tsedawa 2014.10.09 13:04
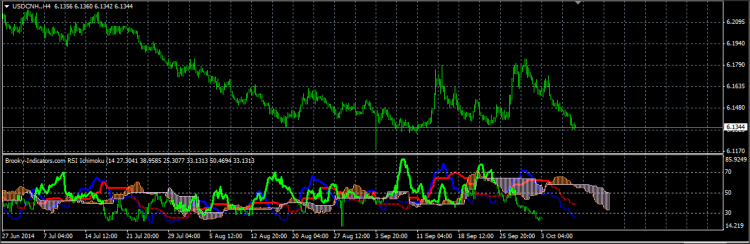
tsedawa:
read more about how to use iCustom name is wrong
hi,
can any one show me where is the failure in this code? (i even tried the shift as 26 instead of 0)
if(iCustom(NULL,240,"Brooky_Rsi_Ichimoku_V3.mq4",9,26,52,14,0,4,0)>67)
thanks
tsedawa:
hi,
thanks for the reply. u right i kept .mq4 in the name. thx
//Tsedawa14 extern double LM=1.3; extern double LM6=2; extern double lot=1; extern double increment=2; extern double TrailingStop=1; extern double pip=4; extern double pipsl=15; extern double piptp=10; extern double profit_target1=4000; extern double profit_target2=200; extern double curb_factor=0.5; extern double curb_factor3=1; extern int max_level1=5; extern int max_level=8; extern int bb_period=20; extern int bb_deviation=2; extern int bb_shift=0; extern int k=5; extern int d=3; extern int slowing=3; extern int price_field=0; extern int stoch_shift=0; extern int lo_level=25; extern int up_level=75; extern int rsi_period=14; extern int rsi_shift=0; extern int lower=25; extern int upper=75; extern int lo_level3=15; extern int up_level3=85; extern int lower3=15; extern int upper3=85; extern int MovingPeriod=12; extern int MovingShift=0; extern int magic=0; extern bool power_lots=false; extern bool closeall=false; extern int curb_level=2; //////////////////////////////////////////////////////////////////////////////////////// double pt,p_lot; double std=0.1; double AE1; double bal_2; double tf240=240; double ordertakeprofit; //+------------------------------------------------------------------+ //| expert initialization function | //+------------------------------------------------------------------+ int init() { //---- pt=Point; if(Digits==3 || Digits==5) pt=10*Point; //---- AE1=AccountEquity(); p_lot=lot/AE1; if(StringLen(Symbol())>6) std=1.0; profit_target1*=std; profit_target2*=std; //---- return(0); } //+------------------------------------------------------------------+ //| expert start function | //+------------------------------------------------------------------+ int start() { //---- int total=0, t_cnt=0, b_cnt=0, s_cnt=0, b2_cnt=0, s2_cnt=0; int b3_cnt=0, s3_cnt=0; double B_LOOP, B2_LOOP, S_LOOP, S2_LOOP; for(int i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; total++; //---- if(cmd()< 2) t_cnt++; if(cmd()==1){ s_cnt++; s3_cnt++; } if(cmd()==0){ b_cnt++; b3_cnt++; } if(s3_cnt==1 || (s3_cnt>1 && OOP()>S_LOOP)) S_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()<B_LOOP)) B_LOOP=OOP(); if(s3_cnt==1 || (s3_cnt>1 && OOP()<S2_LOOP)) S2_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()>B2_LOOP)) B2_LOOP=OOP(); } double o_charts=GlobalVariableGet("open_charts"); o_charts=1; // Global currently by-passed double AB=AccountBalance(), lot2=lot/o_charts, pl_factor=1/o_charts; double incr_1=increment*pl_factor, b_lot=lot2, s_lot=lot2; if(power_lots) { if(AB>AE1) lot2=NormalizeDouble((p_lot*AB)/o_charts,Digits); pl_factor=(lot2/lot)/o_charts; double lot3=lot2; } //+------------------------------------------------------------------+ bool t_buy=false, t_sell=false, t2_buy=false, t2_sell=false, t3_buy=false, t3_sell=false, t4_buy=false, t4_sell=false; if(t_cnt==0 || (b3_cnt==0 && s_cnt==0)) t_buy=true; if(t_cnt==0 || (s3_cnt==0 && b_cnt==0)) t_sell=true; if(b3_cnt==1) t2_buy=true; if(s3_cnt==1) t2_sell=true; if(b3_cnt>1 && b3_cnt<max_level1) t3_buy=true; if(s3_cnt>1 && s3_cnt<max_level1) t3_sell=true; if(b3_cnt>=max_level1 && b_cnt<max_level) t4_buy=true; if(s3_cnt>=max_level1 && s_cnt<max_level) t4_sell=true; //+------------------------------------------------------------------+ double ATR=NormalizeDouble(iATR(Symbol(),0,20,0),Digits); double atr=ATR; if(AgainstTrend()==true) atr=ATR*2; if(iRSI(Symbol(),240,14,PRICE_LOW,1)>=33) { if(t_sell || (t2_sell && Bid>=S_LOOP+atr) || (t3_sell && Bid>=S_LOOP+2*atr) || (t4_sell && Bid>=S_LOOP+NormalizeDouble(1.5*atr,Digits))) { if((s_cnt<1 && (signal2() == 1 || signal() == 1)) || (s_cnt>0 && s_cnt<3 && (signal2() == 1 || signal() == 1 || signal3() == 1)) || s_cnt>2) { if(s_cnt<1) s_lot=lot2; if(s_cnt<=2 && s_cnt>=1) s_lot=lot2+(incr_1*s_cnt); if(s_cnt==3) s_lot=(lot2+(incr_1*s_cnt))*LM; if(s_cnt>3) s_lot=(lot2+(incr_1*s_cnt))*LM6; OrderSend(Symbol(),OP_SELL,s_lot,Bid,3,0,0,"L"+(s_cnt+1),magic,0,Red); } } if((t2_sell && Bid<=S2_LOOP-atr*pt) || (t3_sell && Bid<=S2_LOOP-pt*NormalizeDouble(atr/2,Digits)) || (t4_sell && Bid<=S2_LOOP-pt*NormalizeDouble(atr/3,Digits))) { if(signal3() == 1 || signal() == 1) { OrderSend(Symbol(),1,s_lot,Bid,3,0,0,"L",magic,0,Red); } } } if(iRSI(Symbol(),240,14,PRICE_HIGH,1)<=67) { if(t_buy || (t2_buy && Ask<=B_LOOP-atr*pt) || (t3_buy && Ask<=B_LOOP-atr*2*pt) || (t4_buy && Ask<=B_LOOP-pt*NormalizeDouble(1.5*atr,Digits))) { if((b_cnt<1 && (signal2() == 0 || signal() == 0)) || (b_cnt>0 && b_cnt<3 && (signal2() == 0 || signal() == 0 || signal3() == 0)) || b_cnt>2) { if(b_cnt<1) b_lot=lot2; // L1 if(b_cnt<=2 && b_cnt>=1) b_lot=lot2+(incr_1*b_cnt); // L2,L3 if(b_cnt==3) b_lot=(lot2+(incr_1*b_cnt))*LM; // L4 if(b_cnt>3) b_lot=(lot2+(incr_1*b_cnt))*LM6; // L9 and onwards OrderSend(Symbol(),OP_BUY,b_lot,Ask,3,0,0,"L"+(b_cnt+1),magic,0,Blue); } } //--adding secondary trades to the winning primary trade L1 if((t2_buy && Ask>=B2_LOOP+atr*pt) || (t3_buy && Ask>=B2_LOOP+pt*NormalizeDouble(atr/2,Digits)) || (t4_buy && Ask>=B2_LOOP+pt*NormalizeDouble(atr/3,Digits))) { if(signal3() == 0 || signal() == 0) { OrderSend(Symbol(),0,b_lot,Ask,3,0,0,"L",magic,0,Blue); } } } //+------------------------------------------------------------------+ double ATR2=NormalizeDouble(iATR(Symbol(),0,20,0),Digits); double