Dear Members
I am trying to use <MovingAverage.mqh> SimpleMA method in a Class.
I have defined arrayClose[] and used CopyClose to get closing prices into array.
However, when I use this array in SimpleMA(index,MAPeriod,arrayClose); it is returning 0.0 for SMA values.
Is it necessary to use SimpleMA in Indicator only?
if not then can a custom Close[] will work ?
below is the code
The SimpleMA function doesn't check the AS_SERIES Flag. So instead use the SimpleMAonBuffer function.
Thanks Navdeep
I think I read somewhere in the forum SimpleMAOnBuffer does not work with MQL5 !!!
Thanks Navdeep
I think I read somewhere in the forum SimpleMAOnBuffer does not work with MQL5 !!!
#property indicator_chart_window #property indicator_buffers 1 #property indicator_plots 1 #property indicator_type1 DRAW_LINE #property indicator_color1 clrRed #include <MovingAverages.mqh> input int inpPeriod = 14; double bufferMa[]; int OnInit(void) { SetIndexBuffer(0,bufferMa,INDICATOR_DATA); ArraySetAsSeries(bufferMa,true); IndicatorSetString(INDICATOR_SHORTNAME,"SimpleMAOnBuffer"); return(INIT_SUCCEEDED); } int OnCalculate(const int rates_total, const int prev_calculated, const datetime& time[], const double& open[], const double& high[], const double& low[], const double& close[], const long& tick_volume[], const long& volume[], const int& spread[]) { if(!ArrayGetAsSeries(close)) ArraySetAsSeries(close,true); SimpleMAOnBuffer(rates_total,prev_calculated,0,inpPeriod,close,bufferMa); return(rates_total); }
Working corectly
Yeh I have been trying to check on forum too and found few examples of it.
I have created the one as below for Normalized Volume. It stills works slow though now I have got latest bar displayed.
Is it volume (tick or real) or input period (55) causing it to be SLOW ? Also noticed that if I put 3 versions of different MA Mode, graphical display is still same of the first mode used !!! see the screen shot below
Thanks for your inputs. I am going to try it on another indicator for MASlope and see how it works.
//+----------------------------------------------------------------------------------------------------------+ //| Ci_NormVolume.mq5 //+----------------------------------------------------------------------------------------------------------+ #property description "Normalized Volume" #include <MovingAverages.mqh> #property indicator_separate_window #property indicator_buffers 4 #property indicator_plots 1 #property indicator_color1 clrGreen,clrRed //+----------------------------------------------------------------------------------------------------------+ //| Define Input Parameters //+----------------------------------------------------------------------------------------------------------+ input int Inp_MAPeriod = 55; // Averaging Period input ENUM_MA_METHOD Inp_MAMethod = MODE_LWMA; // Averaging Method input ENUM_APPLIED_VOLUME Inp_AppliedVolume = VOLUME_TICK; // Applied Volume Type input int Inp_Threshold = 150; // int Inp_Digits = 0; //+----------------------------------------------------------------------------------------------------------+ //| Global Variables //+----------------------------------------------------------------------------------------------------------+ int vMAPeriod; //+----------------------------------------------------------------------------------------------------------+ //| Define Indicator Buffers for Data & Arrays for Indicator Calculation //+----------------------------------------------------------------------------------------------------------+ double Buffer_NormVolume[]; double Buffer_Colors[]; double Buffer_Volume[]; double Buffer_VolumeMA[]; //+----------------------------------------------------------------------------------------------------------+ //| Custom indicator initialization function //+----------------------------------------------------------------------------------------------------------+ void OnInit() { //+--------------------------------------------------------------------------------------------------------+ //| Check Input Parameter Values and set them to Minimum Recommended, if required //+--------------------------------------------------------------------------------------------------------+ if(Inp_MAPeriod < 2) { vMAPeriod = 55; PrintFormat("Parameter Inp_MAPeriod=%d. Min Recommended value=%d used for calculations.",Inp_MAPeriod,vMAPeriod); } else vMAPeriod = Inp_MAPeriod; //+--------------------------------------------------------------------------------------------------------+ //| Indicator SubWindow common 'properties' (used instead of #property) //+--------------------------------------------------------------------------------------------------------+ IndicatorSetString(INDICATOR_SHORTNAME,"AKT Normalize Volume ("+(string)vMAPeriod+" / "+EnumToString(Inp_MAMethod)+") "); IndicatorSetInteger(INDICATOR_DIGITS,Inp_Digits); // Set n digits for Normalize Volume IndicatorSetDouble(INDICATOR_MINIMUM,0.00); IndicatorSetInteger(INDICATOR_HEIGHT,125); //--- LEVELS