this is my code
Could you please describe the logic you followed using post-increment here:
else if (TypeHOCLV == "HOLCV") { ArrayResize(DataHOCLV, ArraySize(DataHOCLV) * 5); for ( Di = 0; Di < ArraySize(DataHOCLV); Di++) { DataHOCLV[PosArr++] = iHigh(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iOpen(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iClose(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iLow(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iVolume(HowSymbolWant, _Period, Di); } } else if (TypeHOCLV == "HOLC") { ArrayResize(DataHOCLV, ArraySize(DataHOCLV) * 4); for ( Di = 0; Di < ArraySize(DataHOCLV); Di++) { DataHOCLV[PosArr++] = iHigh(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iOpen(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iClose(HowSymbolWant, _Period, Di); DataHOCLV[PosArr++] = iLow(HowSymbolWant, _Period, Di);
What is it there for? What does it do?
Could you please describe the logic you followed using post-increment here:
What is it there for? What does it do?
The block you are referring to has been modified by increasing its capacity five times to accommodate the data of Open, High, Low, Close, and Volume. This allows the first five values to be associated with one candle, and so on. Therefore, the index you see is used to select the various cells of the array.
My attempt to see which element you are requesting access to:
#property strict #define INT_NOT_USED 0 #define STRING_NOT_USED "" void OnStart() { double someArray[]; ArrayResize(someArray, 10); _ReturnHOCLVofXcandel(someArray, INT_NOT_USED, "HOLC", STRING_NOT_USED); } void _ReturnHOCLVofXcandel(double& DataHOCLV[], int ManyCandle, string TypeHOCLV, string HowSymbolWant) { int Di = 0; int PosArr = 0; if (TypeHOCLV == "HOLC") { ArrayResize(DataHOCLV, ArraySize(DataHOCLV) * 4); for ( Di = 0; Di < ArraySize(DataHOCLV); Di++) { PosArr++; PosArr++; PosArr++; PosArr++; } Alert(StringFormat("Array size %i, attempt to access element at index %i", ArraySize(DataHOCLV), PosArr - 1)); } }
ArrayResize(DataHOCLV, ArraySize(DataHOCLV) * 4);
suppose if i sett arraymany candel in top of function , set 10 he must give me 40
with this for ( Di = 0; Di < ArraySize(DataHOCLV); Di++) {
DataHOCLV[PosArr++] = iHigh(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iOpen(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iClose(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iLow(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iVolume(HowSymbolWant, _Period, Di);
}
for cicle for 10 time aaaa ok i must use ManyCandle in for because if i use arraysize after risize rigth but retrun me always many error
'myDataPrimario' - invalid array access DEMADE2.mq4 372 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 372 21
'myDataCorrelato' - invalid array access DEMADE2.mq4 373 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 373 22
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 372 21
Reason is here:
double myDataPrimario[]; double myDataCorrelato[]; myDataPrimario = _ReturnHOCLVofXcandel(myDataPrimario, 10, "O",Symbol()); myDataCorrelato = _ReturnHOCLVofXcandel(myDataCorrelato, 10, "O",Cross_Correlato);
This is hard to comment on. Such code only says that you need to learn the basics of programming
but how is possible if i do
suppose if i sett arraymany candel in top of function , set 10 he must give me 40
with this for ( Di = 0; Di < ArraySize(DataHOCLV); Di++) {
DataHOCLV[PosArr++] = iHigh(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iOpen(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iClose(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iLow(HowSymbolWant, _Period, Di);
DataHOCLV[PosArr++] = iVolume(HowSymbolWant, _Period, Di);
}
The number of iterations will be equal to the size of the array. But in each iteration you add 1 to the PosArr 5 times.
It looks like you're spending a lot of time trying to get the code to work, but you don't understand what you're doing. Better spend time learning programming. Or at least try to implement something easier.
A person who writes such functions will not do this:
double myDataPrimario[]; double myDataCorrelato[]; myDataPrimario = _ReturnHOCLVofXcandel(myDataPrimario, 10, "O",Symbol()); myDataCorrelato = _ReturnHOCLVofXcandel(myDataCorrelato, 10, "O",Cross_Correlato);But if you did write the functions, then why are you passing an array by reference, and then using an assignment operator to get the result of the function?
i know i am not good...., thanks you for reminding me, but i come here because i want to learn.not to show how I am cool when I write code :D, said this i follow the example here https://www.mql5.com/en/forum/117484 (function with array as return value? ) for return a array at function , i think the syntax is correct , ofcourse i did do a mistake in for i just fixed ,but problem return the same , if someone can help me or explain me much better , is welcome , also after the modify at a script return
'myDataPrimario' - invalid array access DEMADE2.mq4 372 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 372 21
'myDataCorrelato' - invalid array access DEMADE2.mq4 373 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 373 22
thanks at all
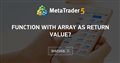
- 2009.05.23
- www.mql5.com
I didn't say it. What I'm talking about is that you are trying to implement complex things, having a misunderstanding of some basic. Try to simplify your task first.
'myDataPrimario' - invalid array access DEMADE2.mq4 372 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 372 21
'myDataCorrelato' - invalid array access DEMADE2.mq4 373 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 373 22
These errors are fixed like this:
double myDataPrimario[]; double myDataCorrelato[]; _ReturnHOCLVofXcandel(myDataPrimario, 10, "O",Symbol()); _ReturnHOCLVofXcandel(myDataCorrelato, 10, "O",Cross_Correlato);
But I think there should be "array out of range" for "HOLCV" and "HOLC" (this is not accurate, I didn't check)

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
the program to return open value of some candel is this
in main program i try to call with this code
but return me thIs error
'myDataPrimario' - invalid array access DEMADE2.mq4 372 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 372 21
'myDataCorrelato' - invalid array access DEMADE2.mq4 373 4
'_ReturnHOCLVofXcandel' - expression of 'void' type is illegal DEMADE2.mq4 373 22
anyone have some idea why ?