Looks like you are doing almost everything correctly.
OnInit should not return 0 on error, but INIT_FAILED.
ZigZag does not have a value as long as there is no connection point. So most of the time the buffer will be zero.
Take a look at the ZigZag Code of your iCustom indicator.
Your code, although small, will be very resource hungry.
BTW, you can also check the return value of CopyBuffer, for completeness.
Dominik Egert # :
Looks like you are doing almost everything correctly.
OnInit should not return 0 on error, but INIT_FAILED.
ZigZag does not have a value as long as there is no connection point. So most of the time the buffer will be zero.
Take a look at the ZigZag Code of your iCustom indicator .
Your code, although small, will be very resource hungry.
BTW, you can also check the return value of CopyBuffer, for completeness.
i am almost near, its not synchronizing buffer values with symbol as series
Not sure what i am doing wrong, any help appreciated
Thanks
//+------------------------------------------------------------------+ //| Read_Buffer.mq5 | //| Copyright 2021, Dark Ryd3r | //| https://t.me/DarkRyd3r | //+------------------------------------------------------------------+ #property copyright "Copyright 2021, Dark Ryd3r" #property link "https://t.me/DarkRyd3r" #property version "1.00" #property indicator_chart_window #property indicator_plots 0 #define RESET 0 int min_rates_total; int symbolCount; int start_pos=0,count=1; string symbolsList[]; struct SATR { int handle; double buffer[]; } Read_Buffer[]; double mbuffer; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { EventSetTimer(5); min_rates_total=100; //--- indicator buffers mapping symbolCount=SymbolsTotal(true); if( ArrayResize(symbolsList, symbolCount) < symbolCount ) return (INIT_FAILED); //Resize Handles if( ArrayResize(Read_Buffer, symbolCount) < symbolCount ) return (INIT_FAILED); for(int i=0; i<symbolCount; i++) { symbolsList[i] = SymbolName(i,true); Read_Buffer[i].handle =iCustom(symbolsList[i],PERIOD_M1,"Examples\\ZigZagColor",55,5,3 ); if(Read_Buffer[i].handle==INVALID_HANDLE) { Print(" Failed to load iCustom Indicator ZigZagColor"); return(INIT_FAILED); } ArraySetAsSeries(Read_Buffer[i].buffer,true); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //---- EventKillTimer(); //---- } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnTimer(void) { for (int j=0; j<symbolCount; j++) { symbolsList[j] = SymbolName(j,true); Print("55",symbolsList[j],mbuffer); } } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int maxbars = rates_total-rates_total+2000; static datetime TIME[]; static datetime curr=NULL; for (int j=0; j<symbolCount; j++) { symbolsList[j] = SymbolName(j,true); //Print(symbolsList[j]); if(BarsCalculated(Read_Buffer[j].handle)<min_rates_total) return(RESET); if(BarsCalculated(Read_Buffer[j].handle)<Bars(symbolsList[j],PERIOD_M1)) return(prev_calculated); if(CopyBuffer(Read_Buffer[j].handle,1,0,maxbars,Read_Buffer[j].buffer)<=0) return(RESET); if(CopyTime(symbolsList[j],PERIOD_M1,0,maxbars,TIME)<=0) return(RESET); //ArraySetAsSeries(Read_Buffer,true); if(curr!=TIME[0]) { curr=TIME[0]; double swing_value[4]= {0.0,0.0,0.0,0.0}; datetime swing_date[4]= {0,0,0,0}; int found=NULL; double tmp=NULL; int bar=NULL; while(found<4 && bar<maxbars && !IsStopped()) { if(Read_Buffer[j].buffer[bar]) { swing_value[found]=Read_Buffer[j].buffer[bar]; swing_date[found]=TIME[bar]; found++; } bar++; } mbuffer= swing_value[0]; } } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+
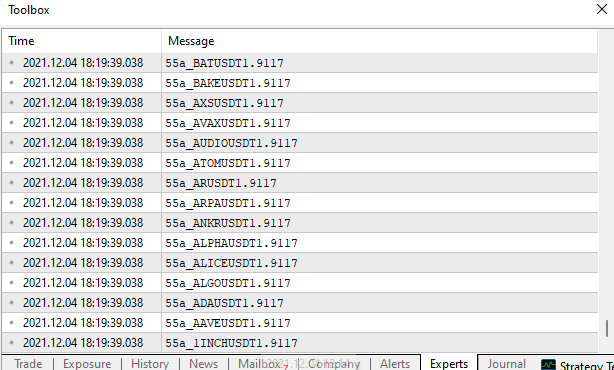

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hi,
I am trying to load ZigZagColor indicator to scan multi symbols and want to read its ZigzagPeakBuffer buffer number '0' but in result its only returning only some of the ZigzagPeakBuffer as a correct result, and other values are returning 0.0
Please help me letting know what i am doing wrong
Thanks