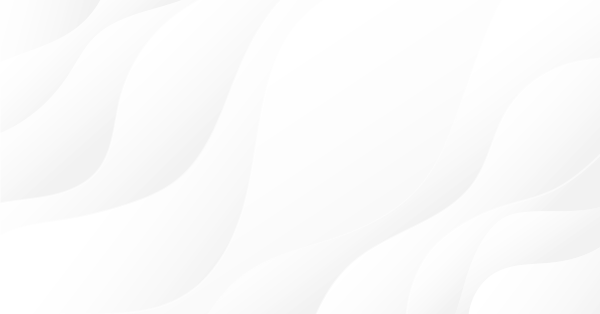
Trabalhando com preços na biblioteca DoEasy (Parte 60): lista-série de dados de dados de tick do símbolo
Sumário
- Ideia
- Aprimorando as classes da biblioteca
- Classe do objeto para a série de dados de tick
- Testando a criação de lista e aquisição de dados
- O que vem agora?
Ideia
No último artigo começamos a criar funcionalidades para trabalhar com dados de tick. Criamos uma classe de objeto de dados de tick. Hoje vamos criar uma lista para armazenar objetos desse tipo. As listas estarão disponíveis cada um dos símbolos usados no programa. Por padrão, o tamanho das listas de dados de tick para um símbolo cobrirá o dia atual. Naturalmente, será possível definir a número de dias para o qual o programa terá um conjunto de dados de tick.
Por que precisamos ter nossas próprias listas de dados de tick, se a linguagem MQL5 permite que você os obtenha a qualquer momento que precisar? Acontece que, com essas listas de dados de tick em nossas mãos, podemos procurar nelas de maneira conveniente e rápida informações de que precisamos, comparar e receber dados. Essa capacidade é fornecida pelo próprio conceito de criação de listas na biblioteca.
Depois de ter as listas criadas para cada símbolo utilizado pelo programa, iremos combiná-las posteriormente numa coleção de dados de tick, com a ajuda disso tornaremos conveniente trabalhar com dados de tick de qualquer símbolo, o que nos permite transportar qualquer pesquisa com fluxos de ticks de diferentes símbolos.
Aprimorando as classes da biblioteca
Em primeiro lugar, vamos adicionar novas mensagens da biblioteca no arquivo \MQL5\Include\DoEasy\Data.mqh. Adicionamos os índices das novas mensagens:
//--- CTick MSG_TICK_TEXT_TICK, // Tick MSG_TICK_TIME_MSC, // Time of the last update of prices in milliseconds MSG_TICK_TIME, // Time of the last update of prices MSG_TICK_VOLUME, // Volume for the current Last price MSG_TICK_FLAGS, // Flags MSG_TICK_VOLUME_REAL, // Volume for the current Last price with greater accuracy MSG_TICK_SPREAD, // Spread MSG_LIB_TEXT_TICK_CHANGED_DATA, // Changed data on tick: MSG_LIB_TEXT_TICK_FLAG_BID, // Bid price change MSG_LIB_TEXT_TICK_FLAG_ASK, // Ask price change MSG_LIB_TEXT_TICK_FLAG_LAST, // Last deal price change MSG_LIB_TEXT_TICK_FLAG_VOLUME, // Volume change //--- CTickSeries MSG_TICKSERIES_TEXT_TICKSERIES, // Tick series MSG_TICKSERIES_ERR_GET_TICK_DATA, // Failed to get tick data MSG_TICKSERIES_FAILED_CREATE_TICK_DATA_OBJ, // Failed to create tick data object MSG_TICKSERIES_FAILED_ADD_TO_LIST, // Failed to add tick data object to list MSG_TICKSERIES_TEXT_IS_NOT_USE, // Tick series not used. Set the flag using SetAvailable() MSG_TICKSERIES_REQUIRED_HISTORY_DAYS, // Requested number of days }; //+------------------------------------------------------------------+
e as mensagens de texto correspondentes aos índices adicionados recentemente:
//--- CTick {"Тик","Tick"}, {"Время последнего обновления цен в миллисекундах","Last price update time in milliseconds"}, {"Время последнего обновления цен","Last price update time"}, {"Объем для текущей цены Last","Volume for the current Last price"}, {"Флаги","Flags"}, {"Объем для текущей цены Last c повышенной точностью","Volume for the current \"Last\" price with increased accuracy"}, {"Спред","Spread"}, {"Изменённые данные на тике:","Changed data on a tick:"}, {"Изменение цены Bid","Bid price change"}, {"Изменение цены Ask","Ask price change"}, {"Изменение цены последней сделки","Last price change"}, {"Изменение объема","Volume change"}, //--- TickSeries {"Тиковая серия","Tickseries"}, {"Ошибка получения тиковых данных","Error getting tick data"}, {"Не удалось создать объект тиковых данных","Failed to create tick data object"}, {"Не удалось добавить объект тиковых данных в список","Failed to add tick data object to the list"}, {"Тиковая серия не используется. Нужно установить флаг использования при помощи SetAvailable()","Tick series are not used. Need to set the use flag using SetAvailable()"}, {"Запрошенное количество дней: ","Number of days requested: "}, }; //+---------------------------------------------------------------------+
Por padrão, iremos armazenar dados de tick para o dia atual. Para especificar o número de dias durante os quais a biblioteca armazenará ticks, no arquivo \MQL5\Include\DoEasy\Defines.mqh vamos introduzir uma nova constante (substituição macro):
//--- Timeseries parameters #define SERIES_DEFAULT_BARS_COUNT (1000) // Required default amount of timeseries data #define PAUSE_FOR_SYNC_ATTEMPTS (16) // Amount of pause milliseconds between synchronization attempts #define ATTEMPTS_FOR_SYNC (5) // Number of attempts to receive synchronization with the server //--- Tick series parameters #define TICKSERIES_DEFAULT_DAYS_COUNT (1) // Required number of days for tick data in default series
Ao analisar novamente o código da classe da série temporal no arquivo \MQL5\Include\DoEasy\Objects\Series\SeriesDE.mqh, apercebi-me de um erro: se o objeto-barra criado por algum motivo não era adicionado à lista, ele não era excluído. Isso pode causar uma perda de memória. Portanto, no método para criar uma lista-séries temporais adicionamos a exclusão de objeto se não for adicionado à lista:
//+------------------------------------------------------------------+ //| Create the timeseries list | //+------------------------------------------------------------------+ int CSeriesDE::Create(const uint required=0) { //--- If the required history depth is not set for the list yet, //--- display the appropriate message and return zero, if(this.m_amount==0) { ::Print(DFUN,this.m_symbol," ",TimeframeDescription(this.m_timeframe),": ",CMessage::Text(MSG_LIB_TEXT_BAR_TEXT_FIRS_SET_AMOUNT_DATA)); return 0; } //--- otherwise, if the passed 'required' value exceeds zero and is not equal to the one already set, //--- while being lower than the available bar number, //--- set the new value of the required history depth for the list else if(required>0 && this.m_amount!=required && required<this.m_bars) { //--- If failed to set a new value, return zero if(!this.SetRequiredUsedData(required,0)) return 0; } //--- For the rates[] array we are to receive historical data to, //--- set the flag of direction like in the timeseries, //--- clear the bar object list and set the flag of sorting by bar index MqlRates rates[]; ::ArraySetAsSeries(rates,true); this.m_list_series.Clear(); this.m_list_series.Sort(SORT_BY_BAR_TIME); ::ResetLastError(); //--- Get historical data of the MqlRates structure to the rates[] array starting from the current bar in the amount of m_amount, //--- if failed to get data, display the appropriate message and return zero int copied=::CopyRates(this.m_symbol,this.m_timeframe,0,(uint)this.m_amount,rates),err=ERR_SUCCESS; if(copied<1) { err=::GetLastError(); ::Print(DFUN,CMessage::Text(MSG_LIB_TEXT_BAR_FAILED_GET_SERIES_DATA)," ",this.m_symbol," ",TimeframeDescription(this.m_timeframe),". ", CMessage::Text(MSG_LIB_SYS_ERROR),": ",CMessage::Text(err),CMessage::Retcode(err)); return 0; } //--- Historical data is received in the rates[] array //--- In the rates[] array loop, for(int i=0; i<copied; i++) { //--- create a new bar object out of the current MqlRates structure by the loop index ::ResetLastError(); CBar* bar=new CBar(this.m_symbol,this.m_timeframe,rates[i]); if(bar==NULL) { ::Print ( DFUN,CMessage::Text(MSG_LIB_SYS_FAILED_CREATE_BAR_OBJ)," ",this.Header()," ",::TimeToString(rates[i].time),". ", CMessage::Text(MSG_LIB_SYS_ERROR),": ",CMessage::Text(::GetLastError()) ); continue; } //--- If failed to add bar object to the list, //--- display the appropriate message with the error description in the journal //--- and remove the newly created object if(!this.m_list_series.Add(bar)) { err=::GetLastError(); ::Print(DFUN,CMessage::Text(MSG_LIB_TEXT_BAR_FAILED_ADD_TO_LIST)," ",bar.Header()," ",::TimeToString(rates[i].time),". ", CMessage::Text(MSG_LIB_SYS_ERROR),": ",CMessage::Text(err),CMessage::Retcode(err)); delete bar; } } //--- Return the size of the created bar object list return this.m_list_series.Total(); } //+------------------------------------------------------------------+
Para criar a capacidade de pesquisar, classificar e selecionar objetos-tick da lista criada, precisamos adicionar ao arquivo
\MQL5\Include\DoEasy\Services\Select.mqh métodos para trabalhar com esta lista e com dados de tick.
