While working on a machine learning model I noticed a weird behavior on the Eig built-in method. When given a matrix
It returns:
Expected Behaviour:
The Eigen values calculating function has nothing to do with changing the dimensions of the eigen vectors so the eigen_vectors matrix is expected to be a 4x4 matrix just like the original matrix, meanwhile the eigen values vector should be expected to have the size of 4
Since most of the Matrix and Vector methods are inspired by python libraries as a documentation explains https://www.mql5.com/en/articles/9805
I took the same code to python:
The results were accurate:
I believe this is a major bug for all complex coders in MQL5, as this method is important in clustering and in dimension reduction algorithms.
There are more than 5 alghorithms to solve non-symmetric matrices. We don't know which algorithm used in Pyton
You can check correctness of obtained eigen vectors and eigen values.
Your example presented in documentation to demonstrate complex solution
See Note section
If a complex solution is encountered when calculating eigenvalues, the calculation is stopped and the error code is set to 4019 (ERR_MATH_OVERFLOW). Use the complex overload of the Eig method to obtain a complete solution in complex space
If a complex eigenvalue has an imaginary part equal to zero, then it is a real eigenvalue. This can be seen in the example below.
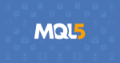
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
While working on a machine learning model I noticed a weird behavior on the Eig built-in method. When given a matrix
It returns:
Expected Behaviour:
The Eigen values calculating function has nothing to do with changing the dimensions of the eigen vectors so the eigen_vectors matrix is expected to be a 4x4 matrix just like the original matrix, meanwhile the eigen values vector should be expected to have the size of 4
Since most of the Matrix and Vector methods are inspired by python libraries as a documentation explains https://www.mql5.com/en/articles/9805
I took the same code to python:
The results were accurate:
I believe this is a major bug for all complex coders in MQL5, as this method is important in clustering and in dimension reduction algorithms.