-
double DiffInPips = MathAbs(NormalizeDouble(stopLoss-openPrice,Digits)/Point);
In code (MT 4): Risk depends on your initial stop loss, lot size, and the value of the pair. It does not depend on margin and leverage.- You place the stop where it needs to be - where the reason for the trade is no longer valid. E.g. trading a support bounce the stop goes below the support.
- AccountBalance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the spread, and DeltaPerLot is usually around $10/pip but it takes account of the exchange rates of the pair vs. your account currency.)
- Do NOT use TickValue by itself - DeltaPerLot
and verify that MODE_TICKVALUE is returning a value in your deposit currency, as promised by the documentation, or
whether it is returning a value in the instrument's base currency.
MODE_TICKVALUE is not reliable on non-fx instruments with many brokers - MQL4 programming forum 2017.10.10
Is there an universal solution for Tick value? - Currency Pairs - General - MQL5 programming forum 2018.02.11
Lot value calculation off by a factor of 100 - MQL5 programming forum 2019.07.19 - You must normalize lots properly and check against min and max.
- You must also check FreeMargin to avoid stop out
Most pairs are worth about $10 per PIP. A $5 risk with a (very small) 5 PIP SL is $5/$10/5 or 0.1 Lots maximum.
Never risk more than a small percentage of your account, certainly less than 2% per trade, 6% total.
- In code (MT 4): Risk depends on your initial stop loss,
lot size, and the value of the pair. It does not depend on margin and leverage.
- You place the stop where it needs to be - where the reason for the trade is no longer valid. E.g. trading a support bounce the stop goes below the support.
- AccountBalance * percent/100 = RISK = OrderLots * (|OrderOpenPrice - OrderStopLoss| * DeltaPerLot + CommissionPerLot) (Note OOP-OSL includes the spread, and DeltaPerLot is usually around $10/pip but it takes account of the exchange rates of the pair vs. your account currency.)
- Do NOT use TickValue by itself - DeltaPerLot and
verify that
MODE_TICKVALUE is returning a value in your deposit currency, as promised by the documentation, or whether it is
returning a value in the instrument's base currency.
MODE_TICKVALUE is not reliable on non-fx instruments with many brokers - MQL4 programming forum 2017.10.10
Is there an universal solution for Tick value? - Currency Pairs - General - MQL5 programming forum 2018.02.11
Lot value calculation off by a factor of 100 - MQL5 programming forum 2019.07.19 - You must normalize lots properly and check against min and max.
- You must also check FreeMargin to avoid stop out
Most pairs are worth about $10 per PIP. A $5 risk with a (very small) 5 PIP SL is $5/$10/5 or 0.1 Lots maximum.
Thanks. I believe that I should be able to arrive at a desired lot size with my percentage risk, stopLossPrice and entryPrice. I ended up doing this:
double DiffInPoints = MathAbs(NormalizeDouble(stopLossPrice-entryPrice,Digits)/Point); double CalculateLotSize(double riskedPoints) { double riskedAmount = percentageRisk/AccountBalance(); return riskedAmount/riskedPoints; }
I want to use
double NormalizeLots(double lots, string pair=""){ if (pair == "") pair = Symbol(); double lotStep = MarketInfo(pair, MODE_LOTSTEP), minLot = MarketInfo(pair, MODE_MINLOT); lots = MathRound(lots/ls) * ls; if (lots < minLot) lots = 0; // or minLot return(lots); }
in https://www.mql5.com/en/forum/146370#comment_3693988 but I don't know what "ls" represents. Pardon me, I don't seem to get this.
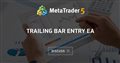
- 2013.08.16
- www.mql5.com
Thanks. I believe that I should be able to arrive at a desired lot size with my percentage risk, stopLossPrice and entryPrice. I ended up doing this:
I want to use
in https://www.mql5.com/en/forum/146370#comment_3693988 but I don't know what "ls" represents. Pardon me, I don't seem to get this.
You have minLot, lotStep, and maxLot.
If minlot equals 0.10 and lotStep is 0.01, then the only valid lot sizes are [0.10, 0.11, 0.12 … maxLot]
If minlot equals 0.10 and lotStep is 0.10, then the only valid lot sizes are [0.10, 0.20, 0.30 … maxLot]
If minlot equals 0.01 and lotStep is 0.02, then the only valid lot sizes are [0.01, 0.03, 0.05 … maxLot]
You have minLot, lotStep, and maxLot.
If minlot equals 0.10 and lotStep is 0.01, then the only valid lot sizes are [0.10, 0.11, 0.12 … maxLot]
If minlot equals 0.10 and lotStep is 0.10, then the only valid lot sizes are [0.10, 0.20, 0.30 … maxLot]
If minlot equals 0.01 and lotStep is 0.02, then the only valid lot sizes are [0.01, 0.03, 0.05 … maxLot]
For example, I made ls = 0.01.
I had a lot size of 5.136550615276671e-08 and when I used NormalizeLots() on the figure I got 0.0 as the result. I want to get the lot size to 2 decimal places, i.e 5.14.
For example, I made ls = 0.01.
I had a lot size of 5.136550615276671e-08 and when I used NormalizeLots() on the figure I got 0.0 as the result. I want to get the lot size to 2 decimal places, i.e 5.14.
- You don't make lotStep anything. The broker states theirs.
- 5.136550615276671e-08 equals 0.00000005136550615276671. Not 5.14

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have written code the place a send order but I want to be able to calculate the lot size based on a few things. Given the entry price and stop loss, how can I calculate my lot size so that 2.5% of my current account balance would be risked on trades?
I know how to calculate the number of pips between my entry price and stop loss:
I believe it is needed to solve this, but not sure how to arrive at the desired lot size.