Anexamos o arquivo de classe do objeto de dados tick à classe:
//+------------------------------------------------------------------+ //| Select.mqh | //| Copyright 2020, MetaQuotes Software Corp. | //| https://mql5.com/en/users/artmedia70 | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://mql5.com/en/users/artmedia70" #property version "1.00" //+------------------------------------------------------------------+ //| Include files | //+------------------------------------------------------------------+ #include <Arrays\ArrayObj.mqh> #include "..\Objects\Orders\Order.mqh" #include "..\Objects\Events\Event.mqh" #include "..\Objects\Accounts\Account.mqh" #include "..\Objects\Symbols\Symbol.mqh" #include "..\Objects\PendRequest\PendRequest.mqh" #include "..\Objects\Series\SeriesDE.mqh" #include "..\Objects\Indicators\Buffer.mqh" #include "..\Objects\Indicators\IndicatorDE.mqh" #include "..\Objects\Indicators\DataInd.mqh" #include "..\Objects\Ticks\DataTick.mqh" //+------------------------------------------------------------------+
No final do corpo da classe escrevemos as declarações de métodos para trabalhar com dados de tick:
//+------------------------------------------------------------------+ //| Methods of work with indicator data | //+------------------------------------------------------------------+ //--- Return the list of indicator data with one out of (1) integer, (2) real and (3) string properties meeting a specified criterion static CArrayObj *ByIndicatorDataProperty(CArrayObj *list_source,ENUM_IND_DATA_PROP_INTEGER property,long value,ENUM_COMPARER_TYPE mode); static CArrayObj *ByIndicatorDataProperty(CArrayObj *list_source,ENUM_IND_DATA_PROP_DOUBLE property,double value,ENUM_COMPARER_TYPE mode); static CArrayObj *ByIndicatorDataProperty(CArrayObj *list_source,ENUM_IND_DATA_PROP_STRING property,string value,ENUM_COMPARER_TYPE mode); //--- Return the indicator data index in the list with the maximum value of (1) integer, (2) real and (3) string property of data static int FindIndDataMax(CArrayObj *list_source,ENUM_IND_DATA_PROP_INTEGER property); static int FindIndDataMax(CArrayObj *list_source,ENUM_IND_DATA_PROP_DOUBLE property); static int FindIndDataMax(CArrayObj *list_source,ENUM_IND_DATA_PROP_STRING property); //--- Return the indicator data index in the list with the minimum value of (1) integer, (2) real and (3) string property of data static int FindIndDataMin(CArrayObj *list_source,ENUM_IND_DATA_PROP_INTEGER property); static int FindIndDataMin(CArrayObj *list_source,ENUM_IND_DATA_PROP_DOUBLE property); static int FindIndDataMin(CArrayObj *list_source,ENUM_IND_DATA_PROP_STRING property); //+------------------------------------------------------------------+ //| Methods of working with tick data | //+------------------------------------------------------------------+ //--- Return the list of tick data with one out of (1) integer, (2) real and (3) string properties meeting a specified criterion static CArrayObj *ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_INTEGER property,long value,ENUM_COMPARER_TYPE mode); static CArrayObj *ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_DOUBLE property,double value,ENUM_COMPARER_TYPE mode); static CArrayObj *ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_STRING property,string value,ENUM_COMPARER_TYPE mode); //--- Return the tick data index in the list with the maximum value of (1) integer, (2) real and (3) string property of data static int FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_INTEGER property); static int FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_DOUBLE property); static int FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_STRING property); //--- Return the tick data index in the list with the minimum value of (1) integer, (2) real and (3) string property of data static int FindTickDataMin(CArrayObj *list_source,ENUM_TICK_PROP_INTEGER property); static int FindTickDataMin(CArrayObj *list_source,ENUM_TICK_PROP_DOUBLE property); static int FindTickDataMin(CArrayObj *list_source,ENUM_TICK_PROP_STRING property); //--- }; //+------------------------------------------------------------------+
Fora do corpo da classe, escrevemos a implementação dos métodos declarados:
//+------------------------------------------------------------------+ //| Methods of working with tick data lists | //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Return the list of tick data with one of integer | //| property meeting the specified criterion | //+------------------------------------------------------------------+ CArrayObj *CSelect::ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_INTEGER property,long value,ENUM_COMPARER_TYPE mode) { if(list_source==NULL) return NULL; CArrayObj *list=new CArrayObj(); if(list==NULL) return NULL; list.FreeMode(false); ListStorage.Add(list); int total=list_source.Total(); for(int i=0; i<total; i++) { CDataTick *obj=list_source.At(i); if(!obj.SupportProperty(property)) continue; long obj_prop=obj.GetProperty(property); if(CompareValues(obj_prop,value,mode)) list.Add(obj); } return list; } //+------------------------------------------------------------------+ //| Return the list of tick data with one of real | //| property meeting the specified criterion | //+------------------------------------------------------------------+ CArrayObj *CSelect::ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_DOUBLE property,double value,ENUM_COMPARER_TYPE mode) { if(list_source==NULL) return NULL; CArrayObj *list=new CArrayObj(); if(list==NULL) return NULL; list.FreeMode(false); ListStorage.Add(list); for(int i=0; i<list_source.Total(); i++) { CDataTick *obj=list_source.At(i); if(!obj.SupportProperty(property)) continue; double obj_prop=obj.GetProperty(property); if(CompareValues(obj_prop,value,mode)) list.Add(obj); } return list; } //+------------------------------------------------------------------+ //| Return the list of tick data with one of string | //| property meeting the specified criterion | //+------------------------------------------------------------------+ CArrayObj *CSelect::ByTickDataProperty(CArrayObj *list_source,ENUM_TICK_PROP_STRING property,string value,ENUM_COMPARER_TYPE mode) { if(list_source==NULL) return NULL; CArrayObj *list=new CArrayObj(); if(list==NULL) return NULL; list.FreeMode(false); ListStorage.Add(list); for(int i=0; i<list_source.Total(); i++) { CDataTick *obj=list_source.At(i); if(!obj.SupportProperty(property)) continue; string obj_prop=obj.GetProperty(property); if(CompareValues(obj_prop,value,mode)) list.Add(obj); } return list; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the maximum integer property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_INTEGER property) { if(list_source==NULL) return WRONG_VALUE; int index=0; CDataTick *max_obj=NULL; int total=list_source.Total(); if(total==0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); long obj1_prop=obj.GetProperty(property); max_obj=list_source.At(index); long obj2_prop=max_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,MORE)) index=i; } return index; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the maximum real property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_DOUBLE property) { if(list_source==NULL) return WRONG_VALUE; int index=0; CDataTick *max_obj=NULL; int total=list_source.Total(); if(total==0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); double obj1_prop=obj.GetProperty(property); max_obj=list_source.At(index); double obj2_prop=max_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,MORE)) index=i; } return index; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the maximum string property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMax(CArrayObj *list_source,ENUM_TICK_PROP_STRING property) { if(list_source==NULL) return WRONG_VALUE; int index=0; CDataTick *max_obj=NULL; int total=list_source.Total(); if(total==0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); string obj1_prop=obj.GetProperty(property); max_obj=list_source.At(index); string obj2_prop=max_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,MORE)) index=i; } return index; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the minimum integer property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMin(CArrayObj* list_source,ENUM_TICK_PROP_INTEGER property) { int index=0; CDataTick *min_obj=NULL; int total=list_source.Total(); if(total==0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); long obj1_prop=obj.GetProperty(property); min_obj=list_source.At(index); long obj2_prop=min_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,LESS)) index=i; } return index; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the minimum real property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMin(CArrayObj* list_source,ENUM_TICK_PROP_DOUBLE property) { int index=0; CDataTick *min_obj=NULL; int total=list_source.Total(); if(total== 0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); double obj1_prop=obj.GetProperty(property); min_obj=list_source.At(index); double obj2_prop=min_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,LESS)) index=i; } return index; } //+------------------------------------------------------------------+ //| Return the tick data in the list | //| with the minimum string property value | //+------------------------------------------------------------------+ int CSelect::FindTickDataMin(CArrayObj* list_source,ENUM_TICK_PROP_STRING property) { int index=0; CDataTick *min_obj=NULL; int total=list_source.Total(); if(total==0) return WRONG_VALUE; for(int i=1; i<total; i++) { CDataTick *obj=list_source.At(i); string obj1_prop=obj.GetProperty(property); min_obj=list_source.At(index); string obj2_prop=min_obj.GetProperty(property); if(CompareValues(obj1_prop,obj2_prop,LESS)) index=i; } return index; } //+------------------------------------------------------------------+
Discutimos repetidamente o trabalho desses métodos e podemos saber mais sobre eles com mais detalhes no artigo 3.
Classe do objeto para a série de dados de tick
Agora vamos escrever a classe do objeto-lista de dados de tick. A lista, como todas as outras na biblioteca, será uma matriz baseada numa classe de uma array dinâmica de ponteiros para instâncias da classe CObject e seus herdeiros.
Iremos calcular o início do dia necessário, dependendo do número especificado de dias para os quais precisamos armazenar o histórico de ticks, e a partir desse dia listaremos todos os ticks disponíveis usando CopyTicksRange(). No próximo artigo, geraremos uma atualização em tempo real dessas listas, para ter sempre um banco de dados de tick atualizado na coleção de nosso programa.
A classe do objeto da lista de dados de tick é semelhante em estrutura à classe da lista-séries temporais do símbolo. Só que no primeiro caso a unidade mínima de armazenamento de dados é o objeto-barra, e já neste caso é o objeto-tick. A composição da classe será padrão para a biblioteca, por isso vamos considerar seu corpo na íntegra e, a seguir, esclarecer alguns detalhes e métodos:
//+------------------------------------------------------------------+ //| TickSeries.mqh | //| Copyright 2020, MetaQuotes Software Corp. | //| https://mql5.com/en/users/artmedia70 | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://mql5.com/en/users/artmedia70" #property version "1.00" #property strict // Necessary for mql4 //+------------------------------------------------------------------+ //| Include files | //+------------------------------------------------------------------+ #include "..\..\Services\Select.mqh" #include "NewTickObj.mqh" #include "DataTick.mqh" //+------------------------------------------------------------------+ //| "Tick data series" class | //+------------------------------------------------------------------+ class CTickSeries : public CBaseObj { private: string m_symbol; // Symbol uint m_amount; // Amount of applied tick series data uint m_required; // Required number of days for tick series data CArrayObj m_list_ticks; // List of tick data CNewTickObj m_new_tick_obj; // "New tick" object public: //--- Return (1) itself, (2) list of tick data and (3) "New tick" object of the tick series CTickSeries *GetObject(void) { return &this; } CArrayObj *GetList(void) { return &m_list_ticks; } CNewTickObj *GetNewTickObj(void) { return &this.m_new_tick_obj;} //--- Return the list of tick objects by selected (1) double, (2) integer and (3) string property fitting a compared condition CArrayObj *GetList(ENUM_TICK_PROP_DOUBLE property,double value,ENUM_COMPARER_TYPE mode=EQUAL){ return CSelect::ByTickDataProperty(this.GetList(),property,value,mode); } CArrayObj *GetList(ENUM_TICK_PROP_INTEGER property,long value,ENUM_COMPARER_TYPE mode=EQUAL) { return CSelect::ByTickDataProperty(this.GetList(),property,value,mode); } CArrayObj *GetList(ENUM_TICK_PROP_STRING property,string value,ENUM_COMPARER_TYPE mode=EQUAL){ return CSelect::ByTickDataProperty(this.GetList(),property,value,mode); } //--- Return the object of tick data by (1) index in the list, (2) time and (4) list size CDataTick *GetTickByListIndex(const uint index); CDataTick *GetTick(const datetime time); CDataTick *GetTick(const ulong time_msc); int DataTotal(void) const { return this.m_list_ticks.Total(); } //--- The comparison method for searching identical tick series objects by a symbol virtual int Compare(const CObject *node,const int mode=0) const { const CTickSeries *compared_obj=node; return(this.Symbol()==compared_obj.Symbol() ? 0 : this.Symbol()>compared_obj.Symbol() ? 1 : -1); } //--- Return the tick series name string Header(void); //--- Display (1) the tick series description and (2) the tick series short description in the journal void Print(void); void PrintShort(void); //--- Constructors CTickSeries(void){;} CTickSeries(const string symbol,const uint required=0); //+------------------------------------------------------------------+ //| Methods of working with objects and accessing their properties | //+------------------------------------------------------------------+ //--- Set (1) a symbol and (2) a number of used tick series data void SetSymbol(const string symbol); void SetRequiredUsedBars(const uint required=0); //--- Return (1) symbol, (2) number of used, (3) requested tick data and (4) new tick flag string Symbol(void) const { return this.m_symbol; } ulong AvailableUsedData(void) const { return this.m_amount; } ulong RequiredUsedDays(void) const { return this.m_required; } bool IsNewTick(void) { return this.m_new_tick_obj.IsNewTick(); } //--- Return (1) Bid, (2) Ask, (3) Last, (4) volume with increased accuracy, //--- (5) spread, (6) volume, (7) tick flags, (8) time, (9) time in milliseconds by index in the list double Bid(const uint index); double Ask(const uint index); double Last(const uint index); double VolumeReal(const uint index); double Spread(const uint index); long Volume(const uint index); uint Flags(const uint index); datetime Time(const uint index); long TimeMSC(const uint index); //--- Return (1) Bid, (2) Ask, (3) Last, (4) volume with increased accuracy, //--- (5) spread, (6) volume, (7) tick flags by tick time in milliseconds double Bid(const ulong time_msc); double Ask(const ulong time_msc); double Last(const ulong time_msc); double VolumeReal(const ulong time_msc); double Spread(const ulong time_msc); long Volume(const ulong time_msc); uint Flags(const ulong time_msc); //--- Return (1) Bid, (2) Ask, (3) Last, (4) volume with increased accuracy, //--- (5) spread, (6) volume and (7) tick flags by tick time double Bid(const datetime time); double Ask(const datetime time); double Last(const datetime time); double VolumeReal(const datetime time); double Spread(const datetime time); long Volume(const datetime time); uint Flags(const datetime time); //--- (1) Create and (2) update the timeseries list int Create(const uint required=0); void Refresh(void); }; //+------------------------------------------------------------------+
Listagens de classes do objeto são anexadas à classe "Novo tick" e "Dados do tick" que escrevemos anteriormente. No próximo artigo, precisaremos do objeto "novo tick" ao atualizar as listas de dados de tick, e o objeto "dados de tick" será uma classe dos objetos que colocaremos na lista.
