I have met similar problem as you did. Seems like accessing 2d array in `OnCalculate` would lead to `Index out of bound` error.
- use the debugger to check the index and the array size (of any dimension) before you access the array: https://www.mql5.com/en/articles/654
- use ArrayRange() to check the sizes of the dimensions.
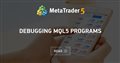
Debugging MQL5 Programs
- www.mql5.com
This article is intended primarily for the programmers who have already learned the language but have not fully mastered the program development yet. It reveals some debugging techniques and presents a combined experience of the author and many other programmers.
You didn't set PDI, NDI, etc.. as buffers with SetIndexBuffer, just declaring a variable as x[] won't work because it is an empty array.

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Dear,
I would like to contrast DIs (ADX) with different averaging time frame , so I created a custom Indicator using the basics of ADX Wilder Moving from PD and ND along with TR to PDS, NDS, PDI and NDI with different averaging number (1,2, 3, to 14). I would like generate a matrix of PDI with in row time and columns 14 PDIs with each different averaging number. I guess that this can be handled with array computation. I tried to do (code below) but i get the message out of range. Please, I appreciate your help and comments: