- Coding help
- WHAT DID YOU DO?????????? HOW COULD YOU UPDATE YOUR SOFTWARE AND MAKE THIS NOT WORK??????????
- Help needed with EMA indicator
Learn to use the </> button to insert your code please.
You can check yourself by:
- the error message has line and char number to know exactly where the problem is.
- Or start the debugger: https://www.metatrader5.com/en/metaeditor/help/development/debug
- learn to use array. Here is a list of all function that deal with array: https://www.mql5.com/en/docs/array
Look e.g. for the example of ArrayResize() how a dynamic array is treated correctly.
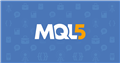
Documentation on MQL5: Array Functions
- www.mql5.com
Array Functions - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5
double ssma = iMA(_Symbol, PERIOD_CURRENT, (int)SSMA_Period, 0, MODE_SMMA, PRICE_CLOSE);
iMA does not return a double in MT5. Invalid call int MT4.
Stop using ChatGPT.
Error message in EA - 'OnInit' - function already defined and has body - MQL4 programming forum (2023)

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register