Your code does not make much sense with respect to the Boolean expression you are using, because:
bool BAH2 = (1||2||3); // will always be 'true', because any non-zero value is equivalent to 'true', so (1||2||3) is (true||true||true) = true bool BAH1 = (4||5); // will always be 'true', because any non-zero value is equivalent to 'true', so (4||5) is (true||true) = true
Also, both Boolean variables are constant and don't depend in any way on the BAH variable. Maybe what you are trying to achieve is the following:
bool BAH2 = (BAH >= 1) && (BAH <= 3); // or (BAH == 1) || (BAH == 2) || (BAH == 3) bool BAH1 = (BAH >= 4) && (BAH <= 5); // or (BAH == 4) || (BAH == 5)
Your code does not make much sense with respect to the Boolean expression you are using, because:
Also, both Boolean variables are constant and don't depend in any way on the BAH variable. Maybe what you are trying to achieve is the following:
Ok thanks, if i want to make 1 to 3 equals to a constant called Takeprofit= 5000
and 4 to 5 equal to a take profit 1000 how do i do that ? My approach above seems to return 1 variable only.
Maybe I am misunderstanding your new question, but did I not just reply to that?
Maybe I should show the complete code for easier understanding:
srand( GetTickCount() ); int BAH = rand() % 5 + 1, TakeProfit2 = 0; bool BAH2 = ( BAH >= 1 ) && ( BAH <= 3 ), BAH1 = ( BAH >= 4 ) && ( BAH <= 5 ); if( BAH2 ) { TakeProfit2 = 500; } if( BAH1 ) { TakeProfit2 = 1000; } Print( "take profit", TakeProfit2 );
And here is a another way of achieving the same result:
srand( GetTickCount() ); int BAH = rand() % 5 + 1, TakeProfit2 = 0; switch( BAH ) { case 1: case 2: case 3: TakeProfit2 = 500; break; case 4: case 5: TakeProfit2 = 1000; break; } Print( "take profit", TakeProfit2 );
In higher level languages this would be a simple task of assigning your values to a list and then passing the list to a random choice function. In MQL we can do the same thing, but it requires us to be slightly more verbose.
void OnStart() { srand(_RandomSeed); int numbers[] = {500, 500, 500, 1000, 1000}; printf("%d is the random choice.", random_choice(numbers)); } template <typename T> T random_choice(T &array[]) { return array[rand() % ArraySize(array)]; }
To help seed the randomization so that its sequence will be different every time you run the code. Without seeding it will always be the same sequence.
You can also use _RandomSeed, as used by @nicholi shen in his post #5. Please folow the link and read the documentation for it.
Also, please remember to read the documentation for which @Anthony Garot gave you the link in his post #2:
MathSrand (or srand)
Sets the starting point for generating a series of pseudorandom integers.
void MathSrand( int seed // initializing number );Parameters
seed
[in] Starting number for the sequence of random numbers.
Return Value
No return value.
Note
The MathRand() function is used for generating a sequence of pseudorandom numbers. Call of MathSrand() with a certain initializing number allows to always produces the same sequence of pseudorandom numbers.
To ensure receipt of non-recurring sequence, use the call of MathSrand(GetTickCount()), since the value of GetTickCount() increases from the moment of the start of the operating system and is not repeated within 49 days, until the built-in counter of milliseconds overflows. Use of MathSrand(TimeCurrent()) is not suitable, because the TimeCurrent() function returns the time of the last tick, which can be unchanged for a long time, for example at the weekend.
Initialization of the random number generator using MathSrand() for indicators and Expert Advisors is better performed in the OnInit() handler; it saves you from the following multiple restarts of the generator in OnTick() and OnCalculate().
Instead of the MathSrand() function you can use the srand() function.
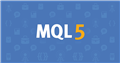
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
p