tp=ATR2; if(AgainstTrend()==true) tp=NormalizeDouble(ATR2/3,Digits); for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()==OP_SELL) tp*=-1; double otp=OOP()+tp*pt; if(OrderTakeProfit()==0) OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),otp,0,CLR_NONE); } //+------------------------------------------------------------------+ for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderComment()=="L1") ordertakeprofit=OrderTakeProfit(); if(t_cnt>1) { OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),ordertakeprofit,0,CLR_NONE); } } //+------------------------------------------------------------------+ // start trailing profit when the takeprofit is about to be hit. for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType() == OP_BUY) { double osl0=OrderTakeProfit()-pipsl*pt; double otp0=OrderTakeProfit()+piptp*pt; double cond0=OrderTakeProfit()-pip*pt; if(Bid>cond0) OrderModify(OrderTicket(),OrderOpenPrice(),osl0,otp0,0,CLR_NONE); } if(OrderType() == OP_SELL) { double osl1=OrderTakeProfit()+pipsl*pt; double otp1=OrderTakeProfit()-piptp*pt; double cond1=OrderTakeProfit()+pip*pt; if(Ask<cond1) OrderModify(OrderTicket(),OrderOpenPrice(),osl1,otp1,0,CLR_NONE); } } //+------------------------------------------------------------------+ double profit=0, profit_1=0, profit_2=0, profit_3=0; for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; profit+=OrderProfit(); if(t_cnt>2 && OT2()=="L") { if(OrderComment()=="L"+t_cnt) profit_1=OrderProfit(); if(OrderComment()=="L"+(t_cnt-1)) profit_2=OrderProfit(); profit_3=profit_1+profit_2; } } //+------------------------------------------------------------------+ double p_targ=profit_target1*pl_factor; if(AgainstTrend()==true) p_targ=profit_target2*pl_factor; for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(AgainstTrend()==false) break; if(curb_level<2 || t_cnt>15) curb_level=0; if(t_cnt>2) curb_factor=curb_factor3; if(OrderType()<2) { if(t_cnt>=curb_level && profit_3>=p_targ*curb_factor) { if(OrderComment()=="L"+t_cnt || OrderComment()=="L"+(t_cnt-1)) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } } //+------------------------------------------------------------------+ double balance=0; for(i=0; i<OrdersHistoryTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_HISTORY); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; balance+=OrderProfit(); } //---- if(t_cnt==0) GlobalVariableSet("bal_2"+Symbol()+magic,balance); bal_2=GlobalVariableGet("bal_2"+Symbol()+magic); if(balance+profit>=bal_2+p_targ) closeall=true; if(closeall) { for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()<2 && closeall && t_cnt>1) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } //+------------------------------------------------------------------+ string line_1="AccountLeverage = "+AccountLeverage()+" | Spread = "+DoubleToStr((Ask-Bid)/pt,2)+"\n"; //---- Comment(line_1); //---- return(0); } //+------------------------------------------------------------------+ // Functions //+------------------------------------------------------------------+ int cmd() { int cmd=OrderType(); return(cmd); } //---- double OOP() { double OOP=OrderOpenPrice(); return(OOP); } //---- string OCM() { string OCM=OrderComment(); return(OCM); } //---- string OT2() { string OT2=StringSubstr(OrderComment(),0,1); return(OT2); } //+------------------------------------------------------------------+ // to catch trades in a runaway trend int signal() { int signal=2; double MA0=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_LOW,0); double MA1=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_HIGH ,0); double DirectionMA1=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,1); double DirectionMA2=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,2); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(Ask<=MA0 && STDB!=EMPTY_VALUE) signal=0; if(Bid>=MA1 && STDS!=EMPTY_VALUE) signal=1; return(signal); } //+------------------------------------------------------------------+ int signal2() { int signal2=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(STDB!=EMPTY_VALUE) { if(Low[bb_shift]<loBB && (stoch<lo_level || rsi<lower)) signal2=0; if(stoch<lo_level && (Low[bb_shift]<loBB || rsi<lower)) signal2=0; if(rsi<lower && (stoch<lo_level || Low[bb_shift]<loBB)) signal2=0; } if(STDS!=EMPTY_VALUE) { if(High[bb_shift]>upBB && (stoch>up_level || rsi>upper)) signal2=1; if(stoch>up_level && (High[bb_shift]>upBB || rsi>upper)) signal2=1; if(rsi>upper && (stoch>up_level || High[bb_shift]>upBB)) signal2=1; } return(signal2); } //+------------------------------------------------------------------+ int signal3() { int signal3=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); if(Low[bb_shift]<loBB && (stoch<lo_level3 || rsi<lower3)) signal3=0; if(stoch<lo_level3 && (Low[bb_shift]<loBB || rsi<lower3)) signal3=0; if(rsi<lower3 && (stoch<lo_level3 || Low[bb_shift]<loBB)) signal3=0; if(High[bb_shift]>upBB && (stoch>up_level3 || rsi>upper3)) signal3=1; if(stoch>up_level3 && (High[bb_shift]>upBB || rsi>upper3)) signal3=1; if(rsi>upper3 && (stoch>up_level3 || High[bb_shift]>upBB)) signal3=1; return(signal3); } //+------------------------------------------------------------------+ bool AgainstTrend() { double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if((OrderType()==0 && STDS!=EMPTY_VALUE)||(OrderType()==1 && STDB!=EMPTY_VALUE)) return(true); return(false); } //+------------------------------------------------------------------+
