Hi Shabaz....
Start by creating a Trend Line....
ObjectCreate(chart_ID,name,OBJ_TREND,sub_window,time1,price1,time2,price2)
...then once you get familiar with how it works... create lines for each OHLC value... It's not that difficult, so... try to work it out yourself...
You can also try these functions:
// Functions: //- HLineCreate() //- HLineMove() //- HLineDelete() //+------------------------------------------------------------------+ //| Create the horizontal line | //+------------------------------------------------------------------+ bool HLineCreate(const long chart_ID=0, // chart's ID const string name="HLine", // line name const int sub_window=0, // subwindow index double price=0, // line price const color clr=clrRed, // line color const ENUM_LINE_STYLE style=STYLE_SOLID, // line style const int width=1, // line width const bool back=false, // in the background const bool selection=true, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- if the price is not set, set it at the current Bid price level if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- create a horizontal line if(!ObjectCreate(chart_ID,name,OBJ_HLINE,sub_window,0,price)) { Print(__FUNCTION__, ": failed to create a horizontal line! Error code = ",GetLastError()); return(false); } //--- set line color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set line display style ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style); //--- set line width ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,width); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the line by mouse //--- when creating a graphical object using ObjectCreate function, the object cannot be //--- highlighted and moved by default. Inside this method, selection parameter //--- is true by default making it possible to highlight and move the object ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Move horizontal line | //+------------------------------------------------------------------+ bool HLineMove(const long chart_ID=0, // chart's ID const string name="HLine", // line name double price=0) // line price { //--- if the line price is not set, move it to the current Bid price level if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- move a horizontal line if(!ObjectMove(chart_ID,name,0,0,price)) { Print(__FUNCTION__, ": failed to move the horizontal line! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Delete a horizontal line | //+------------------------------------------------------------------+ bool HLineDelete(const long chart_ID=0, // chart's ID const string name="HLine") // line name { //--- reset the error value ResetLastError(); //--- delete a horizontal line if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete a horizontal line! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+
And for a trendline:
// Functions: //- TrendCreate() //- TrendPointChange() //- TrendDelete() //- ChangeTrendEmptyPoints() //+------------------------------------------------------------------+ //| Create a trend line by the given coordinates | //+------------------------------------------------------------------+ bool TrendCreate(const long chart_ID=0, // chart's ID const string name="TrendLine", // line name const int sub_window=0, // subwindow index datetime time1=0, // first point time double price1=0, // first point price datetime time2=0, // second point time double price2=0, // second point price const color clr=clrRed, // line color const ENUM_LINE_STYLE style=STYLE_SOLID, // line style const int width=1, // line width const bool back=false, // in the background const bool selection=true, // highlight to move const bool ray_left=false, // line's continuation to the left const bool ray_right=false, // line's continuation to the right const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- set anchor points' coordinates if they are not set ChangeTrendEmptyPoints(time1,price1,time2,price2); //--- reset the error value ResetLastError(); //--- create a trend line by the given coordinates if(!ObjectCreate(chart_ID,name,OBJ_TREND,sub_window,time1,price1,time2,price2)) { Print(__FUNCTION__, ": failed to create a trend line! Error code = ",GetLastError()); return(false); } //--- set line color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set line display style ObjectSetInteger(chart_ID,name,OBJPROP_STYLE,style); //--- set line width ObjectSetInteger(chart_ID,name,OBJPROP_WIDTH,width); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the line by mouse //--- when creating a graphical object using ObjectCreate function, the object cannot be //--- highlighted and moved by default. Inside this method, selection parameter //--- is true by default making it possible to highlight and move the object ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- enable (true) or disable (false) the mode of continuation of the line's display to the left ObjectSetInteger(chart_ID,name,OBJPROP_RAY_LEFT,ray_left); //--- enable (true) or disable (false) the mode of continuation of the line's display to the right ObjectSetInteger(chart_ID,name,OBJPROP_RAY_RIGHT,ray_right); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Move trend line anchor point | //+------------------------------------------------------------------+ bool TrendPointChange(const long chart_ID=0, // chart's ID const string name="TrendLine", // line name const int point_index=0, // anchor point index datetime time=0, // anchor point time coordinate double price=0) // anchor point price coordinate { //--- if point position is not set, move it to the current bar having Bid price if(!time) time=TimeCurrent(); if(!price) price=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- reset the error value ResetLastError(); //--- move trend line's anchor point if(!ObjectMove(chart_ID,name,point_index,time,price)) { Print(__FUNCTION__, ": failed to move the anchor point! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| The function deletes the trend line from the chart. | //+------------------------------------------------------------------+ bool TrendDelete(const long chart_ID=0, // chart's ID const string name="TrendLine") // line name { //--- reset the error value ResetLastError(); //--- delete a trend line if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete a trend line! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Check the values of trend line's anchor points and set default | //| values for empty ones | //+------------------------------------------------------------------+ void ChangeTrendEmptyPoints(datetime &time1,double &price1, datetime &time2,double &price2) { //--- if the first point's time is not set, it will be on the current bar if(!time1) time1=TimeCurrent(); //--- if the first point's price is not set, it will have Bid value if(!price1) price1=SymbolInfoDouble(Symbol(),SYMBOL_BID); //--- if the second point's time is not set, it is located 9 bars left from the second one if(!time2) { //--- array for receiving the open time of the last 10 bars datetime temp[10]; CopyTime(Symbol(),Period(),time1,10,temp); //--- set the second point 9 bars left from the first one time2=temp[0]; } //--- if the second point's price is not set, it is equal to the first point's one if(!price2) price2=price1; } //+------------------------------------------------------------------+
This is the best option for MQL4/5:
https://www.mql5.com/en/docs/standardlibrary/chart_object_classes/obj_lines/cchartobjecthline
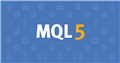
Documentation on MQL5: Standard Library / Graphic Objects / Line Objects / CChartObjectHLine
- www.mql5.com
Standard Library / Graphic Objects / Line Objects / CChartObjectHLine - Reference on algorithmic/automated trading language for MetaTrader 5
Alright. Let me have a good look at it first. Will update this post once i get a hang of it.
Of course there is no 'best way' there will always be issues in between, there is however, preference.
Just go with what works best for you.
ObjectCreate(0,"PrevDayHigh",OBJ_HLINE,0,TimeCurrent(),iHigh(Symbol(),1440,1)); ObjectSet("PrevDayHigh",OBJPROP_COLOR,Blue); ObjectSet("PrevDayHigh",OBJPROP_WIDTH,1); ObjectSet("PrevDayHigh",OBJPROP_STYLE,STYLE_DOT); ObjectSet("PrevDayHigh",OBJPROP_SELECTABLE,false); ObjectSet("PrevDayHigh",OBJPROP_HIDDEN,true); ObjectSet("PrevDayHigh",OBJPROP_BACK,true); ObjectCreate(0,"PrevDayLow",OBJ_HLINE,0,TimeCurrent(),iLow(Symbol(),1440,1)); ObjectSet("PrevDayLow",OBJPROP_COLOR,Red); ObjectSet("PrevDayLow",OBJPROP_WIDTH,1); ObjectSet("PrevDayLow",OBJPROP_STYLE,STYLE_DOT); ObjectSet("PrevDayLow",OBJPROP_SELECTABLE,false); ObjectSet("PrevDayLow",OBJPROP_HIDDEN,true); ObjectSet("PrevDayLow",OBJPROP_BACK,true); ObjectCreate(0,"PrevDayOpen",OBJ_HLINE,0,TimeCurrent(),iOpen(Symbol(),1440,1)); ObjectSet("PrevDayOpen",OBJPROP_COLOR,Blue); ObjectSet("PrevDayOpen",OBJPROP_WIDTH,1); ObjectSet("PrevDayOpen",OBJPROP_STYLE,STYLE_SOLID); ObjectSet("PrevDayOpen",OBJPROP_SELECTABLE,false); ObjectSet("PrevDayOpen",OBJPROP_HIDDEN,true); ObjectSet("PrevDayOpen",OBJPROP_BACK,true); ObjectCreate(0,"PrevDayClose",OBJ_HLINE,0,TimeCurrent(),iClose(Symbol(),1440,1)); ObjectSet("PPrevDayClose",OBJPROP_COLOR,Red); ObjectSet("PrevDayClose",OBJPROP_WIDTH,1); ObjectSet("PrevDayClose",OBJPROP_STYLE,STYLE_SOLID); ObjectSet("PrevDayClose",OBJPROP_SELECTABLE,false); ObjectSet("PrevDayClose",OBJPROP_HIDDEN,true); ObjectSet("PrevDayClose",OBJPROP_BACK,true);

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
hello there,
I am new to MQL4 and i am trying to draw a horizontal line (ray) on OHLC of a candlestick. So far, I am unable to do so. I tried ObjectCreate but no luck. Please help me out.