why this signal won't draw in the indicator window.
A division by zero error will occur.
relVolume[i] = (double)Volume[i] / volumeAvg;
Good call, I've fixed that in case of a zero. Unfortunately that doesn't appear to be the issue.
Good call, I've fixed that in case of a zero. Unfortunately that doesn't appear to be the issue.
Why?
I have find division by zero errors there, and have confirmed that there is no problem after the fix.
What symbols/timeframe are you testing for?
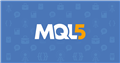
- www.mql5.com
Why?
I have find division by zero errors there, and have confirmed that there is no problem after the fix.
What symbols/timeframe are you testing for?
I added the following to make sure there would never be a divide by zero:
volumeAvg = tempVolSum / RelativePeriod; if(volumeAvg == 0) volumeAvg = 0.001; relVolume[i] = (double)Volume[i] / volumeAvg;
Not a pretty fix, but something to test with. Even with this I'm still not seeing the second buffer draw. Testing on the M15 chart primarily on GBPUSD and a few others. Are you saying it's been working for you?
I added the following to make sure there would never be a divide by zero:
Not a pretty fix, but something to test with. Even with this I'm still not seeing the second buffer draw. Testing on the M15 chart primarily on GBPUSD and a few others. Are you saying it's been working for you?
The "volumeAvg" is long, so even if you put 0.001, it will end up being 0. You should make this part as follows.
volumeAvg = tempVolSum / RelativePeriod; //relVolume[i] = (double)Volume[i] / volumeAvg; if (volumeAvg > 0) relVolume[i] = (double)Volume[i] / volumeAvg; else relVolume[i] = 0.0;
But the root cause of this problem is as follows.
At startup, "prev_calculated" is 0, so "limit = rates_total".
Therefore, the baseBarTime and compBarTime when "i == limit - 1" are as follows.
baseBarTime = iTime(NULL, 0, rates_total - 1); compBarTime = iTime(NULL, 0, (k + rates_total - 1));
At this point, there are no more datetimes to compare, so "tempVolSum" becomes 0.
As a result, "volumeAvg" is also set to 0.
With this in mind, you should modify it as follows.
limit = rates_total - prev_calculated; //if(prev_calculated > 0) //limit++; if(prev_calculated == 0) limit = rates_total - RelativePeriod; for(i = 0; i <= limit; i++) {
The "volumeAvg" is long, so even if you put 0.001, it will end up being 0. You should make this part as follows.
But the root cause of this problem is as follows.
At startup, "prev_calculated" is 0, so "limit = rates_total".
Therefore, the baseBarTime and compBarTime when "i == limit - 1" are as follows.
At this point, there are no more datetimes to compare, so "tempVolSum" becomes 0.
As a result, "volumeAvg" is also set to 0.
With this in mind, you should modify it as follows.
Nagisa, thank you very much for taking the time to help me out! That was indeed the issue and I greatly appreciate your input!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I'm coding a volume-based custom indicator with two signals and for some reason that I just can't figure out the second line won't draw. The name of the buffer that won't draw is relVolumeMA in the code below. I'm not a programming expert by any stretch, but have done quite a few indicators and I'm just not seeing why this signal won't draw in the indicator window. A second set of eyes would be awesome.