//tsedawa15 extern double LM=1.3; extern double LM6=2; extern double lot=1; extern double increment=2; extern double trange=20; extern double trange2=15; extern double trange3=10; extern double rangeST=65; extern double rangeST2=85; extern double rangeST3=100; extern double range=35; extern double range2=45; extern double range3=65; extern double TrailingStop=1; extern double pip=4; extern double pipsl=15; extern double piptp=10; extern double profit_target1=4000; extern double profit_target2=200; extern double curb_factor=0.5; extern double curb_factor3=1; extern int max_level1=5; extern int max_level=8; extern int bb_period=20; extern int bb_deviation=2; extern int bb_shift=0; extern int k=5; extern int d=3; extern int slowing=3; extern int price_field=0; extern int stoch_shift=0; extern int lo_level=25; extern int up_level=75; extern int rsi_period=14; extern int rsi_shift=0; extern int lower=25; extern int upper=75; extern int lo_level3=15; extern int up_level3=85; extern int lower3=15; extern int upper3=85; extern int MovingPeriod=12; extern int MovingShift=0; extern int magic=0; extern bool power_lots=false; extern bool closeall=false; extern int curb_level=2; //////////////////////////////////////////////////////////////////////////////////////// double pt,p_lot; double std=0.1; double AE1; double bal_2; double tf240=240; double ordertakeprofit; //+------------------------------------------------------------------+ //| expert initialization function | //+------------------------------------------------------------------+ int init() { //---- pt=Point; if(Digits==3 || Digits==5) pt=10*Point; //---- AE1=AccountEquity(); p_lot=lot/AE1; if(StringLen(Symbol())>6) std=1.0; profit_target1*=std; profit_target2*=std; //---- return(0); } //+------------------------------------------------------------------+ //| expert start function | //+------------------------------------------------------------------+ int start() { //---- int total=0, t_cnt=0, b_cnt=0, s_cnt=0, b2_cnt=0, s2_cnt=0; int b3_cnt=0, s3_cnt=0; double B_LOOP, B2_LOOP, S_LOOP, S2_LOOP; for(int i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; total++; //---- if(cmd()< 2) t_cnt++; if(cmd()==1){ s_cnt++; s3_cnt++; } if(cmd()==0){ b_cnt++; b3_cnt++; } if(s3_cnt==1 || (s3_cnt>1 && OOP()>S_LOOP)) S_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()<B_LOOP)) B_LOOP=OOP(); if(s3_cnt==1 || (s3_cnt>1 && OOP()<S2_LOOP)) S2_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()>B2_LOOP)) B2_LOOP=OOP(); } double o_charts=GlobalVariableGet("open_charts"); o_charts=1; // Global currently by-passed double AB=AccountBalance(), lot2=lot/o_charts, pl_factor=1/o_charts; double incr_1=increment*pl_factor, b_lot=lot2, s_lot=lot2; if(power_lots) { if(AB>AE1) lot2=NormalizeDouble((p_lot*AB)/o_charts,Digits); pl_factor=(lot2/lot)/o_charts; double lot3=lot2; } //+------------------------------------------------------------------+ bool t_buy=false, t_sell=false, t2_buy=false, t2_sell=false, t3_buy=false, t3_sell=false, t4_buy=false, t4_sell=false; if(t_cnt==0 || (b3_cnt==0 && s_cnt==0)) t_buy=true; if(t_cnt==0 || (s3_cnt==0 && b_cnt==0)) t_sell=true; if(b3_cnt==1) t2_buy=true; if(s3_cnt==1) t2_sell=true; if(b3_cnt>1 && b3_cnt<max_level1) t3_buy=true; if(s3_cnt>1 && s3_cnt<max_level1) t3_sell=true; if(b3_cnt>=max_level1 && b_cnt<max_level) t4_buy=true; if(s3_cnt>=max_level1 && s_cnt<max_level) t4_sell=true; //+------------------------------------------------------------------+ if(AgainstTrend()==true) {range=rangeST; range2=rangeST2; range3=rangeST3;} if(iRSI(Symbol(),240,14,PRICE_LOW,1)>=33) { if(t_sell || (t2_sell && Bid>=S_LOOP+range) || (t3_sell && Bid>=S_LOOP+range2) || (t4_sell && Bid>=S_LOOP+range3)) { if((s_cnt<1 && (signal2() == 1 || signal() == 1)) || (s_cnt>0 && s_cnt<3 && (signal2() == 1 || signal() == 1 || signal3() == 1)) || s_cnt>2) { if(s_cnt<1) s_lot=lot2; if(s_cnt==1 || s_cnt==2) s_lot=lot2+(incr_1*s_cnt); if(s_cnt==3) s_lot=(lot2+(incr_1*s_cnt))*LM; if(s_cnt>3) s_lot=(lot2+(incr_1*s_cnt))*LM6; OrderSend(Symbol(),OP_SELL,s_lot,Bid,3,0,0,"L"+(s_cnt+1),magic,0,Red); } } } if(iRSI(Symbol(),240,14,PRICE_LOW,1)>=33) { if((t2_sell && Bid<=S2_LOOP-trange) || (t3_sell && Bid<=S2_LOOP-trange2) || (t4_sell && Bid<=S2_LOOP-trange3)) { if(signal3() == 1 || signal() == 1) { OrderSend(Symbol(),1,s_lot,Bid,3,0,0,"L",magic,0,Red); } } } if(iRSI(Symbol(),240,14,PRICE_HIGH,1)<=67) { if(t_buy || (t2_buy && Ask<=B_LOOP-range) || (t3_buy && Ask<=B_LOOP-range2) || (t4_buy && Ask<=B_LOOP-range3)) { if((b_cnt<1 && (signal2() == 0 || signal() == 0)) || (b_cnt>0 && b_cnt<3 && (signal2() == 0 || signal() == 0 || signal3() == 0)) || b_cnt>2) { if(b_cnt<1) b_lot=lot2; // L1 if(b_cnt==1 || b_cnt==2) b_lot=lot2+(incr_1*b_cnt); // L2,L3 if(b_cnt==3) b_lot=(lot2+(incr_1*b_cnt))*LM; // L4 if(b_cnt>3) b_lot=(lot2+(incr_1*b_cnt))*LM6; // L9 and onwards OrderSend(Symbol(),OP_BUY,b_lot,Ask,3,0,0,"L"+(b_cnt+1),magic,0,Blue); } } } //--adding secondary trades to the winning primary trade L1 if(iRSI(Symbol(),240,14,PRICE_HIGH,1)<=67) { if((t2_buy && Ask>=B2_LOOP+trange) || (t3_buy && Ask>=B2_LOOP+trange2) || (t4_buy && Ask>=B2_LOOP+trange3)) { if(signal3() == 0 || signal() == 0) { OrderSend(Symbol(),0,b_lot,Ask,3,0,0,"L",magic,0,Blue); } } } //+------------------------------------------------------------------+ double ATR2=NormalizeDouble(iATR(Symbol(),0,20,0),Digits); double tp=MathCeil(ATR2); if(AgainstTrend()==true) tp=MathCeil(ATR2/1.5); for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()==OP_SELL) tp*=-1; double otp=OOP()+tp*pt; if(OrderTakeProfit()==0) OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),otp,0,CLR_NONE); } //+------------------------------------------------------------------+ for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderComment()=="L1") ordertakeprofit=OrderTakeProfit(); if(t_cnt>1) { OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),ordertakeprofit,0,CLR_NONE); } } //+------------------------------------------------------------------+ // start trailing profit when the takeprofit is about to be hit. for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType() == OP_BUY) { double osl0=OrderTakeProfit()-pipsl*pt; double otp0=OrderTakeProfit()+piptp*pt; double cond0=OrderTakeProfit()-pip*pt; if(Bid>cond0) OrderModify(OrderTicket(),OrderOpenPrice(),osl0,otp0,0,CLR_NONE); } if(OrderType() == OP_SELL) { double osl1=OrderTakeProfit()+pipsl*pt; double otp1=OrderTakeProfit()-piptp*pt; double cond1=OrderTakeProfit()+pip*pt; if(Ask<cond1) OrderModify(OrderTicket(),OrderOpenPrice(),osl1,otp1,0,CLR_NONE); } } //+------------------------------------------------------------------+ double profit=0, profit_1=0, profit_2=0, profit_3=0; for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; profit+=OrderProfit(); if(t_cnt>2 && OT2()=="L") { if(OrderComment()=="L"+t_cnt) profit_1=OrderProfit(); if(OrderComment()=="L"+(t_cnt-1)) profit_2=OrderProfit(); profit_3=profit_1+profit_2; } } //+------------------------------------------------------------------+ double p_targ=profit_target1*pl_factor; if(AgainstTrend()==true) p_targ=profit_target2*pl_factor; for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(AgainstTrend()==false) break; if(curb_level<2 || t_cnt>15) curb_level=0; if(t_cnt>2) curb_factor=curb_factor3; if(OrderType()<2) { if(t_cnt>=curb_level && profit_3>=p_targ*curb_factor) { if(OrderComment()=="L"+t_cnt || OrderComment()=="L"+(t_cnt-1)) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } } //+------------------------------------------------------------------+ double balance=0; for(i=0; i<OrdersHistoryTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_HISTORY); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; balance+=OrderProfit(); } //---- if(t_cnt==0) GlobalVariableSet("bal_2"+Symbol()+magic,balance); bal_2=GlobalVariableGet("bal_2"+Symbol()+magic); if(balance+profit>=bal_2+p_targ) closeall=true; if(closeall) { for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()<2 && closeall && t_cnt>1) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } //+------------------------------------------------------------------+ string line_1="AccountLeverage = "+AccountLeverage()+" | Spread = "+DoubleToStr((Ask-Bid)/pt,2)+"\n"; //---- Comment(line_1); //---- return(0); } //+------------------------------------------------------------------+ // Functions //+------------------------------------------------------------------+ int cmd() { int cmd=OrderType(); return(cmd); } //---- double OOP() { double OOP=OrderOpenPrice(); return(OOP); } //---- string OCM() { string OCM=OrderComment(); return(OCM); } //---- string OT2() { string OT2=StringSubstr(OrderComment(),0,1); return(OT2); } //+------------------------------------------------------------------+ // to catch trades in a runaway trend int signal() { int signal=2; double MA0=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_LOW,0); double MA1=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_HIGH ,0); double DirectionMA1=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,1); double DirectionMA2=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,2); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(Ask<=MA0 && STDB!=EMPTY_VALUE) signal=0; if(Bid>=MA1 && STDS!=EMPTY_VALUE) signal=1; return(signal); } //+------------------------------------------------------------------+ int signal2() { int signal2=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(STDB!=EMPTY_VALUE) { if(Low[bb_shift]<loBB && (stoch<lo_level || rsi<lower)) signal2=0; if(stoch<lo_level && (Low[bb_shift]<loBB || rsi<lower)) signal2=0; if(rsi<lower && (stoch<lo_level || Low[bb_shift]<loBB)) signal2=0; } if(STDS!=EMPTY_VALUE) { if(High[bb_shift]>upBB && (stoch>up_level || rsi>upper)) signal2=1; if(stoch>up_level && (High[bb_shift]>upBB || rsi>upper)) signal2=1; if(rsi>upper && (stoch>up_level || High[bb_shift]>upBB)) signal2=1; } return(signal2); } //+------------------------------------------------------------------+ int signal3() { int signal3=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); if(Low[bb_shift]<loBB && (stoch<lo_level3 || rsi<lower3)) signal3=0; if(stoch<lo_level3 && (Low[bb_shift]<loBB || rsi<lower3)) signal3=0; if(rsi<lower3 && (stoch<lo_level3 || Low[bb_shift]<loBB)) signal3=0; if(High[bb_shift]>upBB && (stoch>up_level3 || rsi>upper3)) signal3=1; if(stoch>up_level3 && (High[bb_shift]>upBB || rsi>upper3)) signal3=1; if(rsi>upper3 && (stoch>up_level3 || High[bb_shift]>upBB)) signal3=1; return(signal3); } //+------------------------------------------------------------------+ bool AgainstTrend() { double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if((OrderType()==0 && STDS!=EMPTY_VALUE)||(OrderType()==1 && STDB!=EMPTY_VALUE)) return(true); return(false); } //+------------------------------------------------------------------+
