Markov chains can be applied to intraday trading to model price movements and predict future price levels based on current and past price levels. Here's a step-by-step guide on how to use Markov chains for intraday trading:
Step 1: Define the States
Define discrete states based on price levels or returns. For example, you can divide price levels into ranges and assign each range a state:
- State 1: Price decreases significantly
- State 2: Price decreases slightly
- State 3: Price remains stable
- State 4: Price increases slightly
- State 5: Price increases significantly
Step 2: Collect Historical Data
Collect intraday price data, such as minute-by-minute or tick data. Calculate the returns or price changes for each time interval.
Step 3: Calculate Transition Probabilities
Calculate the transition probabilities between states using historical data. This involves counting how often each state transitions to another state. For example, if the price was in State 1 at time t t t and moved to State 2 at time t + 1 t+1 t+1, this counts as a transition from State 1 to State 2.
The transition probability matrix P P P can be constructed where each element P i j P_{ij} Pij represents the probability of moving from state i i i to state j j j:
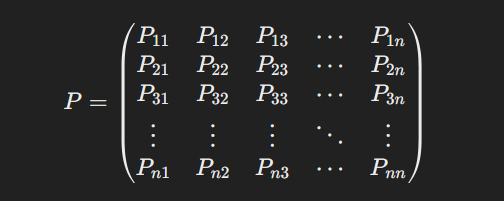
Each element P i j P_{ij} Pij is calculated as:
Step 4: Use the Markov Chain for Predictions
Use the transition probability matrix to predict future states. Given the current state, the future state probabilities can be calculated by multiplying the current state vector with the transition matrix.
If the current state vector is s0
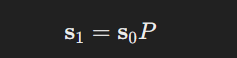
Step 5: Develop a Trading Strategy
Based on the predicted future states, develop a trading strategy. For example, if the model predicts a high probability of price increase (transitioning to an increasing state), you might decide to buy. Conversely, if a price decrease is predicted, you might decide to sell or short the asset.
Example in MQL5
Below is an example of how you might implement a simple Markov chain-based strategy in MQL5:
// Define the states enum PriceState { DECREASE_SIGNIFICANTLY, DECREASE_SLIGHTLY, STABLE, INCREASE_SLIGHTLY, INCREASE_SIGNIFICANTLY }; // Function to determine the current state based on price change PriceState GetState(double priceChange) { if (priceChange < -0.5) return DECREASE_SIGNIFICANTLY; if (priceChange < -0.1) return DECREASE_SLIGHTLY; if (priceChange < 0.1) return STABLE; if (priceChange < 0.5) return INCREASE_SLIGHTLY; return INCREASE_SIGNIFICANTLY; } // Transition matrix (example values) double transitionMatrix[5][5] = { {0.2, 0.3, 0.2, 0.2, 0.1}, {0.1, 0.3, 0.4, 0.1, 0.1}, {0.1, 0.2, 0.4, 0.2, 0.1}, {0.1, 0.1, 0.3, 0.4, 0.1}, {0.1, 0.1, 0.2, 0.3, 0.3} }; // Function to predict next state PriceState PredictNextState(PriceState currentState) { double rnd = MathRand() / (double)RAND_MAX; double cumulativeProbability = 0.0; for (int i = 0; i < 5; i++) { cumulativeProbability += transitionMatrix[currentState][i]; if (rnd <= cumulativeProbability) { return (PriceState)i; } } return currentState; // Default case } // OnTick function to execute the strategy void OnTick() { static PriceState currentState = STABLE; static double previousClose = 0.0; double currentClose = iClose(Symbol(), PERIOD_M1, 0); if (previousClose != 0.0) { double priceChange = (currentClose - previousClose) / previousClose * 100; currentState = GetState(priceChange); // Predict next state PriceState nextState = PredictNextState(currentState); // Trading logic based on predicted state if (nextState == INCREASE_SIGNIFICANTLY || nextState == INCREASE_SLIGHTLY) { // Buy logic if (PositionSelect(Symbol()) == false) { OrderSend(Symbol(), OP_BUY, 0.1, Ask, 2, 0, 0, "Buy Order", 0, 0, Green); } } else if (nextState == DECREASE_SIGNIFICANTLY || nextState == DECREASE_SLIGHTLY) { // Sell logic if (PositionSelect(Symbol()) == true) { OrderSend(Symbol(), OP_SELL, 0.1, Bid, 2, 0, 0, "Sell Order", 0, 0, Red); } } } previousClose = currentClose; }
Key Points:
- Define states: Based on price changes.
- Calculate transition probabilities: From historical data.
- Predict future states: Using the transition matrix.
- Develop strategy: Buy or sell based on predicted states.
Using Markov chains helps in capturing the probabilistic nature of price movements and can be a useful tool in developing trading strategies.