From here you start. Then loop over the arrays to build your vector.
https://www.mql5.com/en/docs/standardlibrary/generic/csortedmap/csortedmapcopyto
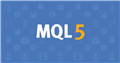
- www.mql5.com
From here you start. Then loop over the arrays to build your vector.
https://www.mql5.com/en/docs/standardlibrary/generic/csortedmap/csortedmapcopyto
Not a valid link:
https://www.mql5.com/en/docs/standardlibrary/generic/csortedmap/csortedmapcopyto
I have read the documentation many times.
The question how do I call the first form of CopyTo
"
CopyTo
Copies all key/value pairs from the sorted hash table to the specified arrays, starting at the specified index.
The version that copies a hash table to the array of key/value pairs.
int CopyTo(
|
"
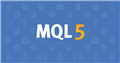
- www.mql5.com
CKeyValuePair<int,int> *values[]; int count=map.CopyTo(values);Did you try this?
Did you try this?
Thank you, very much. That works.
But with your answer I understand now, that I get an array of pointers. This pointers point to objects "on the heap". I have to delete this objects explicit with delete.
//+------------------------------------------------------------------+ //| Script tryCopyTo.mq5 | //+------------------------------------------------------------------+ #property version "1.00" #include <Generic\SortedMap.mqh> //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { //--- CSortedMap<int,int>resultmap; // fill it with some example values resultmap.Add(1, 1); resultmap.Add(2, 4); resultmap.Add(3, 9); printf("resultmap.Count() = %d",resultmap.Count()); CKeyValuePair<int,int>*hValues[]; int count=resultmap.CopyTo(hValues); printf("ArraySize: %d",ArraySize(hValues)); for(int i=0; i<ArraySize(hValues);++i) printf("hValues[i].Key()=%d, hValue[i].Value()=%d",hValues[i].Key(),hValues[i].Value()); // ... program goes on, I can work with hValues[i] ... //--- // ... but it is an array of pointers to dynamically created objects "on the heap". // All that objects must be explicitlly deleted ... // ... that is ugly and I will not use form 1 of CopyTo(). for(int i=0;i<ArraySize(hValues);++i) delete hValues[i]; return; } //+------------------------------------------------------------------+
If the lifetime of the CKeyValuePair does not automatically end at the end of the block of definition, I assume it makes no sence for me to use form 1 of the CopyTo().
So I have to "fall back" to form 2 of the CopyTo(() like the solution in this tread: https://www.mql5.com/en/forum/304390#comment_10728090
Thanks again, MQL5 is very good, but it is not perfect.
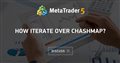
- 2019.02.21
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I use Standard Library Generic Data Collection CSortedMap. To get the values out of the object I like to use CopyTo with an array of CKeyValuePair.