Na seção privada da classe são declaradas todas as variáveis-membro de classe necessárias para armazenar os valores dos parâmetros do objeto, um objeto da classe da lista de objetos (que será a própria lista de ticks) e o objeto novo tick, que será exigido no próximo artigo quando a lista for atualizada em tempo real.
A seção pública da classe contém métodos padrão para objetos de biblioteca que permitem o funcionamento do objeto, métodos para acessar as propriedades do objeto-lista de dados de tick, métodos para acesso simplificado às propriedades do objeto especificado de dados de tick localizado na lista, e métodos para criar e atualizar a lista.
Vamos considerar os métodos do objeto.
No construtor paramétrico de classe limpamos a lista, definimos para ele o sinalizador de lista classificada por tempo de tick em milissegundos e definimos o número necessário de dias para o qual precisamos ter um histórico de ticks usando o método SetRequiredUsedDays().
//+------------------------------------------------------------------+ //| Parametric constructor | //+------------------------------------------------------------------+ CTickSeries::CTickSeries(const string symbol,const uint required=0) : m_symbol(symbol) { this.m_list_ticks.Clear(); this.m_list_ticks.Sort(SORT_BY_TICK_TIME_MSC); this.SetRequiredUsedDays(required); } //+------------------------------------------------------------------+
Método para definir o símbolo da lista de ticks:
//+------------------------------------------------------------------+ //| Set a symbol | //+------------------------------------------------------------------+ void CTickSeries::SetSymbol(const string symbol) { if(this.m_symbol==symbol) return; this.m_symbol=(symbol==NULL || symbol=="" ? ::Symbol() : symbol); } //+------------------------------------------------------------------+
Se o símbolo passado para o método já foi definido, saímos o método, caso contrário, se o valor passado é NULL ou uma string vazia, então definimos o símbolo atual, caso contrário, definimos o símbolo passado para o método.
Método para definir o número de dias para o qual os dados de tick são necessários:
//+------------------------------------------------------------------+ //| Set the number of required tick data | //+------------------------------------------------------------------+ void CTickSeries::SetRequiredUsedDays(const uint required=0) { this.m_required=(required<1 ? TICKSERIES_DEFAULT_DAYS_COUNT : required); } //+------------------------------------------------------------------+
Se ao método for transferido um valor igual a zero ou menor que zero, definiremos o número de dias padrão, caso contrário, estabeleceremos o número de dias transferido.
Método que retorna um objeto-tick da lista com base no índice da lista:
//+------------------------------------------------------------------+ //| Return the tick object by its index in the list | //+------------------------------------------------------------------+ CDataTick *CTickSeries::GetTickByListIndex(const uint index) { return this.m_list_ticks.At(index); } //+------------------------------------------------------------------+
Simplesmente retornamos o objeto que está na lista pelo índice passado ao método. Deve-se ter em mente que o método At() retornará NULL se um índice inválido for especificado ou se a lista estiver vazia.
Método que retorna um objeto-tick da lista de acordo com seu tempo:
//+------------------------------------------------------------------+ //| Return the last tick object by its time | //+------------------------------------------------------------------+ CDataTick *CTickSeries::GetTick(const datetime time) { CArrayObj *list=GetList(TICK_PROP_TIME,time,EQUAL); if(list==NULL) return NULL; return list.At(list.Total()-1); } //+------------------------------------------------------------------+
Obtemos uma lista de objetos-ticks cujo tempo corresponde ao transferido ao método e retornamos o mais recente.
O ponto é que diferentes ticks podem ter o mesmo tempo, por isso o mais recente é retornado aqui.
Método que retorna um objeto-tick da lista com base no seu tempo em milissegundos:
//+------------------------------------------------------------------+ //| Return the last tick object by its time in milliseconds | //+------------------------------------------------------------------+ CDataTick *CTickSeries::GetTick(const ulong time_msc) { CArrayObj *list=GetList(TICK_PROP_TIME_MSC,time_msc,EQUAL); if(list==NULL) return NULL; return list.At(list.Total()-1); } //+------------------------------------------------------------------+
Obtemos uma lista de objetos-ticks para os quais o tempo em milissegundos corresponde ao passado para o método, e retornamos o mais recente.
Aqui, assim como no método que acabamos de considerar, diferentes ticks podem ter o mesmo tempo em milissegundos, portanto, neste caso, o mais recente é retornado com a expectativa de sua maior relevância.
Os outros métodos para obter objetos-tick serão idênticos entre si e terão três sobrecargas - obter pelo índice da lista, obter por tempo e obter por tempo em milissegundos. Vamos considerar esses três métodos para obter o preço Bid do tick e, para os demais métodos idênticos, simplesmente forneceremos sua listagem.
Método que retorna o preço Bid de um objeto-tick por seu índice na lista:
//+------------------------------------------------------------------+ //| Return tick's Bid by index in the list | //+------------------------------------------------------------------+ double CTickSeries::Bid(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Bid() : 0); } //+------------------------------------------------------------------+
Obtemos um objeto da lista com base no índice passado para o método e retornamos um Bid do objeto recebido ou 0 (se o objeto não puder ser obtido)
Método que retorna o preço Bid do último objeto-tick na lista de acordo com seu tempo em milissegundos:
//+------------------------------------------------------------------+ //| Return tick's Bid by time in milliseconds | //+------------------------------------------------------------------+ double CTickSeries::Bid(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Bid() : 0); } //+------------------------------------------------------------------+
Pegamos o último objeto da lista pelo tempo passado para o método em milissegundos (acima consideramos esse método) e retornamos o Bid do objeto recebido ou 0 (se o objeto não pode ser recebido)
Método que retorna o preço Bid do último objeto-tick na lista de acordo com seu tempo:
//+------------------------------------------------------------------+ //| Return tick's Bid by time | //+------------------------------------------------------------------+ double CTickSeries::Bid(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Bid() : 0); } //+------------------------------------------------------------------+
Pegamos o último objeto da lista de acordo com o tempo passado para o método (acima consideramos esse método de recepção) e retornamos o Bid a partir do objeto recebido ou 0 (se o objeto não pôde ser obtido)
Os métodos restantes para obter os valores das propriedades dos objetos-tick a partir da lista são idênticos aos três acima, e deixaremos sua análise para estudá-la individualmente.
