
You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Here I am again because I could not solve my problem and I do not understand where I am wrong...I show an image:
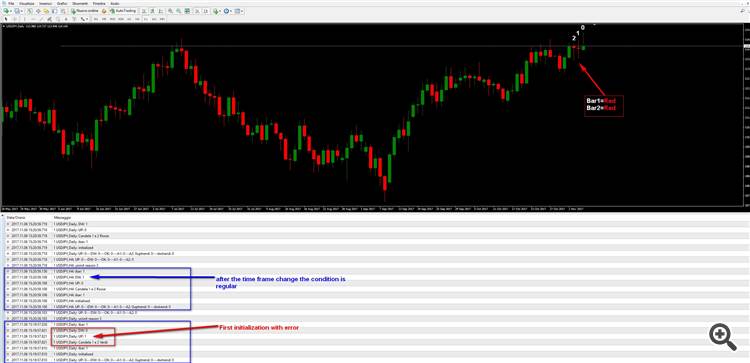
Any suggestions to resolve? Thank you all!!!Download history in MQL4 EA - MQL4 and MetaTrader 4 - MQL4 programming forum
if I use different time frames, I should download M15, M30, H1, H4, D1 data with the function ?!?
I have created a Multi Time Frame Indicator, and I would like to be in M30 by default but can read, for example, Candles in D1, H4, H1 and M30 with iTime () iCLose () iOpen () etc ... I should download data every time launching MT4
@whroeder1 has already answered you in regards to that:
Be it multi-time-frame or multi-symbol, the concepts are similar, so read the following thread (from beginning to end) for some insight:
hello to all, I tried to do some tests to take the color of Heinke Hashi in D1 staying in M30 but it does not always work.
thanks