- Right click -> R is just as easy
- Why do you need to refresh the chart?
- Right click -> R is just as easy
- Why do you need to refresh the chart?
Objects are saved when you close the terminal. Buffers are not. You reopen the chart, it has to recalculate/redraw everything. Otherwise you have a blank chart.
Although I don't understand why you need to force a refresh on your chart since the data is already available.
However... If you want to store your data "long term", you have a few options such as GlobalVariables and/or saving the data to a text file for later use.
https://www.mql5.com/en/docs/globals
If you do that, you should also consider reading over this: https://www.mql5.com/en/docs/basis/variables/variable_scope
And also this for file functions: https://www.mql5.com/en/docs/files
- Jack
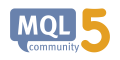
- www.mql5.com
Thanks to everyone for help I will study your advice to find a solution!
How can you remember something on start up?
Your "need" is irrelevant. The chart will be updated as soon as it connects to the server.
How can you not update the indicators creating the current
condition? You aren't explaining what your code is doing and what it is
not.
the problem is that when I close the MT4 and reopen the next day, I remember the same condition written in the graph before the MT4 closure and I need to update the chart for find the new condition
When you restart MT4, the data gets updated from the broker to the terminal.
You shouldn't need force a refresh, the chart data is automatically updated with the current candle values when it loads.
When that tick data comes in, your indicator should automatically recalculate it's output and adjust the display as required.
If your indicator isn't able to update objects, or delete objects, then it's probably a bug in your indicator.
Without the full indicator source code, it's pretty hard to figure out what is happening.
What other indicators are running on the chart?
Sorry my bad English!
NewBar () function bug. When I change graph I have this kind of problem, I initialize the lastbar variable = 0; initialbar initialization = 0; I find myself though that lastbar has the old time memory and all the code within NewBar is not read correctly, so I need to update the graph again manually. Is there any mistake I do not understand?
//+------------------------------------------------------------------+ //| Test.mq4 | //| Copyright 2017, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2017, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property indicator_chart_window static datetime lastbar[2]; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { lastbar[0]=0; lastbar[1]=0; lastbar[2]=0; lastbar[3]=0; //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator De initialization function | //+------------------------------------------------------------------+ int deinit() { lastbar[0]=0; lastbar[1]=0; lastbar[2]=0; Print("lastbar0: "+TimeToString(lastbar[0])); Print("lastbar1: "+TimeToString(lastbar[1])); Print("lastbar2: "+TimeToString(lastbar[2])); return(0); } //+------------------------------------------------------------------+ //| Funzione Per NewBar | //+------------------------------------------------------------------+ bool NewBar(int x){ datetime prevBar =lastbar[x]; lastbar[x]=Time[0]; return prevBar!=lastbar[x]; } //--------NewBar------------ //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { if(NewBar(0)){ Print("lastbar0: "+TimeToString(lastbar[0])); Print("lastbar1: "+TimeToString(lastbar[1])); Print("lastbar2: "+TimeToString(lastbar[2])); } return(rates_total); } //+------------------------------------------------------------------+
if I do not use the NewBar feature, I do not have any updating issues, but the code is too heavy for not having this feature

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I created a button that updates the chart and its objects but is just an escamotage, you have less rudimentary suggestions