IndicatorSetInteger(INDICATOR_LEVELS,0,clrSilver); IndicatorSetDouble(INDICATOR_LEVELVALUE,0,Inp_Threshold); //+--------------------------------------------------------------------------------------------------------+ //| Indicator Buffers mapping / binding //+--------------------------------------------------------------------------------------------------------+ SetIndexBuffer(0,Buffer_NormVolume,INDICATOR_DATA); SetIndexBuffer(1,Buffer_Colors,INDICATOR_COLOR_INDEX); SetIndexBuffer(2,Buffer_Volume,INDICATOR_CALCULATIONS); SetIndexBuffer(3,Buffer_VolumeMA,INDICATOR_CALCULATIONS); //+--------------------------------------------------------------------------------------------------------+ //| Plot Indicator Lables in SubWindow (used instead of #property) //+--------------------------------------------------------------------------------------------------------+ PlotIndexSetString(0,PLOT_LABEL,"Norm Vol ("+string(vMAPeriod)+")"); PlotIndexSetInteger(0,PLOT_DRAW_TYPE,DRAW_COLOR_HISTOGRAM); PlotIndexSetInteger(0,PLOT_LINE_STYLE,STYLE_SOLID); PlotIndexSetInteger(0,PLOT_LINE_WIDTH,2); PlotIndexSetDouble(0,PLOT_EMPTY_VALUE,EMPTY_VALUE); //--- indexes draw begin settings //PlotIndexSetInteger(0,PLOT_DRAW_BEGIN,vMAPeriod); } //+----------------------------------------------------------------------------------------------------------+ //| MAIN Iteration function for Custom Normalize Volume Indicator //+----------------------------------------------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //ulong start = GetMicrosecondCount(); // CALCULATION START TIME //+--------------------------------------------------------------------------------------------------------+ //| Loop to FILL Applied Volume values into Buffer_Volume //+--------------------------------------------------------------------------------------------------------+ if(prev_calculated == 0) { Buffer_Volume[0] = 0.00; // Set zero value to Index[0] } int vPos; if(prev_calculated == 0) vPos = 1; // Start filling from Index[1] else vPos = prev_calculated - 1; // Start filling from Last Index in array for(int i = vPos; i < rates_total && !IsStopped(); i++) { if(Inp_AppliedVolume == VOLUME_TICK) Buffer_Volume[i] = (double)tick_volume[i]; else Buffer_Volume[i] = (double)volume[i]; } //+--------------------------------------------------------------------------------------------------------+ //| Loop to calculate Normalized (Smoothed) Values of the Volume //+--------------------------------------------------------------------------------------------------------+ int vBegin = 0; // Index starting from which data for smoothing available switch(Inp_MAMethod) { case MODE_EMA : if(ExponentialMAOnBuffer(rates_total,prev_calculated,vBegin,vMAPeriod, Buffer_Volume,Buffer_VolumeMA // Source & Target Buffer to Calculate Averaged Values )==0) return 0; case MODE_LWMA : if(LinearWeightedMAOnBuffer(rates_total,prev_calculated,vBegin,vMAPeriod, Buffer_Volume,Buffer_VolumeMA // Source & Target Buffer to Calculate Averaged Values )==0) return 0; //--- Default mode SMA default : if(SimpleMAOnBuffer(rates_total,prev_calculated,vBegin,vMAPeriod, Buffer_Volume,Buffer_VolumeMA // Source & Target Buffer to Calculate Averaged Values )==0) return 0; } //+--------------------------------------------------------------------------------------------------------+ //| Color Index Indicator bars based on Threshold Limit //+--------------------------------------------------------------------------------------------------------+ for(int i = vPos; i < rates_total && !IsStopped(); i++) { Buffer_NormVolume[i] = NormalizeDouble(Buffer_VolumeMA[i],Inp_Digits); Buffer_Colors[i] = (Buffer_NormVolume[i] < Inp_Threshold ? 1:0); } //--- //ulong finish = GetMicrosecondCount(); // CALCULATION END TIME //PrintFormat("%s in %s took %.1f ms",__FUNCTION__,__FILE__,(finish-start)/1000); // Get Calculation time used by the indicator //--- OnCalculate done, return new prev_calculated. return(rates_total); } // END Of Ci_NormVolume indicator calculation //+----------------------------------------------------------------------------------------------------------+
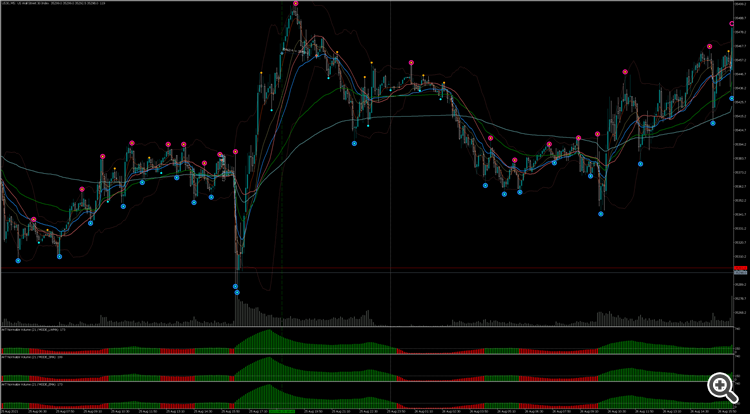
Yeh I have been trying to check on forum too and found few examples of it.
I have created the one as below for Normalized Volume. It stills works slow though now I have got latest bar displayed. Is it volume (tick or real) or input period (55) causing it to be SLOW ?
Thanks for your inputs. I am going to try it on another indicator for MASlope and see how it works.
Run your code on profiler and see what's consuming more resources
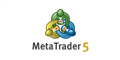
- www.metatrader5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Dear Members
I am trying to use <MovingAverage.mqh> SimpleMA method in a Class.
I have defined arrayClose[] and used CopyClose to get closing prices into array.
However, when I use this array in SimpleMA(index,MAPeriod,arrayClose); it is returning 0.0 for SMA values.
Is it necessary to use SimpleMA in Indicator only?
if not then can a custom Close[] will work ?
below is the code