Try this code
//+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ bool Triangle(string obj_name,int x0,int y0,int x1,int y1,int x2,int y2,color c) { uint data[]; string rcname="::"+obj_name; int t,w,h; //--- sort by Y if(y0>y1) { t=y1; y1=y0; y0=t; t=x1; x1=x0; x0=t; } if(y0>y2) { t=y0; y0=y2; y2=t; t=x0; x0=x2; x2=t; } if(y1>y2) { t=y1; y1=y2; y2=t; t=x1; x1=x2; x2=t; } //--- min/max by X int minx=MathMin(x0,MathMin(x1,x2)); int maxx=MathMax(x0,MathMax(x1,x2)); //--- invisible if(y2<0 || maxx<0) { if(ArrayResize(data,1)<0) return(false); data[0]=0; w =1; h =1; } else { w=maxx+1; h=y2+1; //--- if(ArrayResize(data,w*h)<0) return(false); ArrayInitialize(data,0); //--- double k1,k2; //--- if((t=y0-y1)!=0) k1=(x0-x1)/(double)t; if((t=y0-y2)!=0) k2=(x0-x2)/(double)t; double xd1=x0; double xd2=x0; //--- for(int i=y0,xx1,xx2;i<=y2;i++) { if(i==y1) { if((t=y1-y2)!=0) k1=(x1-x2)/(double)t; xd1=x1; } //--- xx1=(int)xd1; xd1+=k1; xx2=(int)xd2; xd2+=k2; //--- if(i<0) continue; //--- if(xx1>xx2) { t=xx1; xx1=xx2; xx2=t; } //--- if(xx2<0 || xx1>=w) continue; //--- if(xx1<0) xx1=0; if(xx2>=w) xx2=w-1; for(int j=xx1;j<=xx2;j++) data[i*w+j]=0xFF000000|((c&0xFF)<<16)|(c&0xFF00)|((c>>16)&0xFF); } } //--- if(!ResourceCreate(rcname,data,w,h,0,0,0,COLOR_FORMAT_ARGB_RAW)) return(false); ObjectSetString(0,obj_name,OBJPROP_BMPFILE,rcname); return(true); } //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { ObjectCreate(0,"tri",OBJ_BITMAP_LABEL,0,0,0); ObjectSetInteger(0,"tri",OBJPROP_XDISTANCE,100); ObjectSetInteger(0,"tri",OBJPROP_YDISTANCE,100); ObjectSetInteger(0,"tri",OBJPROP_SELECTABLE,1); ObjectSetInteger(0,"tri",OBJPROP_SELECTED,1); //--- while(!IsStopped()) { Triangle("tri",rand()%100,rand()%100,rand()%200,rand()%200,rand()%200,rand()%200,clrRed); ChartRedraw(); Sleep(100); } //--- ObjectDelete(0,"tri"); }
Try this code
Hello,
this code is very helpfull. What's about objects placed on price/time basement.
I try to code a zigzag with a cumulate volume for each swing. But all my volumes are placed in the upper left corner of the current xy-coordinates.
Do you have any ideas, to change the xy-base to price/time to place my text object over the specified bars.
https://www.mql5.com/en/forum/6388#comment_170999
Greetings
Mike
Whoa !!! Thank you very much - I'm so exited here :D. My apology I just read your post and I didn't check that function. I update to 655 and read Service Desk and I thought those function are for next build above 655. So I see there's no documentation yet, but thats OK :D
[Edit : Oops, Click here ChartTimePriceToXY and ChartXYToTimePrice .]
This is good, now we know exactly where - at least for example - mouse's cursor location in bar time and price coordinate, so we don't click pixels anymore.
Excellently awesome !!!. Thank you very much !!!
Whoa !!! Thank you very much - I'm so exited here :D. My apology I just read your post and I didn't check that function. I update to 655 and read Service Desk and I thought those function are for next build above 655. So I see there's no documentation yet, but thats OK :D
Documentation is always available online and you can download it manually - https://www.mql5.com/en/docs/chart_operations
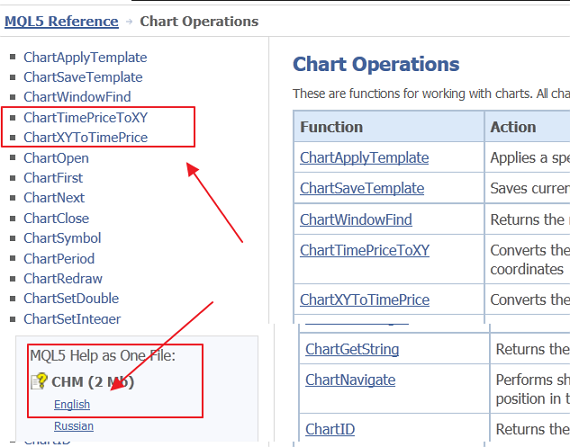
Documentation is always available online and you can download it manually - https://www.mql5.com/en/docs/chart_operations

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I'm desperately trying to draw a triangle based on x;y coordinates, not on price/time values.
The goal is to create the triangle on the indicator's window, not on the chart window.
I tried the bmp lib on codebase, but the refresh rate is too slow to be usable.
Thanks a lot!