757 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
FG 0 12:26:47.041 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HL 0 12:26:47.054 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
CQ 0 12:26:49.095 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HF 0 12:26:50.614 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
LN 0 12:26:50.614 DumpTicksOne (DDH22,M1) 13. Deal after 193735 ms at 15233.0 Vol: 4
. . .
Code to get every Deal (Time & Sale)
The question remains how to determine the number of ticks to be copied. While all FDAX traders were at lunch, I got max 4 deals on one OnTick call. Maybe I just take the number of deals found during the latest OnTick + 10 for the next call.
Which gives you:
PJ 0 12:26:21.733 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
CM 0 12:26:21.739 DumpTicksOne (DDH22,M1) 1. Deal after 0 ms at 15238.0 Vol: 1
LD 0 12:26:21.739 DumpTicksOne (DDH22,M1) 2. Deal after 2711 ms at 15237.0 Vol: 1
RJ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 3. Deal after 163161 ms at 15231.0 Vol: 1
GQ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 4. Deal after 164853 ms at 15232.0 Vol: 7
PD 0 12:26:21.739 DumpTicksOne (DDH22,M1) 5. Deal after 164856 ms at 15233.0 Vol: 1
RJ 0 12:26:21.739 DumpTicksOne (DDH22,M1) 6. Deal after 164859 ms at 15233.0 Vol: 1
DP 0 12:26:21.744 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HD 0 12:26:22.100 DumpTicksOne (DDH22,M1) 7. Deal after 164866 ms at 15233.0 Vol: 1
CJ 0 12:26:22.101 DumpTicksOne (DDH22,M1) 8. Deal after 164947 ms at 15232.0 Vol: 3
NP 0 12:26:22.101 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
OE 0 12:26:22.768 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
GJ 0 12:26:26.610 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
IO 0 12:26:26.928 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
DE 0 12:26:26.928 DumpTicksOne (DDH22,M1) 9. Deal after 170051 ms at 15232.0 Vol: 1
MJ 0 12:26:29.800 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
KO 0 12:26:30.145 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
PF 0 12:26:30.145 DumpTicksOne (DDH22,M1) 10. Deal after 173264 ms at 15233.0 Vol: 1
HI 0 12:26:33.127 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HN 0 12:26:33.336 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
ND 0 12:26:33.754 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HI 0 12:26:39.123 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
EL 0 12:26:39.123 DumpTicksOne (DDH22,M1) 11. Deal after 182244 ms at 15234.0 Vol: 1
DS 0 12:26:40.437 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
RJ 0 12:26:40.438 DumpTicksOne (DDH22,M1) 12. Deal after 183560 ms at 15235.0 Vol: 1
FM 0 12:26:40.455 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
JS 0 12:26:41.681 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
OH 0 12:26:41.701 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
PM 0 12:26:41.760 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
KR 0 12:26:42.757 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
FG 0 12:26:47.041 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HL 0 12:26:47.054 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
CQ 0 12:26:49.095 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
HF 0 12:26:50.614 DumpTicksOne (DDH22,M1) ------------------------------------ OnTick
LN 0 12:26:50.614 DumpTicksOne (DDH22,M1) 13. Deal after 193735 ms at 15233.0 Vol: 4
. . .

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I would like to add the volume of deals. Separated by market buy and market sell.
The problem: Apparently OnTick() is often not called!
Test: I placed a chart with this EA right next to the MT5 T&S. In the T&S you can see deals coming in that are not seen by the EA.
I did this during a time with low turnaround. 2 to 4 ticks a sek. for sure no performance problem.
What is going on here?