This is very useful code. When I import to.fxdreema, it says ' no record found'
Would you upload.it again. Thanks.
69446729 #:
This is very useful code. When I import to.fxdreema, it says ' no record found'
Would you upload.it again. Thanks.
In this optimized version, we maintain a list of orders for the current symbol and update it whenever a new order is opened or closed. We also directly calculate drawdown using the maximum balance and current balance. These changes should improve the efficiency and performance of the expert advisor.
//+------------------------------------------------------------------+ //| BalanceDrawdownInMT4.mq4 | //| Copyright 2024, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2024, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" #property strict // Global variables bool ENABLE_MAGIC_NUMBER = true; // Enable Magic Number(For manual trades set false) int MAGIC_NUMBER_INPUT = 20131111; // Magic Number double START_BALANCE = 1000; // Start Balance double LOTS = 0.01; double STOPLOSS = 300; double TAKEPROFIT = 400; int MAGIC_NUMBER; double MaxBalance = START_BALANCE; // Maximum balance so far double CurrentBalance = START_BALANCE; // Current balance bool OrderListInitialized = false; // Flag to check if order list is initialized int OrdersList[]; // Array to store orders for the current symbol //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { if (ENABLE_MAGIC_NUMBER) MAGIC_NUMBER = MAGIC_NUMBER_INPUT; return (INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { // Initialize order list if (!OrderListInitialized) { InitializeOrderList(); OrderListInitialized = true; } // Open a buy order if no orders are present if (OrdersTotal() == 0) { int res = OrderSend(Symbol(), OP_BUY, LOTS, Ask, 3, Ask - STOPLOSS * Point(), Ask + TAKEPROFIT * Point(), "", MAGIC_NUMBER, 0, clrGreen); } // Update drawdown information UpdateDrawdown(); } //+------------------------------------------------------------------+ //| Initialize order list for the current symbol | //+------------------------------------------------------------------+ void InitializeOrderList() { ArrayResize(OrdersList, 0); for (int i = 0; i < OrdersTotal(); i++) { if (OrderSelect(i, SELECT_BY_POS, MODE_TRADES) && ((ENABLE_MAGIC_NUMBER && OrderMagicNumber() == MAGIC_NUMBER) || !ENABLE_MAGIC_NUMBER) && OrderSymbol() == Symbol()) { ArrayPushBack(OrdersList, i); } } } //+------------------------------------------------------------------+ //| Update drawdown information | //+------------------------------------------------------------------+ void UpdateDrawdown() { double currentProfit = TotalCurrentProfit(Symbol()); CurrentBalance = START_BALANCE + currentProfit; if (CurrentBalance > MaxBalance) MaxBalance = CurrentBalance; double drawdown = ((MaxBalance - CurrentBalance) * 100) / MaxBalance; Comment("Current Drawdown: " + DoubleToStr(drawdown, 2) + "%"); } //+------------------------------------------------------------------+ //| Get current total profit of a symbol | //+------------------------------------------------------------------+ double TotalCurrentProfit(string symbol) { double profit = 0; for (int i = 0; i < ArraySize(OrdersList); i++) { if (OrderSelect(OrdersList[i], SELECT_BY_POS, MODE_TRADES) && OrderSymbol() == symbol) profit += OrderProfit(); } return profit; }
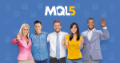
Discover new MetaTrader 5 opportunities with MQL5 community and services
- 2024.04.10
- www.mql5.com
MQL5: language of trade strategies built-in the MetaTrader 5 Trading Platform, allows writing your own trading robots, technical indicators, scripts and libraries of functions

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Calculate Drawdown in MT4:
this shortcode will calculate the drawdown of a particular ea, where it is applied to a specified symbol.
Author: Biswarup Banerjee