Hi i have made an ea and it has no errors but fails to send orders, could someone help me find out why
It's better you learn it yourself!
Learn to use the debugger!
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
Also, as a newbie, you shouldn't start with a blank page!
Before you learn to program in MQL5, learn to search, because there is practically nothing that has not already been programmed for MT4/MT5!
=> Search in the articles: https://www.mql5.com/en/articles
=> Search in the codebase: https://www.mql5.com/en/code
=> Search in general: https://www.mql5.com/en/search or via Google with: "site:mql5.com .." (forgives typos and variants)
Hint: If you place the cursor on a MQL function and press F1, you will see the reference directly, many with examples to copy and paste - the fastest form to code!
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator
https://www.mql5.com/en/articles/35
and here are articles with about indicators, their interpretation and usage with code to copy & paste:
https://www.mql5.com/en/articles/13406
https://www.mql5.com/en/articles/13244
https://www.mql5.com/en/articles/13277
- www.metatrader5.com
ive done that and it doesnt give me anything to go off, thats why i came here to ask experienced individuals for help. Id say its definitely better to learn from people who know how to do it
It's better you learn it yourself!
Learn to use the debugger!
Code debugging: https://www.metatrader5.com/en/metaeditor/help/development/debug
Error Handling and Logging in MQL5: https://www.mql5.com/en/articles/2041
Tracing, Debugging and Structural Analysis of Source Code, scroll down to: "Launching and Debuggin": https://www.mql5.com/en/articles/272
Also, as a newbie, you shouldn't start with a blank page!
Before you learn to program in MQL5, learn to search, because there is practically nothing that has not already been programmed for MT4/MT5!
=> Search in the articles: https://www.mql5.com/en/articles
=> Search in the codebase: https://www.mql5.com/en/code
=> Search in general: https://www.mql5.com/en/search or via Google with: "site:mql5.com .." (forgives typos and variants)
Hint: If you place the cursor on a MQL function and press F1, you will see the reference directly, many with examples to copy and paste - the fastest form to code!
Introduction to MQL5: How to write simple Expert Advisor and Custom Indicator
https://www.mql5.com/en/articles/35
and here are articles with about indicators, their interpretation and usage with code to copy & paste:
https://www.mql5.com/en/articles/13406
https://www.mql5.com/en/articles/13244
https://www.mql5.com/en/articles/13277
ive done that and it doesnt give me anything to go off, thats why i came here to ask experienced individuals for help. Id say its definitely better to learn from people who know how to do it
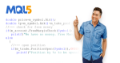
- 2024.02.21
- www.mql5.com
- Just because it compiles do not mean "it has no errors".
-
double stop_loss = NormalizeDouble(SymbolInfoDouble(Symbol(), SYMBOL_BID) + Stop_Loss * _Point, _Digits); double take_profit = NormalizeDouble(SymbolInfoDouble(Symbol(), SYMBOL_BID) - Take_Profit * _Point, _Digits);
You used NormalizeDouble, It's use is usually wrong, as it is in your case.
-
Floating point has an infinite number of decimals, it's you were not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum #10 (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
-
-
double stop_loss = NormalizeDouble(SymbolInfoDouble(Symbol(), SYMBOL_ASK) - Stop_Loss * _Point, _Digits); double take_profit = NormalizeDouble(SymbolInfoDouble(Symbol(), SYMBOL_ASK) + Take_Profit * _Point, _Digits); MqlTradeRequest request = {10}; request.action = TRADE_ACTION_DEAL; // Specify the trade action request.type = ORDER_TYPE_BUY;
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-
thanks, it worked now just need to adjust some settings so it works better
- Just because it compiles do not mean "it has no errors".
-
You used NormalizeDouble, It's use is usually wrong, as it is in your case.
-
Floating point has an infinite number of decimals, it's you were not understanding floating point and that some numbers can't be represented exactly. (like 1/10.)
Double-precision floating-point format - Wikipedia, the free encyclopediaSee also The == operand. - MQL4 programming forum (2013)
-
Print out your values to the precision you want with DoubleToString - Conversion Functions - MQL4 Reference.
-
SL/TP (stops) need to be normalized to tick size (not Point) — code fails on non-currencies.
On 5Digit Broker Stops are only allowed to be placed on full pip values. How to find out in mql? - MQL4 programming forum #10 (2011)And abide by the limits Requirements and Limitations in Making Trades - Appendixes - MQL4 Tutorial and that requires understanding floating point equality Can price != price ? - MQL4 programming forum (2012)
-
Open price for pending orders need to be adjusted. On Currencies, Point == TickSize, so you will get the same answer, but it won't work on non-currencies. So do it right.
Trailing Bar Entry EA - MQL4 programming forum (2013)
Bid/Ask: (No Need) to use NormalizeDouble in OrderSend - MQL4 programming forum (2012) -
Lot size must also be adjusted to a multiple of LotStep and check against min and max. If that is not a power of 1/10 then NormalizeDouble is wrong. Do it right.
(MT4 2013)) (MT5 2022)) -
MathRound() and NormalizeDouble() are rounding in a different way. Make it explicit.
MT4:NormalizeDouble - MQL5 programming forum (2017)
How to Normalize - Expert Advisors and Automated Trading - MQL5 programming forum (2017) -
Prices you get from the terminal are already correct (normalized).
-
PIP, Point, or Tick are all different in general.
What is a TICK? - MQL4 programming forum (2014)
-
-
You buy at the Ask and sell at the Bid. Pending Buy Stop orders become market orders when hit by the Ask.
-
Your buy order's TP/SL (or Sell Stop's/Sell Limit's entry) are triggered when the Bid / OrderClosePrice reaches it. Using Ask±n, makes your SL shorter and your TP longer, by the spread. Don't you want the specified amount used in either direction?
-
Your sell order's TP/SL (or Buy Stop's/Buy Limit's entry) will be triggered when the Ask / OrderClosePrice reaches it. To trigger close at a specific Bid price, add the average spread.
MODE_SPREAD (Paul) - MQL4 programming forum - Page 3 #25 -
The charts show Bid prices only. Turn on the Ask line to see how big the spread is (Tools → Options (control+O) → charts → Show ask line.)
Most brokers with variable spreads widen considerably at end of day (5 PM ET) ± 30 minutes.
My GBPJPY shows average spread = 26 points, average maximum spread = 134.
My EURCHF shows average spread = 18 points, average maximum spread = 106.
(your broker will be similar).
Is it reasonable to have such a huge spreads (20 PIP spreads) in EURCHF? - General - MQL5 programming forum (2022)
-

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi i have made an ea and it has no errors but fails to send orders, could someone help me find out why