ECURRENCY TEAM: please fix it
For the fourth+ time, there are no slaves here.
- Learn to code it. If you don't learn MQL4/5, there is no common language for us to communicate. If we tell you what you need, you can't code it. If we give you the code, you don't know how to integrate it into yours.
- or pay (Freelance) someone to code it.
No free help
urgent help.
double smax[25000],smin[25000],bsmax[25000],bsmin[25000];
Make your arrays, extra IndicatorBuffers
ECURRENCY TEAM:
this indicator showing error waring
"the size of local variables is too large (more than 512kb).
please fix it
ok.
https://www.mql5.com/en/charts/10425663/eurusd-h1-qnb-finans-yatirim
#property indicator_chart_window #property indicator_buffers 6 #property indicator_color1 RoyalBlue #property indicator_color2 Red #property indicator_color3 RoyalBlue #property indicator_color4 Red #property indicator_color5 RoyalBlue #property indicator_color6 Red //---- input parameters extern int Length=20; // Bollinger Bands Period extern int Deviation=2; // Deviation was 2 extern double MoneyRisk=1.00; // Offset Factor extern int Signal=1; // Display signals mode: 1-Signals & Stops; 0-only Stops; 2-only Signals; extern int Line=1; // Display line mode: 0-no,1-yes extern int Nbars=1000; //---- indicator buffers double UpTrendBuffer[]; double DownTrendBuffer[]; double UpTrendSignal[]; double DownTrendSignal[]; double UpTrendLine[]; double DownTrendLine[]; double smax[],smin[],bsmax[],bsmin[]; extern bool SoundON=true; bool TurnedUp = false; bool TurnedDown = false; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int init() { string short_name; //---- indicator line IndicatorBuffers(10); SetIndexBuffer(0,UpTrendBuffer); SetIndexBuffer(1,DownTrendBuffer); SetIndexBuffer(2,UpTrendSignal); SetIndexBuffer(3,DownTrendSignal); SetIndexBuffer(4,UpTrendLine); SetIndexBuffer(5,DownTrendLine); SetIndexBuffer(6,smax); SetIndexBuffer(7,smin); SetIndexBuffer(8,bsmax); SetIndexBuffer(9,bsmin); SetIndexStyle(0,DRAW_ARROW,0,1); SetIndexStyle(1,DRAW_ARROW,0,1); SetIndexStyle(2,DRAW_ARROW,0,1); SetIndexStyle(3,DRAW_ARROW,0,1); SetIndexStyle(4,DRAW_LINE); SetIndexStyle(5,DRAW_LINE); SetIndexStyle(6,DRAW_NONE); SetIndexStyle(7,DRAW_NONE); SetIndexStyle(8,DRAW_NONE); SetIndexStyle(9,DRAW_NONE); SetIndexArrow(0,159); SetIndexArrow(1,159); SetIndexArrow(2,108); SetIndexArrow(3,108); IndicatorDigits(MarketInfo(Symbol(),MODE_DIGITS)); //---- name for DataWindow and indicator subwindow label short_name="BBands Stop("+Length+","+Deviation+")"; IndicatorShortName(short_name); SetIndexLabel(0,"UpTrend Stop"); SetIndexLabel(1,"DownTrend Stop"); SetIndexLabel(2,"UpTrend Signal"); SetIndexLabel(3,"DownTrend Signal"); SetIndexLabel(4,"UpTrend Line"); SetIndexLabel(5,"DownTrend Line"); //---- SetIndexDrawBegin(0,Length); SetIndexDrawBegin(1,Length); SetIndexDrawBegin(2,Length); SetIndexDrawBegin(3,Length); SetIndexDrawBegin(4,Length); SetIndexDrawBegin(5,Length); //---- return(0); } //+------------------------------------------------------------------+ //| Bollinger Bands_Stop_v1 | //+------------------------------------------------------------------+ int start() { int shift,trend; for (shift=Nbars;shift>=0;shift--) { UpTrendBuffer[shift]=0; DownTrendBuffer[shift]=0; UpTrendSignal[shift]=0; DownTrendSignal[shift]=0; UpTrendLine[shift]=EMPTY_VALUE; DownTrendLine[shift]=EMPTY_VALUE; } for (shift=Nbars-Length-1;shift>=0;shift--) { smax[shift]=iBands(NULL,0,Length,Deviation,0,PRICE_CLOSE,MODE_UPPER,shift); smin[shift]=iBands(NULL,0,Length,Deviation,0,PRICE_CLOSE,MODE_LOWER,shift); if (Close[shift]>smax[shift+1]) trend=1; if (Close[shift]<smin[shift+1]) trend=-1; if(trend>0 && smin[shift]<smin[shift+1]) smin[shift]=smin[shift+1]; if(trend<0 && smax[shift]>smax[shift+1]) smax[shift]=smax[shift+1]; bsmax[shift]=smax[shift]+0.5*(MoneyRisk-1)*(smax[shift]-smin[shift]); bsmin[shift]=smin[shift]-0.5*(MoneyRisk-1)*(smax[shift]-smin[shift]); if(trend>0 && bsmin[shift]<bsmin[shift+1]) bsmin[shift]=bsmin[shift+1]; if(trend<0 && bsmax[shift]>bsmax[shift+1]) bsmax[shift]=bsmax[shift+1]; if (trend>0) { if (Signal>0 && UpTrendBuffer[shift+1]==-1.0) { UpTrendSignal[shift]=bsmin[shift]; UpTrendBuffer[shift]=bsmin[shift]; if(Line>0) UpTrendLine[shift]=bsmin[shift]; if (SoundON==true && shift==0 && !TurnedUp) { Alert("BBands going Up on ",Symbol(),"-",Period()); TurnedUp = true; TurnedDown = false; } } else { UpTrendBuffer[shift]=bsmin[shift]; if(Line>0) UpTrendLine[shift]=bsmin[shift]; UpTrendSignal[shift]=-1; } if (Signal==2) UpTrendBuffer[shift]=0; DownTrendSignal[shift]=-1; DownTrendBuffer[shift]=-1.0; DownTrendLine[shift]=EMPTY_VALUE; } if (trend<0) { if (Signal>0 && DownTrendBuffer[shift+1]==-1.0) { DownTrendSignal[shift]=bsmax[shift]; DownTrendBuffer[shift]=bsmax[shift]; if(Line>0) DownTrendLine[shift]=bsmax[shift]; if (SoundON==true && shift==0 && !TurnedDown) { Alert("BBands going Down on ",Symbol(),"-",Period()); TurnedDown = true; TurnedUp = false; } } else { DownTrendBuffer[shift]=bsmax[shift]; if(Line>0)DownTrendLine[shift]=bsmax[shift]; DownTrendSignal[shift]=-1; } if (Signal==2) DownTrendBuffer[shift]=0; UpTrendSignal[shift]=-1; UpTrendBuffer[shift]=-1.0; UpTrendLine[shift]=EMPTY_VALUE; } } return(0); }
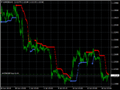
Chart EURUSD, H1, 2019.07.17 12:36 UTC, QNB Finans Yatirim Menkul Degerler A.S., MetaTrader 4, Demo
- www.mql5.com
Symbol: EURUSD. Periodicity: H1. Broker: QNB Finans Yatirim Menkul Degerler A.S.. Trading Platform: MetaTrader 4. Trading Mode: Demo. Date: 2019.07.17 12:36 UTC.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
this indicator showing error waring
"the size of local variables is too large (more than 512kb).
please fix it