//+------------------------------------------------------------------+ //| Return tick's Ask by index in the list | //+------------------------------------------------------------------+ double CTickSeries::Ask(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Ask() : 0); } //+------------------------------------------------------------------+ //| Return tick's Ask by time in milliseconds | //+------------------------------------------------------------------+ double CTickSeries::Ask(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Ask() : 0); } //+------------------------------------------------------------------+ //| Return tick's Ask by time | //+------------------------------------------------------------------+ double CTickSeries::Ask(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Ask() : 0); } //+------------------------------------------------------------------+ //| Return tick's Last by index in the list | //+------------------------------------------------------------------+ double CTickSeries::Last(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Last() : 0); } //+------------------------------------------------------------------+ //| Return tick's Last by time in milliseconds | //+------------------------------------------------------------------+ double CTickSeries::Last(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Last() : 0); } //+------------------------------------------------------------------+ //| Return tick's Last by time | //+------------------------------------------------------------------+ double CTickSeries::Last(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Last() : 0); } //+-------------------------------------------------------------------------+ //| Return the volume with the increased tick accuracy by index in the list | //+-------------------------------------------------------------------------+ double CTickSeries::VolumeReal(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.VolumeReal() : 0); } //+--------------------------------------------------------------------------+ //|Return the volume with the increased tick accuracy by time in milliseconds| //+--------------------------------------------------------------------------+ double CTickSeries::VolumeReal(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.VolumeReal() : 0); } //+------------------------------------------------------------------+ //| Return the volume with the increased tick accuracy by time | //+------------------------------------------------------------------+ double CTickSeries::VolumeReal(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.VolumeReal() : 0); } //+------------------------------------------------------------------+ //| Return the tick spread by index in the list | //+------------------------------------------------------------------+ double CTickSeries::Spread(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Spread() : 0); } //+------------------------------------------------------------------+ //| Return tick's spread by time in milliseconds | //+------------------------------------------------------------------+ double CTickSeries::Spread(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Spread() : 0); } //+------------------------------------------------------------------+ //| Return tick's spread by time | //+------------------------------------------------------------------+ double CTickSeries::Spread(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Spread() : 0); } //+------------------------------------------------------------------+ //| Return the tick volume by index in the list | //+------------------------------------------------------------------+ long CTickSeries::Volume(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Volume() : 0); } //+------------------------------------------------------------------+ //| Return tick's volume by time in milliseconds | //+------------------------------------------------------------------+ long CTickSeries::Volume(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Volume() : 0); } //+------------------------------------------------------------------+ //| Return tick's volume by time | //+------------------------------------------------------------------+ long CTickSeries::Volume(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Volume() : 0); } //+------------------------------------------------------------------+ //| Return the tick flags by index in the list | //+------------------------------------------------------------------+ uint CTickSeries::Flags(const uint index) { CDataTick *tick=this.GetTickByListIndex(index); return(tick!=NULL ? tick.Flags() : 0); } //+------------------------------------------------------------------+ //| Return the tick flags by time in milliseconds | //+------------------------------------------------------------------+ uint CTickSeries::Flags(const ulong time_msc) { CDataTick *tick=this.GetTick(time_msc); return(tick!=NULL ? tick.Flags() : 0); } //+------------------------------------------------------------------+ //| Return the tick flags by time | //+------------------------------------------------------------------+ uint CTickSeries::Flags(const datetime time) { CDataTick *tick=this.GetTick(time); return(tick!=NULL ? tick.Flags() : 0); } //+------------------------------------------------------------------+
Cada um dos métodos é representado por três métodos sobrecarregado idênticos que permitem obter um objeto-tick por seu índice na lista e pelo tempo, tanto no formato de data quanto em milissegundos.
Método que retorna o nome de string da série de tick:
//+------------------------------------------------------------------+ //| Return the tick series name | //+------------------------------------------------------------------+ string CTickSeries::Header(void) { return CMessage::Text(MSG_TICKSERIES_TEXT_TICKSERIES)+" \""+this.m_symbol+"\""; } //+------------------------------------------------------------------+
Retorna uma string no formato
Tickseries "symbol name"
Por exemplo:
Série de tick "EURUSD"
Método que imprime no log uma descrição completa de uma série de tick:
//+------------------------------------------------------------------+ //| Display the tick series description in the journal | //+------------------------------------------------------------------+ void CTickSeries::Print(void) { string txt= ( CMessage::Text(MSG_TICKSERIES_REQUIRED_HISTORY_DAYS)+(string)this.RequiredUsedDays()+", "+ CMessage::Text(MSG_LIB_TEXT_TS_AMOUNT_HISTORY_DATA)+(string)this.DataTotal() ); ::Print(this.Header(),": ",txt); } //+------------------------------------------------------------------+
O método cria uma string a partir da descrição do número de dias durante os quais os ticks são armazenados na série e quantos ticks são realmente armazenados na lista.
Em seguida, o cabeçalho da série de tick e a string gerada são impressos. Por exemplo:
Série de tick "EURUSD": número de dias solicitados: 1, dados históricos criados: 256714
Método que imprime no log uma breve descrição de uma série de ticks:
//+------------------------------------------------------------------+ //| Display the brief tick series description in the journal | //+------------------------------------------------------------------+ void CTickSeries::PrintShort(void) { ::Print(this.Header()); } //+------------------------------------------------------------------+
Simplesmente imprime no log o nome de string da série de tick.
Método para criar uma série de tick:
//+------------------------------------------------------------------+ //| Create the series list of tick data | //+------------------------------------------------------------------+ int CTickSeries::Create(const uint required=0) { //--- If the tick series is not used, inform of that and exit if(!this.m_available) { ::Print(DFUN,this.m_symbol,": ",CMessage::Text(MSG_TICKSERIES_TEXT_IS_NOT_USE)); return false; } //--- Declare the ticks[] array we are to receive historical data to, //--- clear the list of tick data objects and set the flag of sorting by time in milliseconds MqlTick ticks_array[]; this.m_list_ticks.Clear(); this.m_list_ticks.Sort(SORT_BY_TICK_TIME_MSC); ::ResetLastError(); int err=ERR_SUCCESS; //--- Calculate the day start time in milliseconds the ticks should be copied from MqlDateTime date_str={0}; datetime date=::iTime(m_symbol,PERIOD_D1,this.m_required); ::TimeToStruct(date,date_str); date_str.hour=date_str.min=date_str.sec=0; date=::StructToTime(date_str); long date_from=(long)date*1000; if(date_from<1) date_from=1; //--- Get historical data of the MqlTick structure to the tick[] array //--- from the calculated date to the current time and save the obtained number in m_amount. //--- If failed to get data, display the appropriate message and return zero this.m_amount=::CopyTicksRange(m_symbol,ticks_array,COPY_TICKS_ALL,date_from); if(this.m_amount<1) { err=::GetLastError(); ::Print(DFUN,CMessage::Text(MSG_TICKSERIES_ERR_GET_TICK_DATA),": ",CMessage::Text(err),CMessage::Retcode(err)); return 0; } //--- Historical data is received in the rates[] array //--- In the ticks[] array loop for(int i=0; i<(int)this.m_amount; i++) { //--- create a new object of tick data out of the current MqlTick structure data from the ticks[] array by the loop index ::ResetLastError(); CDataTick* tick=new CDataTick(this.m_symbol,ticks_array[i]); if(tick==NULL) { ::Print ( DFUN,CMessage::Text(MSG_TICKSERIES_FAILED_CREATE_TICK_DATA_OBJ)," ",this.Header()," ",::TimeMSCtoString(ticks_array[i].time_msc),". ", CMessage::Text(MSG_LIB_SYS_ERROR),": ",CMessage::Text(::GetLastError()) ); continue; } //--- If failed to add a new tick data object to the list, //--- display the appropriate message with the error description in the journal //--- and remove the newly created object if(!this.m_list_ticks.Add(tick)) { err=::GetLastError(); ::Print(DFUN,CMessage::Text(MSG_TICKSERIES_FAILED_ADD_TO_LIST)," ",tick.Header()," ", CMessage::Text(MSG_LIB_SYS_ERROR),": ",CMessage::Text(err),CMessage::Retcode(err)); delete tick; } } //--- Return the size of the created bar object list return this.m_list_ticks.Total(); } //+------------------------------------------------------------------+
O método é descrito em detalhes diretamente na listagem de código. Resumidamente:
calculamos a hora de início do dia a partir da qual precisamos copiar os ticks, solicitamos os ticks desde a data calculada para o array, e se os ticks forem copiados com sucesso, então, num loop para o array de ticks resultante, obtemos o próximo tick no formato da estrutura MqlTick, e com base nisso, criamos um novo objeto-tick, que, após uma criação bem-sucedida, colocamos na lista. No final do ciclo, retornamos o número de ticks colocados na lista de dados de tick.