//tsedawa13 extern double LM=1.3; extern double LM2=1.5; extern double LM3=1.7; extern double LM4=2; extern double LM5=2.4; extern double LM6=3; extern double lot=.01; extern double increment=.01; extern double trange=20; extern double trange2=15; extern double trange3=10; extern double rangeST=65; extern double rangeST2=85; extern double rangeST3=100; extern double range=35; extern double range2=45; extern double range3=65; extern double TrailingStop=1; extern double pip=4; extern double pipsl=20; extern double piptp=15; extern double tp=50; extern double tp2=15; extern double profit_target1=20; extern double profit_target2=.20; extern double curb_factor=0.5; extern double curb_factor3=1; extern int max_level1=5; extern int max_level=8; extern int bb_period=20; extern int bb_deviation=2; extern int bb_shift=0; extern int k=5; extern int d=3; extern int slowing=3; extern int price_field=0; extern int stoch_shift=0; extern int lo_level=25; extern int up_level=75; extern int rsi_period=14; extern int rsi_shift=0; extern int lower=25; extern int upper=75; extern int lo_level3=15; extern int up_level3=85; extern int lower3=15; extern int upper3=85; extern int MovingPeriod=12; extern int MovingShift=0; extern int magic=0; extern bool power_lots=false; extern bool closeall=false; extern int curb_level=2; //////////////////////////////////////////////////////////////////////////////////////// double pt,p_lot; double std=0.1; double AE1; double bal_2; double tf1440=1440; double tf240=240; double tf60=60; double tf15=15; double tf5=5; double Chinkou_Buffer; double atr, ordertakeprofit; //+------------------------------------------------------------------+ //| expert initialization function | //+------------------------------------------------------------------+ int init() { //---- pt=Point; if(Digits==3 || Digits==5) pt=10*Point; range*=pt; range2*=pt; range3*=pt; trange*=pt; trange2*=pt; trange3*=pt; rangeST*=pt; rangeST2*=pt; rangeST3*=pt; //---- AE1=AccountEquity(); p_lot=lot/AE1; if(StringLen(Symbol())>6) std=1.0; profit_target1*=std; profit_target2*=std; //---- return(0); } //+------------------------------------------------------------------+ //| expert start function | //+------------------------------------------------------------------+ int start() { //---- int total=0, t_cnt=0, b_cnt=0, s_cnt=0, b2_cnt=0, s2_cnt=0; int b3_cnt=0, s3_cnt=0; double B_LOOP, B2_LOOP, S_LOOP, S2_LOOP; for(int i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; total++; //---- if(cmd()< 2) t_cnt++; if(cmd()==1){ s_cnt++; s3_cnt++; } if(cmd()==0){ b_cnt++; b3_cnt++; } if(s3_cnt==1 || (s3_cnt>1 && OOP()>S_LOOP)) S_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()<B_LOOP)) B_LOOP=OOP(); if(s3_cnt==1 || (s3_cnt>1 && OOP()<S2_LOOP)) S2_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()>B2_LOOP)) B2_LOOP=OOP(); } double o_charts=GlobalVariableGet("open_charts"); o_charts=1; // Global currently by-passed double AB=AccountBalance(), lot2=lot/o_charts, pl_factor=1/o_charts; double incr_1=increment*pl_factor, b_lot=lot2, s_lot=lot2; if(power_lots) { if(AB>AE1) lot2=NormalizeDouble((p_lot*AB)/o_charts,Digits); pl_factor=(lot2/lot)/o_charts; double lot3=lot2; } //+------------------------------------------------------------------+ bool t_buy=false, t_sell=false, t2_buy=false, t2_sell=false, t3_buy=false, t3_sell=false, t4_buy=false, t4_sell=false; if(t_cnt==0 || (b3_cnt==0 && s_cnt==0)) t_buy=true; if(t_cnt==0 || (s3_cnt==0 && b_cnt==0)) t_sell=true; if(b3_cnt==1) t2_buy=true; if(s3_cnt==1) t2_sell=true; if(b3_cnt>1 && b3_cnt<max_level1) t3_buy=true; if(s3_cnt>1 && s3_cnt<max_level1) t3_sell=true; if(b3_cnt>=max_level1 && b_cnt<max_level) t4_buy=true; if(s3_cnt>=max_level1 && s_cnt<max_level) t4_sell=true; //+------------------------------------------------------------------+ if(AgainstTrend()==true) {range=rangeST; range2=rangeST2; range3=rangeST3;} if(SellAllowed()==1) { if(t_sell || (t2_sell && Bid>=S_LOOP+range) || (t3_sell && Bid>=S_LOOP+range2) || (t4_sell && Bid>=S_LOOP+range3)) { if((s_cnt<1 && (signal2() == 1 || signal() == 1)) || (s_cnt>0 && s_cnt<3 && (signal2() == 1 || signal() == 1 || signal3() == 1)) || s_cnt>2) { if(s_cnt<1) s_lot=lot2; if(s_cnt<=2 && s_cnt>=1) s_lot=lot2+(incr_1*s_cnt); if(s_cnt==3) s_lot=(lot2+(incr_1*s_cnt))*LM; if(s_cnt==4) s_lot=(lot2+(incr_1*s_cnt))*LM2; if(s_cnt==5) s_lot=(lot2+(incr_1*s_cnt))*LM3; if(s_cnt==6) s_lot=(lot2+(incr_1*s_cnt))*LM4; if(s_cnt==7) s_lot=(lot2+(incr_1*s_cnt))*LM5; if(s_cnt>7) s_lot=(lot2+(incr_1*s_cnt))*LM6; OrderSend(Symbol(),OP_SELL,s_lot,Bid,3,0,0,"L"+(s_cnt+1),magic,0,Red); } } } if(BuyAllowed()==0) { if(t_buy || (t2_buy && Ask<=B_LOOP-range) || (t3_buy && Ask<=B_LOOP-range2) || (t4_buy && Ask<=B_LOOP-range3)) { if((b_cnt<1 && (signal2() == 0 || signal() == 0)) || (b_cnt>0 && b_cnt<3 && (signal2() == 0 || signal() == 0 || signal3() == 0)) || b_cnt>2) { if(b_cnt<1) b_lot=lot2; // L1 if(b_cnt<=2 && b_cnt>=1) b_lot=lot2+(incr_1*b_cnt); // L2,L3 if(b_cnt==3) b_lot=(lot2+(incr_1*b_cnt))*LM; // L4 if(b_cnt==4) b_lot=(lot2+(incr_1*b_cnt))*LM2; // L5 if(b_cnt==5) b_lot=(lot2+(incr_1*b_cnt))*LM3; // L6 if(b_cnt==6) b_lot=(lot2+(incr_1*b_cnt))*LM4; // L7 if(b_cnt==7) b_lot=(lot2+(incr_1*b_cnt))*LM5; // L8 if(b_cnt>7) b_lot=(lot2+(incr_1*b_cnt))*LM6; // L9 and onwards OrderSend(Symbol(),OP_BUY,b_lot,Ask,3,0,0,"L"+(b_cnt+1),magic,0,Blue); } } } //--adding secondary trades to the winning primary trade L1 if(BuyAllowed()==0) { if((t2_buy && Ask>=B2_LOOP+trange) || (t3_buy && Ask>=B2_LOOP+trange2) || (t4_buy && Ask>=B2_LOOP+trange3)) { if(signal3() == 0 || signal() == 0) { OrderSend(Symbol(),0,b_lot,Ask,3,0,0,"L",magic,0,Blue); } } } if(SellAllowed()==1) { if((t2_sell && Bid<=S2_LOOP-trange) || (t3_sell && Bid<=S2_LOOP-trange2) || (t4_sell && Bid<=S2_LOOP-trange3)) { if(signal3() == 1 || signal() == 1) { OrderSend(Symbol(),1,s_lot,Bid,3,0,0,"L",magic,0,Red); } } } //+------------------------------------------------------------------+ for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(AgainstTrend()==true) tp=tp2; if(OrderType()==OP_SELL) tp*=-1; double otp=OOP()+tp*pt; if(OrderTakeProfit()==0) OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),otp,0,CLR_NONE); } //+------------------------------------------------------------------+ for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderComment()=="L1") ordertakeprofit=OrderTakeProfit(); if(t_cnt>1) { OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),ordertakeprofit,0,CLR_NONE); } } //+------------------------------------------------------------------+ // start trailing profit when the takeprofit is about to be hit. for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType() == OP_BUY) { double osl0=OrderTakeProfit()-pipsl*pt; double otp0=OrderTakeProfit()+piptp*pt; double cond0=OrderTakeProfit()-pip*pt; if(Bid>cond0) OrderModify(OrderTicket(),OrderOpenPrice(),osl0,otp0,0,CLR_NONE); } if(OrderType() == OP_SELL) { double osl1=OrderTakeProfit()+pipsl*pt; double otp1=OrderTakeProfit()-piptp*pt; double cond1=OrderTakeProfit()+pip*pt; if(Ask<cond1) OrderModify(OrderTicket(),OrderOpenPrice(),osl1,otp1,0,CLR_NONE); } } //+------------------------------------------------------------------+ double profit=0, profit_1=0, profit_2=0, profit_3=0; for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; profit+=OrderProfit(); if(t_cnt>2 && OT2()=="L") { if(OrderComment()=="L"+t_cnt) profit_1=OrderProfit(); if(OrderComment()=="L"+(t_cnt-1)) profit_2=OrderProfit(); profit_3=profit_1+profit_2; } } //+------------------------------------------------------------------+ double p_targ=profit_target1*pl_factor; if(AgainstTrend()==true) p_targ=profit_target2*pl_factor; for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(AgainstTrend()==false) break; if(curb_level<2 || t_cnt>15) curb_level=0; if(t_cnt>2) curb_factor=curb_factor3; if(OrderType()<2) { if(t_cnt>=curb_level && profit_3>=p_targ*curb_factor) { if(OrderComment()=="L"+t_cnt || OrderComment()=="L"+(t_cnt-1)) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } } //+------------------------------------------------------------------+ double balance=0; for(i=0; i<OrdersHistoryTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_HISTORY); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; balance+=OrderProfit(); } //---- if(t_cnt==0) GlobalVariableSet("bal_2"+Symbol()+magic,balance); bal_2=GlobalVariableGet("bal_2"+Symbol()+magic); if(balance+profit>=bal_2+p_targ) closeall=true; if(closeall) { for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()<2 && closeall && t_cnt>1) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } //+------------------------------------------------------------------+ string line_1="AccountLeverage = "+AccountLeverage()+" | Spread = "+DoubleToStr((Ask-Bid)/pt,2)+"\n"; //---- Comment(line_1); //---- return(0); } //+------------------------------------------------------------------+ // Functions //+------------------------------------------------------------------+ int cmd() { int cmd=OrderType(); return(cmd); } //---- double OOP() { double OOP=OrderOpenPrice(); return(OOP); } //---- string OCM() { string OCM=OrderComment(); return(OCM); } //---- string OT2() { string OT2=StringSubstr(OrderComment(),0,1); return(OT2); } //+------------------------------------------------------------------+ // to catch trades in a runaway trend int signal() { int signal=2; double MA0=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_LOW,0); double MA1=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_HIGH ,0); double DirectionMA1=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,1); double DirectionMA2=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,2); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(Ask<=MA0 && STDB!=EMPTY_VALUE) signal=0; if(Bid>=MA1 && STDS!=EMPTY_VALUE) signal=1; return(signal); } //+------------------------------------------------------------------+ int signal2() { int signal2=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(STDB!=EMPTY_VALUE) { if(Low[bb_shift]<loBB && (stoch<lo_level || rsi<lower)) signal2=0; if(stoch<lo_level && (Low[bb_shift]<loBB || rsi<lower)) signal2=0; if(rsi<lower && (stoch<lo_level || Low[bb_shift]<loBB)) signal2=0; } if(STDS!=EMPTY_VALUE) { if(High[bb_shift]>upBB && (stoch>up_level || rsi>upper)) signal2=1; if(stoch>up_level && (High[bb_shift]>upBB || rsi>upper)) signal2=1; if(rsi>upper && (stoch>up_level || High[bb_shift]>upBB)) signal2=1; } return(signal2); } //+------------------------------------------------------------------+ int signal3() { int signal3=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); if(Low[bb_shift]<loBB && (stoch<lo_level3 || rsi<lower3)) signal3=0; if(stoch<lo_level3 && (Low[bb_shift]<loBB || rsi<lower3)) signal3=0; if(rsi<lower3 && (stoch<lo_level3 || Low[bb_shift]<loBB)) signal3=0; if(High[bb_shift]>upBB && (stoch>up_level3 || rsi>upper3)) signal3=1; if(stoch>up_level3 && (High[bb_shift]>upBB || rsi>upper3)) signal3=1; if(rsi>upper3 && (stoch>up_level3 || High[bb_shift]>upBB)) signal3=1; return(signal3); } //+------------------------------------------------------------------+ bool AgainstTrend() { double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if((OrderType()==0 && STDS!=EMPTY_VALUE)||(OrderType()==1 && STDB!=EMPTY_VALUE)) return(true); return(false); } //+------------------------------------------------------------------+ int BuyAllowed() { int BuyAllowed=0; if(iCustom(NULL,240,"Brooky_Rsi_Ichimoku_V3",9,26,52,14,0,4,26)>67) BuyAllowed=1; return(BuyAllowed); } //+------------------------------------------------------------------+ int SellAllowed() { int SellAllowed=1; if(iCustom(NULL,240,"Brooky_Rsi_Ichimoku_V3",9,26,52,14,0,4,26)<33) SellAllowed=0; return(SellAllowed); } //+------------------------------------------------------------------+
