Anky Five:
Hello traders,
I would like to create a sound alert on an indicator I use when the price closes under or below the indicator lines.
It is a volatility indicator :
Thanks for any help :-)
Just insert this line where you want the sound to be played:
PlaySound("alert2.wav");
Put the wav sound under terminal_directory\Sounds or its subfolders
Further reference:
https://www.mql5.com/en/docs/common/playsound
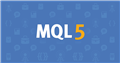
Documentation on MQL5: Common Functions / PlaySound
- www.mql5.com
Common Functions / PlaySound - Reference on algorithmic/automated trading language for MetaTrader 5
Thanks a lot rrocchi !

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Hello traders,
I would like to create a sound alert on an indicator I use when the price closes under or below the indicator lines.
It is a volatility indicator :
Thanks for any help :-)