Assim fica concluída a criação da lista de dados de tick.
Testando a criação de lista e aquisição de dados
Para teste, simplesmente, ao iniciar o programa, criamos uma lista de objetos-ticks para o símbolo e o dia atual. Na lista resultante, encontramos o tick com o maior preço Ask e o menor preço Bid e exibimos no log os dados dos objetos-tick encontrados. Para fazer isso, pegamos o Expert Advisor do artigo anterior e o salvamos na nova pasta \MQL5\Experts\TestDoEasy\Part60\ com o novo nome TestDoEasyPart60.mq5.
Como este é apenas um teste da lista de dados de tick, e não há acesso a ele diretamente da biblioteca, então ao arquivo do EA anexamos a classe do objeto-lista de dados de tick :
//| TestDoEasyPart60.mq5 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://mql5.com/en/users/artmedia70 | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://mql5.com/en/users/artmedia70" #property version "1.00" //--- includes #include <DoEasy\Engine.mqh> #include <DoEasy\Objects\Ticks\TickSeries.mqh> //--- enums
No escopo de variáveis globais do programa declaramos um objeto-lista de dados de tick:
//--- global variables CEngine engine; SDataButt butt_data[TOTAL_BUTT]; string prefix; double lot; double withdrawal=(InpWithdrawal<0.1 ? 0.1 : InpWithdrawal); ushort magic_number; uint stoploss; uint takeprofit; uint distance_pending; uint distance_stoplimit; uint distance_pending_request; uint bars_delay_pending_request; uint slippage; bool trailing_on; bool pressed_pending_buy; bool pressed_pending_buy_limit; bool pressed_pending_buy_stop; bool pressed_pending_buy_stoplimit; bool pressed_pending_close_buy; bool pressed_pending_close_buy2; bool pressed_pending_close_buy_by_sell; bool pressed_pending_sell; bool pressed_pending_sell_limit; bool pressed_pending_sell_stop; bool pressed_pending_sell_stoplimit; bool pressed_pending_close_sell; bool pressed_pending_close_sell2; bool pressed_pending_close_sell_by_buy; bool pressed_pending_delete_all; bool pressed_pending_close_all; bool pressed_pending_sl; bool pressed_pending_tp; double trailing_stop; double trailing_step; uint trailing_start; uint stoploss_to_modify; uint takeprofit_to_modify; int used_symbols_mode; string array_used_symbols[]; string array_used_periods[]; bool testing; uchar group1; uchar group2; double g_point; int g_digits; //--- "New tick" object CNewTickObj check_tick; //--- Object of the current symbol tick series data CTickSeries tick_series; //+------------------------------------------------------------------+
No OnTick() removemos o bloco de código para trabalhar com objetos de dados de tick - isso ficou do último artigo, e aqui não criaremos nenhum objeto:
//--- Create a temporary list for storing “Tick data” objects,
//--- a variable for obtaining tick data and
//--- a variable for calculating incoming ticks
static int tick_count=0;
CArrayObj list;
MqlTick tick_struct;
//--- Check a new tick on the current symbol
if(check_tick.IsNewTick())
{
//--- If failed to get the price - exit
if(!SymbolInfoTick(Symbol(),tick_struct))
return;
//--- Create a new tick data object
CDataTick *tick_obj=new CDataTick(Symbol(),tick_struct);
if(tick_obj==NULL)
return;
//--- Increase tick counter (simply to display on the screen, no other purpose is provided)
tick_count++;
//--- Limit the number of ticks in the counting as one hundred thousand (again, no purpose is provided)
if(tick_count>100000) tick_count=1;
//--- In the comment on the chart display the tick number and its short description
Comment("--- #",IntegerToString(tick_count,5,'0'),": ",tick_obj.Header());
//--- If this is the first tick (which follows the first launch of EA) display its full description in the journal
if(tick_count==1)
tick_obj.Print();
//--- Remove if failed to put the created tick data object in the list
if(!list.Add(tick_obj))
delete tick_obj;
}
Assim, o manipulador OnTick() fica desse modo:
//+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- Handle the NewTick event in the library engine.OnTick(rates_data); //--- If working in the tester if(MQLInfoInteger(MQL_TESTER)) { engine.OnTimer(rates_data); // Working in the timer PressButtonsControl(); // Button pressing control engine.EventsHandling(); // Working with events } //--- If the trailing flag is set if(trailing_on) { TrailingPositions(); // Trailing positions TrailingOrders(); // Trailing pending orders } } //+------------------------------------------------------------------+
Criamos a lista no manipulador OnInit() dentro da função de inicialização da biblioteca DoEasy, no bloco de código:
//+------------------------------------------------------------------+ //| Initializing DoEasy library | //+------------------------------------------------------------------+ void OnInitDoEasy() { //--- Check if working with the full list is selected used_symbols_mode=InpModeUsedSymbols; if((ENUM_SYMBOLS_MODE)used_symbols_mode==SYMBOLS_MODE_ALL) { int total=SymbolsTotal(false); string ru_n="\nКоличество символов на сервере "+(string)total+".\nМаксимальное количество: "+(string)SYMBOLS_COMMON_TOTAL+" символов."; string en_n="\nNumber of symbols on server "+(string)total+".\nMaximum number: "+(string)SYMBOLS_COMMON_TOTAL+" symbols."; string caption=TextByLanguage("Внимание!","Attention!"); string ru="Выбран режим работы с полным списком.\nВ этом режиме первичная подготовка списков коллекций символов и таймсерий может занять длительное время."+ru_n+"\nПродолжить?\n\"Нет\" - работа с текущим символом \""+Symbol()+"\""; string en="Full list mode selected.\nIn this mode, the initial preparation of lists of symbol collections and timeseries can take a long time."+en_n+"\nContinue?\n\"No\" - working with the current symbol \""+Symbol()+"\""; string message=TextByLanguage(ru,en); int flags=(MB_YESNO | MB_ICONWARNING | MB_DEFBUTTON2); int mb_res=MessageBox(message,caption,flags); switch(mb_res) { case IDNO : used_symbols_mode=SYMBOLS_MODE_CURRENT; break; default: break; } } //--- Set the counter start point to measure the approximate library initialization time ulong begin=GetTickCount(); Print(TextByLanguage("--- Инициализация библиотеки \"DoEasy\" ---","--- Initializing the \"DoEasy\" library ---")); //--- Fill in the array of used symbols CreateUsedSymbolsArray((ENUM_SYMBOLS_MODE)used_symbols_mode,InpUsedSymbols,array_used_symbols); //--- Set the type of the used symbol list in the symbol collection and fill in the list of symbol timeseries engine.SetUsedSymbols(array_used_symbols); //--- Displaying the selected mode of working with the symbol object collection in the journal string num= ( used_symbols_mode==SYMBOLS_MODE_CURRENT ? ": \""+Symbol()+"\"" : TextByLanguage(". Количество используемых символов: ",". The number of symbols used: ")+(string)engine.GetSymbolsCollectionTotal() ); Print(engine.ModeSymbolsListDescription(),num); //--- Implement displaying the list of used symbols only for MQL5 - MQL4 has no ArrayPrint() function #ifdef __MQL5__ if(InpModeUsedSymbols!