It's not possible for your struct because it contains a dynamic array. But you could rearrange your container. Make it a dynamic array of a struct that is writeable.
struct test { int zero; int one; double two; }; test guy[]; void OnStart() { int size = 10; int handle = FileOpen("test.bin", FILE_BIN | FILE_WRITE); ArrayResize(guy, size); Fill(guy); for(int i = 0; i < size; i++) { FileWriteStruct(handle, guy[i]); } FileClose(handle); }
Is it possible? If so, what am I doing wrong?
the compiler displays the error ".. structures or classes containing objects are not allowed."
thanks.
struct test { int zero; int one[]; double two[]; }; test guy; //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { int handle = FileOpen("test.bin", FILE_BIN | FILE_WRITE); FileWriteInteger(handle, guy.zero); int size = ArraySize(guy.one); FileWriteInteger(handle, size); for( int i = 0; i < size; i++ ) FileWriteInteger(handle, guy.one[i]); size = ArraySize(guy.two); FileWriteInteger(handle, size); for( int i = 0; i < size; i++ ) FileWriteDouble(handle, guy.two[i]); FileClose(handle); }
It's not possible for your struct because it contains a dynamic array. But you could rearrange your container. Make it a dynamic array of a struct that is writeable.
thanks for your reply.
the reason why i wanted the dynamic arrays inside the structure is to be able to access how many elements they contain using ArraySize() (as i was attempting in the code example i posted) without having to use other functions.
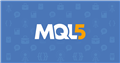
- www.mql5.com
struct test { int zero; int one[]; double two[]; void write(int h){ FileWriteInteger(h,zero); int size = ArraySize(one); FileWriteInteger(h, size); for( int i = 0; i < size; i++ ) FileWriteInteger(h, one[i]); size = ArraySize(two); FileWriteInteger(h, size); for( int i = 0; i < size; i++ ) FileWriteDouble(handle, two[i]); } };Encapsulate your I/O inside the structure.
void OnStart() { int handle = FileOpen("test.bin", FILE_BIN | FILE_WRITE); guy.write(handle); FileClose(handle);
Encapsulate your I/O inside the structure.
this worked. thank you.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Is it possible? If so, what am I doing wrong?
the compiler displays the error ".. structures or classes containing objects are not allowed."
thanks.