Could you show me an example?
I know about pointers, they are storing addresses. But how do I reference to the next one?
class order {
private:
int ticket;
public:
void enter_ticket();
} ;
They are referenced with the At() method.
Look at the documentation example: https://www.mql5.com/en/docs/standardlibrary/datastructures/carrayobj/carrayobjat
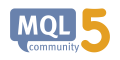
- www.mql5.com
?
class order { private: int ticket; public: void enter_ticket(int tick){ticket=tick}; } ; ... order first; first.enter_ticket(5468);
Modified your class version. I have not tested so there may be errors, but it's more or less like the ff:
int max_order = 10; class Order : public CObject { private: int ticket; datetime open_time; int type; double lots; string symbol; double open_price; double take_profit; int magic_number; double ATR; bool losing_order; double next_Win_Entry; double next_Lose_Entry; double next_LotSize; public: void Order(void){}; void set_ticket (int value) {ticket=value;}; int read_ticket (void) {return ticket;}; }; CArrayObj MyOrders; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { Order *order1 = new Order(); order1.set_ticket(1); Order *order2 = new Order(); order2.set_ticket(2); Order *order3 = new Order(); order3.set_ticket(3); MyOrders.Add(order1); //add first order object MyOrders.Add(order2); //add second order object MyOrders.Add(order3); //add third order object Print(MyOrders.Total()); //returns total number of orders = 3 //iterate over orders within MyOrders for (int i=0;i<MyOrders.Total();i++) { Order *item = MyOrders.At(i); //get order object at index i Print(item.read_ticket()); //print ticket of order object at index i } return(INIT_SUCCEEDED); }
CArrayObj already has a built-in array in its private class members. The array is of type CObject, so the objects that you would add to this CArrayObj should also inherit from CObject (hence
class Order : public CObject
). There is no need to declare an additional array.
For this function:
return_ticket_of_largest_open_price()
CArrayObj won't be able to provide that, but you can declare a new Orders object that inherits from CArrayObj, use that object instead of CArrayObj, and declare the member function there.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I am new to OOP, and I have read some articles on it, but I do not know how to implement it.
an order class has ticket, open_time.
MyOrders have 10 tickets in array
I would like to call them by
MyOrders[0].ticket = 123;
how do I do it?