//tsedawa extern double LM=1.3; extern double LM6=2; extern double lot=1; extern double increment=2; extern double TrailingStop=1; extern double pip=4; extern double pipsl=15; extern double piptp=10; extern double profit_target1=4000; extern double profit_target2=200; extern double curb_factor=0.5; extern double curb_factor3=1; extern int max_level1=5; extern int max_level=8; extern int bb_period=20; extern int bb_deviation=2; extern int bb_shift=0; extern int k=5; extern int d=3; extern int slowing=3; extern int price_field=0; extern int stoch_shift=0; extern int lo_level=25; extern int up_level=75; extern int rsi_period=14; extern int rsi_shift=0; extern int lower=25; extern int upper=75; extern int lo_level3=15; extern int up_level3=85; extern int lower3=15; extern int upper3=85; extern int MovingPeriod=12; extern int MovingShift=0; extern int magic=0; extern bool power_lots=false; extern bool closeall=false; extern int curb_level=2; //////////////////////////////////////////////////////////////////////////////////////// double pt,p_lot; double std=0.1; double AE1; double bal_2; double tf240=240; double ordertakeprofit; //+------------------------------------------------------------------+ //| expert initialization function | //+------------------------------------------------------------------+ int init() { //---- pt=Point; if(Digits==3 || Digits==5) pt=10*Point; //---- AE1=AccountEquity(); p_lot=lot/AE1; if(StringLen(Symbol())>6) std=1.0; profit_target1*=std; profit_target2*=std; //---- return(0); } //+------------------------------------------------------------------+ //| expert start function | //+------------------------------------------------------------------+ int start() { //---- int total=0, t_cnt=0, b_cnt=0, s_cnt=0, b2_cnt=0, s2_cnt=0; int b3_cnt=0, s3_cnt=0; double B_LOOP, B2_LOOP, S_LOOP, S2_LOOP; for(int i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; total++; //---- if(cmd()< 2) t_cnt++; if(cmd()==1){ s_cnt++; s3_cnt++; } if(cmd()==0){ b_cnt++; b3_cnt++; } if(s3_cnt==1 || (s3_cnt>1 && OOP()>S_LOOP)) S_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()<B_LOOP)) B_LOOP=OOP(); if(s3_cnt==1 || (s3_cnt>1 && OOP()<S2_LOOP)) S2_LOOP=OOP(); if(b3_cnt==1 || (b3_cnt>1 && OOP()>B2_LOOP)) B2_LOOP=OOP(); } double o_charts=GlobalVariableGet("open_charts"); o_charts=1; // Global currently by-passed double AB=AccountBalance(), lot2=lot/o_charts, pl_factor=1/o_charts; double incr_1=increment*pl_factor, b_lot=lot2, s_lot=lot2; if(power_lots) { if(AB>AE1) lot2=NormalizeDouble((p_lot*AB)/o_charts,Digits); pl_factor=(lot2/lot)/o_charts; double lot3=lot2; } //+------------------------------------------------------------------+ bool t_buy=false, t_sell=false, t2_buy=false, t2_sell=false, t3_buy=false, t3_sell=false, t4_buy=false, t4_sell=false; if(t_cnt==0 || (b3_cnt==0 && s_cnt==0)) t_buy=true; if(t_cnt==0 || (s3_cnt==0 && b_cnt==0)) t_sell=true; if(b3_cnt==1) t2_buy=true; if(s3_cnt==1) t2_sell=true; if(b3_cnt>1 && b3_cnt<max_level1) t3_buy=true; if(s3_cnt>1 && s3_cnt<max_level1) t3_sell=true; if(b3_cnt>=max_level1 && b_cnt<max_level) t4_buy=true; if(s3_cnt>=max_level1 && s_cnt<max_level) t4_sell=true; //+------------------------------------------------------------------+ double ATR=NormalizeDouble(iATR(Symbol(),0,20,0),Digits); double atr=ATR; if(AgainstTrend()==true) atr=ATR*2; if(iRSI(Symbol(),240,14,PRICE_LOW,1)>=33) { if(t_sell || (t2_sell && Bid>=S_LOOP+atr*pt) || (t3_sell && Bid>=S_LOOP+2*atr*pt) || (t4_sell && Bid>=S_LOOP+NormalizeDouble(1.5*atr*pt,Digits))) { if((s_cnt<1 && (signal2() == 1 || signal() == 1)) || (s_cnt>0 && s_cnt<3 && (signal2() == 1 || signal() == 1 || signal3() == 1)) || s_cnt>2) { if(s_cnt<1) s_lot=lot2; if(s_cnt<=2 && s_cnt>=1) s_lot=lot2+(incr_1*s_cnt); if(s_cnt==3) s_lot=(lot2+(incr_1*s_cnt))*LM; if(s_cnt>3) s_lot=(lot2+(incr_1*s_cnt))*LM6; OrderSend(Symbol(),OP_SELL,s_lot,Bid,3,0,0,"L"+(s_cnt+1),magic,0,Red); } } } if(iRSI(Symbol(),240,14,PRICE_LOW,1)>=33) { if((t2_sell && Bid<=S2_LOOP-atr*pt) || (t3_sell && Bid<=S2_LOOP-NormalizeDouble(pt*atr/2,Digits)) || (t4_sell && Bid<=S2_LOOP-NormalizeDouble(pt*atr/3,Digits))) { if(signal3() == 1 || signal() == 1) { OrderSend(Symbol(),1,s_lot,Bid,3,0,0,"L",magic,0,Red); } } } if(iRSI(Symbol(),240,14,PRICE_HIGH,1)<=67) { if(t_buy || (t2_buy && Ask<=B_LOOP-atr*pt) || (t3_buy && Ask<=B_LOOP-atr*2*pt) || (t4_buy && Ask<=B_LOOP-NormalizeDouble(1.5*atr*pt,Digits))) { if((b_cnt<1 && (signal2() == 0 || signal() == 0)) || (b_cnt>0 && b_cnt<3 && (signal2() == 0 || signal() == 0 || signal3() == 0)) || b_cnt>2) { if(b_cnt<1) b_lot=lot2; // L1 if(b_cnt<=2 && b_cnt>=1) b_lot=lot2+(incr_1*b_cnt); // L2,L3 if(b_cnt==3) b_lot=(lot2+(incr_1*b_cnt))*LM; // L4 if(b_cnt>3) b_lot=(lot2+(incr_1*b_cnt))*LM6; // L9 and onwards OrderSend(Symbol(),OP_BUY,b_lot,Ask,3,0,0,"L"+(b_cnt+1),magic,0,Blue); } } } //--adding secondary trades to the winning primary trade L1 if(iRSI(Symbol(),240,14,PRICE_HIGH,1)<=67) { if((t2_buy && Ask>=B2_LOOP+atr*pt) || (t3_buy && Ask>=B2_LOOP+NormalizeDouble(pt*atr/2,Digits)) || (t4_buy && Ask>=B2_LOOP+NormalizeDouble(pt*atr/3,Digits))) { if(signal3() == 0 || signal() == 0) { OrderSend(Symbol(),0,b_lot,Ask,3,0,0,"L",magic,0,Blue); } } } //+------------------------------------------------------------------+ double ATR2=NormalizeDouble(iATR(Symbol(),0,20,0),Digits); double tp=MathCeil(ATR2); if(AgainstTrend()==true) tp=MathCeil(ATR2/1.5); for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()==OP_SELL) tp*=-1; double otp=OOP()+tp*pt; if(OrderTakeProfit()==0) OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),otp,0,CLR_NONE); } //+------------------------------------------------------------------+ for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderComment()=="L1") ordertakeprofit=OrderTakeProfit(); if(t_cnt>1) { OrderModify(OrderTicket(),OrderOpenPrice(),OrderStopLoss(),ordertakeprofit,0,CLR_NONE); } } //+------------------------------------------------------------------+ // start trailing profit when the takeprofit is about to be hit. for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType() == OP_BUY) { double osl0=OrderTakeProfit()-pipsl*pt; double otp0=OrderTakeProfit()+piptp*pt; double cond0=OrderTakeProfit()-pip*pt; if(Bid>cond0) OrderModify(OrderTicket(),OrderOpenPrice(),osl0,otp0,0,CLR_NONE); } if(OrderType() == OP_SELL) { double osl1=OrderTakeProfit()+pipsl*pt; double otp1=OrderTakeProfit()-piptp*pt; double cond1=OrderTakeProfit()+pip*pt; if(Ask<cond1) OrderModify(OrderTicket(),OrderOpenPrice(),osl1,otp1,0,CLR_NONE); } } //+------------------------------------------------------------------+ double profit=0, profit_1=0, profit_2=0, profit_3=0; for(i=0; i<OrdersTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; profit+=OrderProfit(); if(t_cnt>2 && OT2()=="L") { if(OrderComment()=="L"+t_cnt) profit_1=OrderProfit(); if(OrderComment()=="L"+(t_cnt-1)) profit_2=OrderProfit(); profit_3=profit_1+profit_2; } } //+------------------------------------------------------------------+ double p_targ=profit_target1*pl_factor; if(AgainstTrend()==true) p_targ=profit_target2*pl_factor; for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(AgainstTrend()==false) break; if(curb_level<2 || t_cnt>15) curb_level=0; if(t_cnt>2) curb_factor=curb_factor3; if(OrderType()<2) { if(t_cnt>=curb_level && profit_3>=p_targ*curb_factor) { if(OrderComment()=="L"+t_cnt || OrderComment()=="L"+(t_cnt-1)) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } } //+------------------------------------------------------------------+ double balance=0; for(i=0; i<OrdersHistoryTotal(); i++) { OrderSelect(i,SELECT_BY_POS,MODE_HISTORY); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; balance+=OrderProfit(); } //---- if(t_cnt==0) GlobalVariableSet("bal_2"+Symbol()+magic,balance); bal_2=GlobalVariableGet("bal_2"+Symbol()+magic); if(balance+profit>=bal_2+p_targ) closeall=true; if(closeall) { for(i=OrdersTotal()-1; i>=0; i--) { OrderSelect(i,SELECT_BY_POS,MODE_TRADES); if(OrderSymbol()!=Symbol() || OrderMagicNumber()!=magic) continue; if(OrderType()<2 && closeall && t_cnt>1) OrderClose(OrderTicket(),OrderLots(),OrderClosePrice(),3,CLR_NONE); } } //+------------------------------------------------------------------+ string line_1="AccountLeverage = "+AccountLeverage()+" | Spread = "+DoubleToStr((Ask-Bid)/pt,2)+"\n"; //---- Comment(line_1); //---- return(0); } //+------------------------------------------------------------------+ // Functions //+------------------------------------------------------------------+ int cmd() { int cmd=OrderType(); return(cmd); } //---- double OOP() { double OOP=OrderOpenPrice(); return(OOP); } //---- string OCM() { string OCM=OrderComment(); return(OCM); } //---- string OT2() { string OT2=StringSubstr(OrderComment(),0,1); return(OT2); } //+------------------------------------------------------------------+ // to catch trades in a runaway trend int signal() { int signal=2; double MA0=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_LOW,0); double MA1=iMA(NULL,240,MovingPeriod,MovingShift,MODE_SMA,PRICE_HIGH ,0); double DirectionMA1=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,1); double DirectionMA2=iMA(NULL,0,MovingPeriod,MovingShift,MODE_SMA,PRICE_CLOSE,2); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(Ask<=MA0 && STDB!=EMPTY_VALUE) signal=0; if(Bid>=MA1 && STDS!=EMPTY_VALUE) signal=1; return(signal); } //+------------------------------------------------------------------+ int signal2() { int signal2=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if(STDB!=EMPTY_VALUE) { if(Low[bb_shift]<loBB && (stoch<lo_level || rsi<lower)) signal2=0; if(stoch<lo_level && (Low[bb_shift]<loBB || rsi<lower)) signal2=0; if(rsi<lower && (stoch<lo_level || Low[bb_shift]<loBB)) signal2=0; } if(STDS!=EMPTY_VALUE) { if(High[bb_shift]>upBB && (stoch>up_level || rsi>upper)) signal2=1; if(stoch>up_level && (High[bb_shift]>upBB || rsi>upper)) signal2=1; if(rsi>upper && (stoch>up_level || High[bb_shift]>upBB)) signal2=1; } return(signal2); } //+------------------------------------------------------------------+ int signal3() { int signal3=2; double upBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_UPPER,bb_shift); double loBB=iBands(NULL,0,bb_period,bb_deviation,0,PRICE_CLOSE,MODE_LOWER,bb_shift); double stoch=iStochastic(NULL,0,k,d,slowing,MODE_SMA,price_field,MODE_SIGNAL,stoch_shift); double rsi=iRSI(NULL,0,rsi_period,PRICE_CLOSE,rsi_shift); if(Low[bb_shift]<loBB && (stoch<lo_level3 || rsi<lower3)) signal3=0; if(stoch<lo_level3 && (Low[bb_shift]<loBB || rsi<lower3)) signal3=0; if(rsi<lower3 && (stoch<lo_level3 || Low[bb_shift]<loBB)) signal3=0; if(High[bb_shift]>upBB && (stoch>up_level3 || rsi>upper3)) signal3=1; if(stoch>up_level3 && (High[bb_shift]>upBB || rsi>upper3)) signal3=1; if(rsi>upper3 && (stoch>up_level3 || High[bb_shift]>upBB)) signal3=1; return(signal3); } //+------------------------------------------------------------------+ bool AgainstTrend() { double STDB=iCustom(NULL,240,"SuperTrend",10,3.0,0,0); double STDS=iCustom(NULL,240,"SuperTrend",10,3.0,1,0); if((OrderType()==0 && STDS!=EMPTY_VALUE)||(OrderType()==1 && STDB!=EMPTY_VALUE)) return(true); return(false); } //+------------------------------------------------------------------+

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register