hello , i have this fuction to grow an array and return its latest element for immediate access :
I can't return a pointer to a primitive array element.
but i can do this :
its not a problem , just wondering is there a macro substititution i can use to do something like
thank you
Yes, you can:
#define grow_and_use_last(x) x[expand_primitive_array(x)] int primitive[]; grow_and_use_last(primitive) = 5;
template<typename X> void addToArray(X& array[], X value){ int iEnd=ArraySize(array); ArrayResize(array, iEnd+1); Array[iEnd]=value; }
That makes sure the value added is, or can be converted to, the proper type.
Always prefer actual functions to macros.
That makes sure the value added is, or can be converted to, the proper type.
I would suggest to extend the functionality, so the replacement is function-compatible with the macro:
template <typename X> const X addToArray(X& array[], const X new_value) { const int new_value_ptr = ArraySize(array); ArrayResize(array, new_value_ptr + 1); array[new_value_ptr] = new_value; return(new_value); };
Do you mean this? ...
Forum on trading, automated trading systems and testing trading strategies
any way to store expression into a variable or array?
Fernando Carreiro, 2022.08.18 21:03
There is also a way of using macros to "stringify" the expression, so that you don't have to type the expression twice. This will help keep the code consistent when you update the expressions.
However, this is a more power programmer coding skill that needs careful planing of the code logic, but you can research the "#" Stringification functionality of the standard C/C++ preprocessor which also works on MQL.
- https://users.informatik.haw-hamburg.de/~krabat/FH-Labor/gnupro/2_GNUPro_Compiler_Tools/The_C_Preprocessor/cppStringification.html
- https://renenyffenegger.ch/notes/development/languages/C-C-plus-plus/preprocessor/macros/stringify
- https://docs.microsoft.com/en-us/cpp/preprocessor/stringizing-operator-hash?view=msvc-170
Yes, you can define a macro substitution to achieve the functionality you're looking for. Here's an example of how you could define such a macro:
#define GROW_AND_USE_LAST(arr, val) arr[expand_primitive_array(arr)] = val;
With this macro, you can simply use the following code to grow the array and set the value of the last element:
GROW_AND_USE_LAST(primitive, 5);
This will expand the primitive array and set its last element to the value 5.
Note that this macro uses the function expand_primitive_array that you provided in your code.
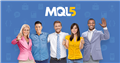
Yes, you can define a macro substitution to achieve the functionality you're looking for. Here's an example of how you could define such a macro:
#define GROW_AND_USE_LAST(arr, val) arr[expand_primitive_array(arr)] = val;
With this macro, you can simply use the following code to grow the array and set the value of the last element:
GROW_AND_USE_LAST(primitive, 5);
This will expand the primitive array and set its last element to the value 5.
Note that this macro uses the function expand_primitive_array that you provided in your code.
Thanks , that is very compact .
Ps : i like how seo optimized your reply is 😊
Google will rank a page higher if it finds the form :
Can i do X? (in the header)
- Yes, you can do X by .... (as much of the question present in the answer possible)
- No, you cannot do X ...
Because it makes more sense to the user and it means they can potentially find what they searched for.
If "Yes, you can do X by ..." is garbage however , the user will return to google , and the page will be penalized.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
hello , i have this fuction to grow an array and return its latest element for immediate access :
I can't return a pointer to a primitive array element.
but i can do this :
its not a problem , just wondering is there a macro substititution i can use to do something like
primitive[grow_and_use_last]=5;
thank you