
Otherwise look at this code to help you
Free download of the 'MACD code for beginners by William210' indicator by 'William210' for MetaTrader 5 in the MQL5 Code Base, 2023.09.02
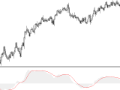
- www.mql5.com
You need to define plots.
#property indicator_separate_window #property indicator_buffers 5 #property indicator_plots 2 //--- plot MACD #property indicator_label1 "MACD" #property indicator_type1 DRAW_HISTOGRAM #property indicator_color1 clrRed #property indicator_style1 STYLE_SOLID #property indicator_width1 1 //--- plot Signal #property indicator_label2 "Signal" #property indicator_type2 DRAW_LINE #property indicator_color2 clrRed #property indicator_style2 STYLE_SOLID #property indicator_width2 1
The signal calculation is wrong: EMA of the MACD line is called the signal line.
(You assume EMA of close price is the signal line)You will learn through this code
https://www.mql5.com/en/code/14669
If you want to figure things out yourself, that's ok as a challenge, but reason why I'm linking you this code is because it is programmed well with optimizations.
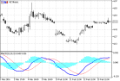
- www.mql5.com
thanks for all help. i change my code to this and now is worked. also I checked the macd classic with the trading view and it seems all calculate and show currently.
but it is very slow and 15 - 30 second after drag and drop on the chart, indicator line is show. how can I optimize my code? please help.
my entire code is:
//+------------------------------------------------------------------+ //| MACD Classic 2024.mq5 | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #include <MovingAverages.mqh> //--- input parameters input int FastEMA = 12; input int SlowEMA = 26; input int Signal = 9; //--- indicator buffers double MACDBuffer[]; double SignalBuffer[]; double HistogramBuffer[]; double fastEMA_Array[]; double slowEMA_Array[]; int Iwindows =0; int handleFastEMA; int handle1SlowEMA; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { Iwindows = ChartWindowFind(0,"MACD Classic 2024"); //--- indicator buffers mapping SetIndexBuffer(0,MACDBuffer,INDICATOR_DATA); SetIndexBuffer(1,SignalBuffer,INDICATOR_DATA); SetIndexBuffer(2,HistogramBuffer,INDICATOR_DATA); IndicatorSetString(INDICATOR_SHORTNAME,"MACD Classic 2024"); ArraySetAsSeries(MACDBuffer,true); ArraySetAsSeries(SignalBuffer,true); ArraySetAsSeries(HistogramBuffer,true); ArraySetAsSeries(fastEMA_Array,true); ArraySetAsSeries(slowEMA_Array,true); handleFastEMA = iMA(NULL, PERIOD_CURRENT, FastEMA, 0, MODE_EMA,PRICE_CLOSE); handle1SlowEMA = iMA(NULL, PERIOD_CURRENT, SlowEMA, 0, MODE_EMA, PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { //--- int bars = rates_total-1; if(prev_calculated>0) { bars=rates_total-(prev_calculated-1); } CopyBuffer(handleFastEMA, MAIN_LINE, 0, bars, fastEMA_Array); CopyBuffer(handle1SlowEMA, MAIN_LINE, 0, bars, slowEMA_Array); for(int i=bars; i>=0; i--) { MACDBuffer[i] = fastEMA_Array[i-1] - slowEMA_Array[i-1]; ExponentialMAOnBuffer(rates_total,prev_calculated,i,Signal,MACDBuffer,SignalBuffer); HistogramBuffer[i] = MACDBuffer[i] - SignalBuffer[i]; } //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+
thanks for all help. i change my code to this and now is worked. also I checked the macd classic with the trading view and it seems all calculate and show currently.
but it is very slow and 15 - 30 second after drag and drop on the chart, indicator line is show. how can I optimize my code? please help.
my entire code is:
I recommend you to compare your code with the macd that is in the Examples menu of mt5 to get yours working.
that's not your entire code, I don't know how you can say this because you're missing all the properties at the top of the script
Your for loop will cause an exception, you can't index [i-1] with i>=0
so make it i>0, and use the
ExponentialMAOnBuffer
outside of the for loop
for(int i=bars; i>0; i--) { MACDBuffer[i] = fastEMA_Array[i-1] - slowEMA_Array[i-1]; } ExponentialMAOnBuffer(rates_total,prev_calculated,0,Signal,MACDBuffer,SignalBuffer);
full script:
//+------------------------------------------------------------------+ //| MACD Classic 2024.mq5 | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #include <MovingAverages.mqh> //--- input parameters input int FastEMA = 12; input int SlowEMA = 26; input int Signal = 9; #property indicator_separate_window #property indicator_buffers 3 #property indicator_plots 3 // Plot MACD #property indicator_label1 "MACD" #property indicator_type1 DRAW_LINE #property indicator_color1 clrPurple #property indicator_style1 STYLE_SOLID #property indicator_width1 2 // Plot Signal #property indicator_label2 "Signal" #property indicator_type2 DRAW_LINE #property indicator_color2 clrRed #property indicator_style2 STYLE_SOLID #property indicator_width2 1 // Plot Histogram #property indicator_label3 "Histogram" #property indicator_type3 DRAW_HISTOGRAM #property indicator_color3 clrGray #property indicator_style3 STYLE_SOLID #property indicator_width3 2 //--- indicator buffers double MACDBuffer[]; double SignalBuffer[]; double HistogramBuffer[]; double fastEMA_Array[]; double slowEMA_Array[]; int Iwindows =0; int handleFastEMA; int handle1SlowEMA; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { Iwindows = ChartWindowFind(1,"MACD Classic 2044"); //--- indicator buffers mapping SetIndexBuffer(0,MACDBuffer,INDICATOR_DATA); SetIndexBuffer(1,SignalBuffer,INDICATOR_DATA); SetIndexBuffer(2,HistogramBuffer,INDICATOR_DATA); IndicatorSetString(INDICATOR_SHORTNAME,"MACD Classic 2024"); ArraySetAsSeries(MACDBuffer,true); ArraySetAsSeries(SignalBuffer,true); ArraySetAsSeries(HistogramBuffer,true); ArraySetAsSeries(fastEMA_Array,true); ArraySetAsSeries(slowEMA_Array,true); handleFastEMA = iMA(NULL, PERIOD_CURRENT, FastEMA, 0, MODE_EMA,PRICE_CLOSE); handle1SlowEMA = iMA(NULL, PERIOD_CURRENT, SlowEMA, 0, MODE_EMA, PRICE_CLOSE); //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate(const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[]) { int bars = rates_total-1; if(prev_calculated<=0) bars = rates_total-1; if(prev_calculated>0) { bars=rates_total-(prev_calculated - (rates_total==prev_calculated)); } CopyBuffer(handleFastEMA, 0, 0, bars, fastEMA_Array); CopyBuffer(handle1SlowEMA, 0, 0, bars, slowEMA_Array); for(int i=bars; i>0; i--) { MACDBuffer[i] = fastEMA_Array[i-1] - slowEMA_Array[i-1]; } ExponentialMAOnBuffer(rates_total,prev_calculated,0,Signal,MACDBuffer,SignalBuffer); for(int i=bars; i>=0; i--) { HistogramBuffer[i] = MACDBuffer[i] - SignalBuffer[i]; } //--- return value of prev_calculated for next call return(rates_total); }

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hi I want create MACD Classic in MQL 5. in this macd the signal line also should the create with Mode_EMA. (all of other indicator i find the Signal line in sma mode and can not be changed).
My entire code is like below. I do not have the syntax error, But the indicator not run. please help.