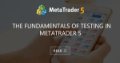
- www.mql5.com
- PROBLEM WITH STRATEGY TESTER MT4
- EA - MQL5 - managing different position opening on different TF
- How to check if a position is open?
So I'm pretty new to MQL5 and Metatrader in general but I wanted to build my own EA with simple moving average and stochastic crossover. I feel as though I have the code right but when run in the strategy tester it places no orders, even when the conditions to do so are seemingly met in the data. Is there anything super obvious I'm missing? Thanks in advance!
// Calculate indicators double fast_ma = iMA(NULL, 0, Fast_MA_Period, 0, MODE_EMA, PRICE_CLOSE); double medium_ma = iMA(NULL, 0, Medium_MA_Period, 0, MODE_EMA, PRICE_CLOSE); double slow_ma = iMA(NULL, 0, Slow_MA_Period, 0, MODE_EMA, PRICE_CLOSE); double stochastic_main = iStochastic(NULL, 0, Stochastic_Period, 3, Stochastic_Slowing, Stochastic_Method, STO_LOWHIGH); double stochastic_signal = iStochastic(NULL, 0, Stochastic_Period, 3, Stochastic_Slowing, Stochastic_Method, STO_CLOSECLOSE);
These functions return the indicator handle, not the buffer values.
https://www.mql5.com/en/docs/indicators/ima
https://www.mql5.com/en/docs/indicators/istochastic
Most likely, you generated this code using artificial intelligence. Artificial intelligence harms MQL learning because it generates complete nonsense. Don't use artificial intelligence when learning MQL
These functions return the indicator handle, not the buffer values.
https://www.mql5.com/en/docs/indicators/ima
https://www.mql5.com/en/docs/indicators/istochastic
Most likely, you generated this code using artificial intelligence. Artificial intelligence harms MQL learning because it generates complete nonsense. Don't use artificial intelligence when learning MQL
That being said I did update my code using CopyBuffer but still no orders being placed.
#include <Trade\Trade.mqh> input int Fast_MA_Period = 5; input int Medium_MA_Period = 8; input int Slow_MA_Period = 13; input int Stochastic_Period = 5; input int Stochastic_Slowing = 3; input ENUM_MA_METHOD Stochastic_Method = MODE_SMA; // Stochastic Method input double Overbought_Level = 80; input double Oversold_Level = 20; input double Stop_Loss_Multiplier = 1.5; // Multiplier for dynamic stop loss input double Take_Profit_Multiplier = 2.0; // Multiplier for dynamic take profit input double Volume = 0.1; // Trading volume double Ask, Bid; int signal = 0; // 0 - no signal, 1 - buy signal, -1 - sell signal double stopLossLevel, takeProfitLevel; int fast_ma_handle, medium_ma_handle, slow_ma_handle; int stochastic_main_handle, stochastic_signal_handle; void OnInit() { fast_ma_handle = iMA(NULL, 0, Fast_MA_Period, 0, MODE_EMA, PRICE_CLOSE); medium_ma_handle = iMA(NULL, 0, Medium_MA_Period, 0, MODE_EMA, PRICE_CLOSE); slow_ma_handle = iMA(NULL, 0, Slow_MA_Period, 0, MODE_EMA, PRICE_CLOSE); stochastic_main_handle = iStochastic(NULL, 0, Stochastic_Period, 3, Stochastic_Slowing, Stochastic_Method, STO_LOWHIGH); stochastic_signal_handle = iStochastic(NULL, 0, Stochastic_Period, 3, Stochastic_Slowing, Stochastic_Method, STO_CLOSECLOSE); } void OnTick() { Ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); Bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); double fast_ma_value[], medium_ma_value[], slow_ma_value[]; double stochastic_main_value[], stochastic_signal_value[]; ArraySetAsSeries(fast_ma_value, true); ArraySetAsSeries(medium_ma_value, true); ArraySetAsSeries(slow_ma_value, true); ArraySetAsSeries(stochastic_main_value, true); ArraySetAsSeries(stochastic_signal_value, true); CopyBuffer(fast_ma_handle, 0, 0, ArraySize(fast_ma_value), fast_ma_value); CopyBuffer(medium_ma_handle, 0, 0, ArraySize(medium_ma_value), medium_ma_value); CopyBuffer(slow_ma_handle, 0, 0, ArraySize(slow_ma_value), slow_ma_value); CopyBuffer(stochastic_main_handle, MAIN_LINE, 0, ArraySize(stochastic_main_value), stochastic_main_value); CopyBuffer(stochastic_signal_handle, SIGNAL_LINE, 0, ArraySize(stochastic_signal_value), stochastic_signal_value); for (int i = ArraySize(fast_ma_value) - 1; i >= 0; i--) { double fast_ma = fast_ma_value[i]; double medium_ma = medium_ma_value[i]; double slow_ma = slow_ma_value[i]; double stochastic_main = stochastic_main_value[i]; double stochastic_signal = stochastic_signal_value[i]; if (fast_ma > medium_ma && medium_ma > slow_ma && stochastic_main < Oversold_Level && stochastic_signal < Oversold_Level) { signal = 1; stopLossLevel = Bid - Stop_Loss_Multiplier * (medium_ma - slow_ma); takeProfitLevel = Bid + Take_Profit_Multiplier * (medium_ma - slow_ma); break; } else if (fast_ma < medium_ma && medium_ma < slow_ma && stochastic_main > Overbought_Level && stochastic_signal > Overbought_Level) { signal = -1; stopLossLevel = Ask + Stop_Loss_Multiplier * (medium_ma - slow_ma); takeProfitLevel = Ask - Take_Profit_Multiplier * (medium_ma - slow_ma); break; } else { signal = 0; } } if (signal == 1) { // Buy CTrade trade; trade.Buy(Volume, _Symbol, Ask, stopLossLevel, takeProfitLevel, "Buy Order"); } else if (signal == -1) { // Sell CTrade trade; trade.Sell(Volume, _Symbol, Bid, stopLossLevel, takeProfitLevel, "Sell Order"); } } //+------------------------------------------------------------------+
Thank you I do see what I did wrong there. I didn't use AI but I have been extensively youtube/googling and I guess you're bound to end up with something ridiculous at some point.
That being said I did update my code using CopyBuffer but still no orders being placed.
void OnTick() { Ask = SymbolInfoDouble(_Symbol, SYMBOL_ASK); Bid = SymbolInfoDouble(_Symbol, SYMBOL_BID); double fast_ma_value[], medium_ma_value[], slow_ma_value[]; double stochastic_main_value[], stochastic_signal_value[]; ArraySetAsSeries(fast_ma_value, true); ArraySetAsSeries(medium_ma_value, true); ArraySetAsSeries(slow_ma_value, true); ArraySetAsSeries(stochastic_main_value, true); ArraySetAsSeries(stochastic_signal_value, true); CopyBuffer(fast_ma_handle, 0, 0, ArraySize(fast_ma_value), fast_ma_value); CopyBuffer(medium_ma_handle, 0, 0, ArraySize(medium_ma_value), medium_ma_value); CopyBuffer(slow_ma_handle, 0, 0, ArraySize(slow_ma_value), slow_ma_value); CopyBuffer(stochastic_main_handle, MAIN_LINE, 0, ArraySize(stochastic_main_value), stochastic_main_value); CopyBuffer(stochastic_signal_handle, SIGNAL_LINE, 0, ArraySize(stochastic_signal_value), stochastic_signal_value); Comment(ArraySize(fast_ma_value)); // 0
[EDIT]
You are copying 0 values because the sizes of the arrays are zero

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use