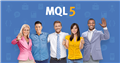
- www.mql5.com
int init(){ ⋮ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]){ ⋮ int deinit(){
Use the new event handlers or the old ones. Don't mix!
You should stop using the old event handlers and IndicatorCounted() and start using new event handlers.
Event
Handling Functions - Functions - Language Basics - MQL4 Reference
How
to do your lookbacks correctly - MQL4 programming forum #9-14.
Use the new event handlers or the old ones. Don't mix!
You should stop using the old event handlers and IndicatorCounted() and start using new event handlers.
Event
Handling Functions - Functions - Language Basics - MQL4 Reference
How
to do your lookbacks correctly - MQL4 programming forum #9-14.
Hi William,
Thank you for your input.
I have edited the event handlers as advised, but it's still not working...
I tried unifying using old handlers as well but no luck..
I would appreciate your further advice.
You should always check the Experts tab when you have a problem.
The Deinit is not the problem.
The problem with your code is an array out of range due to
SetIndexBuffer(0,Buffer_0); SetIndexStyle(0,DRAW_ARROW); SetIndexArrow(0,242); SetIndexBuffer(0,Buffer_1); SetIndexStyle(0,DRAW_ARROW); SetIndexArrow(0,241);
Buffer_0 is not set because you re-assign index 0 to Buffer_1.
You should do
SetIndexBuffer(1,Buffer_1); SetIndexStyle(1,DRAW_ARROW); SetIndexArrow(1,241);
You should always check the Experts tab when you have a problem.
The Deinit is not the problem.
The problem with your code is an array out of range due to
Buffer_0 is not set because you re-assign index 0 to Buffer_1.
You should do
Hi Keith,
OMG That was it! You're a genius! Thank you so much!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use