Hello Everyone, Im facing trouble in deleting an active pending order from mt5 through python. I keep getting an error out of this code. Can someone please help ???
import MetaTrader5 as mt5
from MetaTrader5 import *
if not mt5.initialize():
print("initialize() failed, error code =", mt5.last_error())
quit()
symbol = 'BTCUSD'
lot = 0.02
price = mt5.symbol_info_tick(symbol).bid
deviation = 20
close_request={ "action": mt5.TRADE_ACTION_REMOVE,
"symbol": 'BTCUSD',
"volume": lot,
"type": mt5.ORDER_TYPE_BUY_STOP,
"position": 114296760,
"price": price,
"deviation": deviation,
"magic": 0,
"comment": "python script op",
"type_time": mt5.ORDER_TIME_GTC, # good till cancelled
"type_filling": 1,
}
# send a close request
result=mt5.order_send(close_request)
print(" result",result)
you might try below at your python codes
1. change
"position": 114296760,
to
"order": (with pending order's order #),
2. remove "price" and "deviation" and "type" ("type_time" might NOT be required too) - those parameters are NOT required for pending order removal at MT5-python.
3. ensure "type_filling" is the right one. try either mt5.ORDER_FILLING_IOC or mt5.ORDER_FILLING_FOK
See this ariticle...
https://kritthanit-m.medium.com/manage-mt5-orders-using-python-7f4d8dee742f
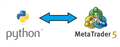
- Kritthanit Malathong
- kritthanit-m.medium.com
See this ariticle...
https://kritthanit-m.medium.com/manage-mt5-orders-using-python-7f4d8dee742f
Hello,
I tried the code from the link and it didn t close any orders. Can you help?
Deleting pending orders:
def delete_pending(mt5, ticket): close_request = { "action": mt5.TRADE_ACTION_REMOVE, "order": ticket, "type_time": mt5.ORDER_TIME_GTC, "type_filling": mt5.ORDER_FILLING_IOC, } result = mt5.order_send(close_request) if result.retcode != mt5.TRADE_RETCODE_DONE: result_dict = result._asdict() print(result_dict) else: print('Delete complete...')

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello Everyone, Im facing trouble in deleting an active pending order from mt5 through python. I keep getting an error out of this code. Can someone please help ???
import MetaTrader5 as mt5
from MetaTrader5 import *
if not mt5.initialize():
print("initialize() failed, error code =", mt5.last_error())
quit()
symbol = 'BTCUSD'
lot = 0.02
price = mt5.symbol_info_tick(symbol).bid
deviation = 20
close_request={ "action": mt5.TRADE_ACTION_REMOVE,
"symbol": 'BTCUSD',
"volume": lot,
"type": mt5.ORDER_TYPE_BUY_STOP,
"position": 114296760,
"price": price,
"deviation": deviation,
"magic": 0,
"comment": "python script op",
"type_time": mt5.ORDER_TIME_GTC, # good till cancelled
"type_filling": 1,
}
# send a close request
result=mt5.order_send(close_request)
print(" result",result)