=SYMBOLS_MODE_CURRENT) { string array_symbols[]; CArrayObj* list_symbols=engine.GetListAllUsedSymbols(); for(int i=0;i<list_symbols.Total();i++) { CSymbol *symbol=list_symbols.At(i); if(symbol==NULL) continue; ArrayResize(array_symbols,ArraySize(array_symbols)+1,SYMBOLS_COMMON_TOTAL); array_symbols[ArraySize(array_symbols)-1]=symbol.Name(); } ArrayPrint(array_symbols); } #endif //--- Set used timeframes CreateUsedTimeframesArray(InpModeUsedTFs,InpUsedTFs,array_used_periods); //--- Display the selected mode of working with the timeseries object collection string mode= ( InpModeUsedTFs==TIMEFRAMES_MODE_CURRENT ? TextByLanguage("Работа только с текущим таймфреймом: ","Work only with the current Period: ")+TimeframeDescription((ENUM_TIMEFRAMES)Period()) : InpModeUsedTFs==TIMEFRAMES_MODE_LIST ? TextByLanguage("Работа с заданным списком таймфреймов:","Work with a predefined list of Periods:") : TextByLanguage("Работа с полным списком таймфреймов:","Work with the full list of all Periods:") ); Print(mode); //--- Implement displaying the list of used timeframes only for MQL5 - MQL4 has no ArrayPrint() function #ifdef __MQL5__ if(InpModeUsedTFs!=TIMEFRAMES_MODE_CURRENT) ArrayPrint(array_used_periods); #endif //--- Create timeseries of all used symbols engine.SeriesCreateAll(array_used_periods); //--- Check created timeseries - display descriptions of all created timeseries in the journal //--- (true - only created ones, false - created and declared ones) engine.GetTimeSeriesCollection().PrintShort(false); // Short descriptions //engine.GetTimeSeriesCollection().Print(true); // Full descriptions //--- Code block for checking the tick list creation and working with it Print(""); //--- Since the tick series object is created with the default constructor, //--- set a symbol, usage flag and the number of days (the default is 1) to copy the ticks //--- Create the tick series and printed data in the journal tick_series.SetSymbol(Symbol()); tick_series.SetAvailable(true); tick_series.SetRequiredUsedDays(); tick_series.Create(); tick_series.Print(); Print(""); //--- Get and display in the journal the data of an object with the highest Ask price in the daily price range int index_max=CSelect::FindTickDataMax(tick_series.GetList(),TICK_PROP_ASK); CDataTick *tick_max=tick_series.GetList().At(index_max); if(tick_max!=NULL) tick_max.Print(); //--- Get and display in the journal the data of an object with the lowest Bid price in the daily price range int index_min=CSelect::FindTickDataMin(tick_series.GetList(),TICK_PROP_BID); CDataTick *tick_min=tick_series.GetList().At(index_min); if(tick_min!=NULL) tick_min.Print(); //--- Create resource text files engine.CreateFile(FILE_TYPE_WAV,"sound_array_coin_01",TextByLanguage("Звук упавшей монетки 1","Falling coin 1"),sound_array_coin_01); engine.CreateFile(FILE_TYPE_WAV,"sound_array_coin_02",TextByLanguage("Звук упавших монеток","Falling coins"),sound_array_coin_02); engine.CreateFile(FILE_TYPE_WAV,"sound_array_coin_03",TextByLanguage("Звук монеток","Coins"),sound_array_coin_03); engine.CreateFile(FILE_TYPE_WAV,"sound_array_coin_04",TextByLanguage("Звук упавшей монетки 2","Falling coin 2"),sound_array_coin_04); engine.CreateFile(FILE_TYPE_WAV,"sound_array_click_01",TextByLanguage("Звук щелчка по кнопке 1","Button click 1"),sound_array_click_01); engine.CreateFile(FILE_TYPE_WAV,"sound_array_click_02",TextByLanguage("Звук щелчка по кнопке 2","Button click 2"),sound_array_click_02); engine.CreateFile(FILE_TYPE_WAV,"sound_array_click_03",TextByLanguage("Звук щелчка по кнопке 3","Button click 3"),sound_array_click_03); engine.CreateFile(FILE_TYPE_WAV,"sound_array_cash_machine_01",TextByLanguage("Звук кассового аппарата","Cash machine"),sound_array_cash_machine_01); engine.CreateFile(FILE_TYPE_BMP,"img_array_spot_green",TextByLanguage("Изображение \"Зелёный светодиод\"","Image \"Green Spot lamp\""),img_array_spot_green); engine.CreateFile(FILE_TYPE_BMP,"img_array_spot_red",TextByLanguage("Изображение \"Красный светодиод\"","Image \"Red Spot lamp\""),img_array_spot_red); //--- Pass all existing collections to the main library class engine.CollectionOnInit(); //--- Set the default magic number for all used symbols engine.TradingSetMagic(engine.SetCompositeMagicNumber(magic_number)); //--- Set synchronous passing of orders for all used symbols engine.TradingSetAsyncMode(false); //--- Set the number of trading attempts in case of an error engine.TradingSetTotalTry(InpTotalAttempts); //--- Set correct order expiration and filling types to all trading objects engine.TradingSetCorrectTypeExpiration(); engine.TradingSetCorrectTypeFilling(); //--- Set standard sounds for trading objects of all used symbols engine.SetSoundsStandart(); //--- Set the general flag of using sounds engine.SetUseSounds(InpUseSounds); //--- Set the spread multiplier for symbol trading objects in the symbol collection engine.SetSpreadMultiplier(InpSpreadMultiplier); //--- Set controlled values for symbols //--- Get the list of all collection symbols CArrayObj *list=engine.GetListAllUsedSymbols(); if(list!=NULL && list.Total()!=0) { //--- In a loop by the list, set the necessary values for tracked symbol properties //--- By default, the LONG_MAX value is set to all properties, which means "Do not track this property" //--- It can be enabled or disabled (by setting the value less than LONG_MAX or vice versa - set the LONG_MAX value) at any time and anywhere in the program /* for(int i=0;i<list.Total();i++) { CSymbol* symbol=list.At(i); if(symbol==NULL) continue; //--- Set control of the symbol price increase by 100 points symbol.SetControlBidInc(100000*symbol.Point()); //--- Set control of the symbol price decrease by 100 points symbol.SetControlBidDec(100000*symbol.Point()); //--- Set control of the symbol spread increase by 40 points symbol.SetControlSpreadInc(400); //--- Set control of the symbol spread decrease by 40 points symbol.SetControlSpreadDec(400); //--- Set control of the current spread by the value of 40 points symbol.SetControlSpreadLevel(400); } */ } //--- Set controlled values for the current account CAccount* account=engine.GetAccountCurrent(); if(account!=NULL) { //--- Set control of the profit increase to 10 account.SetControlledValueINC(ACCOUNT_PROP_PROFIT,10.0); //--- Set control of the funds increase to 15 account.SetControlledValueINC(ACCOUNT_PROP_EQUITY,15.0); //--- Set profit control level to 20 account.SetControlledValueLEVEL(ACCOUNT_PROP_PROFIT,20.0); } //--- Get the end of the library initialization time counting and display it in the journal ulong end=GetTickCount(); Print(TextByLanguage("Время инициализации библиотеки: ","Library initialization time: "),TimeMSCtoString(end-begin,TIME_MINUTES|TIME_SECONDS)); } //+------------------------------------------------------------------+
O bloco de código para criar uma série de ticks do símbolo atual para o dia atual e pesquisa de dois objetos tick para enviar seus dados para o log é comentado em detalhes e, eu acho que não surgirão perguntas ao estudá-lo. Em qualquer caso, todas as perguntas podem ser feitas na discussão do artigo.
