Michael Ronald Beasley: Im having an issue which Im sure the answer will be straight forward. Im basically building an EA in MT4 which is built on basic indicators, for my Stop loss i am refering back to these indicator values, position type. However I want to know how I can refer back to an indicators value, candle price or calculated number that was calculated in a previous function. Basically I want to write a function to return a number then be able to refer to that number in many other functions in the EA without having to do the calculation again. I my example below you can see my Buy and Sell conditions. in my ManageOpen function. How can I check in a seperate function if that condition has been met, like my SLP function in my example without having to get the EA to run through my buy/sell conditions again.
Firstly, in MQL4, you don't have to use iOpen, iClose, etc. for getting OHLC values for the current Sumbol and Time-frame. You can use the built-in time series arrays, like Open[], Close[]. That way, you don't have to save the values in variables as they are already predefined global variables.
For saving indicator values in global variables, here is an example:
input int FastMAPeriod = 13; input ENUM_MA_METHOD FastMAType = MODE_SMA; input ENUM_APPLIED_PRICE FastMAPrice = PRICE_CLOSE; // Define Global Variables for storing Fast MA Values #define COUNT_FAST_MA 4 double FastMAValue[ COUNT_FAST_MA ]; void GetFastMAValues() { for( int i = 0; i < COUNT_FAST_MA; i++ ) FastMAValue[ i ] = iMA( _Symbol, _Period, FastMAPeriod, 0, FastMAType, FastMAPrice, i + 1 ); } void OnTick() { // Check for New Bar static datetime BarTimeCurrent = WRONG_VALUE; datetime BarTimePrevious = BarTimeCurrent; BarTimeCurrent = Time[0]; bool NewBarFlag = ( BarTimeCurrent != BarTimePrevious ); // Process MA Indicator Values if( NewBarFlag ) { GetFastMAValues(); // Get the Fast MA Values if( ( FastMAValue[0] > FastMAValue[1] ) && ( Close[1] > Close[2] ) ) { /* Trend is up! Do something ... */ } else { if( ( FastMAValue[0] < FastMAValue[1] ) && ( Close[1] < Close[2] ) ) { /* Trend is down! Do something ... */ } else { /* Lets do something else ... */ } } } }
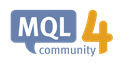
Open - Predefined Variables - MQL4 Reference
- docs.mql4.com
Open - Predefined Variables - MQL4 Reference

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hey guys,
Im having an issue which Im sure the answer will be straight forward. Im basically building an EA in MT4 which is built on basic indicators, for my Stop loss i am refering back to these indicator values, position type. However I want to know how I can refer back to an indicators value, candle price or calculated number that was calculated in a previous function. Basically I want to write a function to return a number then be able to refer to that number in many other functions in the EA without having to do the calculation again.
I my example below you can see my Buy and Sell conditions. in my ManageOpen function. How can I check in a seperate function if that condition has been met, like my SLP function in my example without having to get the EA to run through my buy/sell conditions again.