Esta função é chamada a partir do manipulador OnInit(), por isso, a lista será criada uma vez quando o programa iniciar. E imediatamente nele serão encontrados dois objetos de dados de tick - com Ask máximo e o Bid mínimo para o dia atual. A saída dos dados levará algum tempo - se ainda não houver dados de tick localmente, o upload será ativado.
Vamos compilar o Expert Advisor e iniciá-lo no gráfico de qualquer símbolo, tendo previamente especificado nas configurações o uso do símbolo atual e o timeframe atual. Quando o EA for inicializado, ele exibirá dados sobre os parâmetros do EA criado por TimeSeries e, depois de um tempo, os dados sobre a série de ticks criada. Abaixo serão mostrados dados sobre dois ticks encontrados - com Ask máximo e o Bid mínimo para o dia atual.
Account 8550475: Artyom Trishkin (MetaQuotes Software Corp.) 10426.13 USD, 1:100, Hedge, MetaTrader 5 demo --- Initializing "DoEasy" library --- Working with the current symbol only: "EURUSD" Working with the current timeframe only: H4 EURUSD symbol timeseries: - Timeseries "EURUSD" H4: Requested: 1000, Actual: 1000, Created: 1000, On the server: 6336 Tick series "EURUSD": Requested number of days: 1, Historical data created: 276143 ============= Beginning of parameter list (Tick "EURUSD" 2021.01.06 14:25:32.156) ============= Last price update time in milliseconds: 2021.01.06 14:25:32.156 Last price update time: 2021.01.06 14:25:32 Volume for the current Last price: 0 Flags: 134 Changed data on the tick: - Ask price change - Bid price change ------ Bid price: 1.23494 Ask price: 1.23494 Last price: 0.00000 Volume for the current Last price with greater accuracy: 0.00 Spread: 0.00000 ------ Symbol: "EURUSD" ============= End of parameter list (Tick "EURUSD" 2021.01.06 14:25:32.156) ============= ============= Beginning of parameter list (Tick "EURUSD" 2021.01.07 12:51:40.632) ============= Last price update time in milliseconds: 2021.01.07 12:51:40.632 Last price update time: 2021.01.07 12:51:40 Volume for the current Last price: 0 Flags: 134 Changed data on the tick: - Ask price change - Bid price change ------ Bid price: 1.22452 Ask price: 1.22454 Last price: 0.00000 Volume for the current Last price with greater accuracy: 0.00 Spread: 0.00002 ------ Symbol: "EURUSD" ============= End of parameter list (Tick "EURUSD" 2021.01.07 12:51:40.632) ============= Library initialization time: 00:00:12.828
A inicialização levou 12,8 segundos - tempo de carga dos dados históricos de tick.
O que vem agora?
No próximo artigo, criaremos uma classe-coleção de dados de tick cobrindo todos os símbolos usados no programa e implementaremos a atualização em tempo real de todas as listas criadas.
Todos os arquivos da versão atual da biblioteca e o arquivo do EA de teste para MQL5 estão anexados abaixo. Você pode baixá-los e testar tudo sozinho.
Quero ressaltar que as classes de dados tick estão em desenvolvimento e, portanto, seu uso em seus programas neste estágio é altamente indesejável.
Se você tiver perguntas, comentários e sugestões, poderá expressá-los nos comentários do artigo.
Artigos desta série:
Trabalhando com séries temporais na biblioteca DoEasy (Parte 35): objeto "Barra" e lista-série temporal do símbolo
Trabalhando com séries temporais na biblioteca DoEasy (Parte 36): objeto das séries temporais de todos os períodos usados do símbolo
Trabalhando com séries temporais na biblioteca DoEasy (Parte 37): coleção de séries temporais - banco de dados de séries temporais para símbolos e períodos
Trabalhando com séries temporais na biblioteca DoEasy (Parte 38): coleção de séries temporais - atualização em tempo real e acesso aos dados do programa
Trabalhando com séries temporais na biblioteca DoEasy (Parte 39): indicadores com base na biblioteca - preparação de dados e eventos das séries temporais
Trabalhando com séries temporais na biblioteca DoEasy (Parte 40): indicadores com base na biblioteca - atualização de dados em tempo real
Trabalhando com séries temporais na biblioteca DoEasy (Parte 41): exemplo de indicador multissímbolo multiperíodo
Trabalhando com séries temporais na biblioteca DoEasy (Parte 42): classe de um objeto de buffer abstrato de indicador
Trabalhando com séries temporais na biblioteca DoEasy (Parte 43): classes de objetos de buffers de indicador
Trabalhando com séries temporais na biblioteca DoEasy (Parte 44): classe-coleção de objetos de buffers de indicador
Trabalhando com séries temporais na biblioteca DoEasy (Parte 45): buffers de indicador multiperíodo
Trabalhando com séries temporais na biblioteca DoEasy (Parte 46): buffers de indicador multiperíodos multissímbolos
Trabalhando com séries temporais na biblioteca DoEasy (Parte 47): indicadores padrão multiperíodos multissímbolos
Trabalhando com séries temporais na biblioteca DoEasy (Parte 48): indicadores multissímbolos multiperíodos num buffer de uma subjanela
Trabalhando com séries temporais na biblioteca DoEasy (Parte 49): indicadores padrão multiperíodos multissímbolos multibuffer
Trabalhando com séries temporais na biblioteca DoEasy (Parte 50): indicadores padrão multiperíodos multissímbolos com deslocamento
Trabalhando com séries temporais na biblioteca DoEasy (Parte 51): indicadores padrão multiperíodos multissímbolos compostos
Trabalhando com séries temporais na biblioteca DoEasy (Parte 52): natureza multiplataforma de indicadores padrão multiperíodos multissímbolos de buffer único
Trabalhando com séries temporais na biblioteca DoEasy (Parte 53): classe do indicador base abstrato
Trabalhando com séries temporais na biblioteca DoEasy (Parte 54): classes herdeiras do indicador base abstrato
Trabalhando com séries temporais na biblioteca DoEasy (Parte 55): classe-coleção de indicadores
Trabalhando com séries temporais na biblioteca DoEasy (Parte 56): objeto de indicador personalizado, obtenção de dados a partir de objetos-indicadores numa coleção
Trabalhando com séries temporais na biblioteca DoEasy (Parte 57): objeto de dados do buffer do indicador
Trabalhando com séries temporais na biblioteca DoEasy (Parte 58): séries temporais de dados de buffers de indicadores
Trabalhando com séries temporais na biblioteca DoEasy (Parte 59): objeto para armazenar dados de um tick
Traduzido do russo pela MetaQuotes Ltd.
Artigo original: https://www.mql5.com/ru/articles/8912





- Aplicativos de negociação gratuitos
- 8 000+ sinais para cópia
- Notícias econômicas para análise dos mercados financeiros
Você concorda com a política do site e com os termos de uso