It is in the documentation, for example ... Documentation on MQL5: Python Integration / copy_rates_from
TIMEFRAME is an enumeration with possible chart period values
ID
Description
TIMEFRAME_M1
1 minute
TIMEFRAME_M2
2 minutes
TIMEFRAME_M3
3 minutes
TIMEFRAME_M4
4 minutes
TIMEFRAME_M5
5 minutes
TIMEFRAME_M6
6 minutes
TIMEFRAME_M10
10 minutes
TIMEFRAME_M12
12 minutes
TIMEFRAME_M12
15 minutes
TIMEFRAME_M20
20 minutes
TIMEFRAME_M30
30 minutes
TIMEFRAME_H1
1 hour
TIMEFRAME_H2
2 hours
TIMEFRAME_H3
3 hours
TIMEFRAME_H4
4 hours
TIMEFRAME_H6
6 hours
TIMEFRAME_H8
8 hours
TIMEFRAME_H12
12 hours
TIMEFRAME_D1
1 day
TIMEFRAME_W1
1 week
TIMEFRAME_MN1
1 month
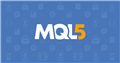
- www.mql5.com
Background
I often got upset about not having much in thé way of pythonic programmatic access to timeframes and their attributes, not to mention the non sequential and byte shifted values of the higher timeframes.
For example, if I wanted a python function that took a timeframe as an argument, I invariable ended up hard coding it, and as for a list of timeframes...
Solution
I then came across the wonderful TimeFrames.mqh by @nicholish en and used it to write a script to write a python script that defines a function to define a python dictionary.The MQL5 script is as follows:
//+------------------------------------------------------------------+ //| tfs.mq5 | //| Copyright 2023, Andy Thompson | //| mailto:andydoc@gmail.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2023, Andy Thompson" #property link "mailto:andydoc@gmail.com" #property version "1.00" #include <TimeFrames.mqh> //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { CTimeFrames tfs; // using all periods when calling the default constructor string res = "dictName = {}\n"; string pyFile = "#Returns dict dictName of records: dictName['mt5.%timeframe%'] = {'mt5 value' : %int enumeration value%, 'minutes' : %int minutes%, 'seconds' : %int seconds%}\ndef getTimeframes(dictName):\n\t"; for(tfs = PERIOD_M1; tfs <= PERIOD_MN1;tfs++) { res+= StringFormat("\tdictName['mt5.%s'] = {'mt5 value' : %u, 'int minutes' : %s, 'int seconds' : %s}",tfs.ToString(),tfs.Period(),(string)(tfs.ToSeconds()/60),(string)tfs.ToSeconds()); if(tfs.Period() != PERIOD_MN1){ res+= "\n"; } } StringAdd(pyFile,res); StringAdd(pyFile,"\n\treturn dictName"); Print(pyFile); int filehandle=FileOpen("getTimeframes.py",FILE_WRITE|FILE_TXT,0,CP_UTF8); FileWriteString(filehandle,pyFile); FileClose(filehandle); } //+------------------------------------------------------------------+
When run, this saves a python script called getTimeframes.py which looks like this:
#Returns dict dictName of records: dictName['mt5.%timeframe%'] = {'mt5 value' : %int enumeration value%, 'minutes' : %int minutes%, 'seconds' : %int seconds%} def getTimeframes(dictName): dictName = {} dictName['mt5.PERIOD_M1'] = {'mt5 value' : 1, 'int minutes' : 1, 'int seconds' : 60} dictName['mt5.PERIOD_M2'] = {'mt5 value' : 2, 'int minutes' : 2, 'int seconds' : 120} dictName['mt5.PERIOD_M3'] = {'mt5 value' : 3, 'int minutes' : 3, 'int seconds' : 180} dictName['mt5.PERIOD_M4'] = {'mt5 value' : 4, 'int minutes' : 4, 'int seconds' : 240} dictName['mt5.PERIOD_M5'] = {'mt5 value' : 5, 'int minutes' : 5, 'int seconds' : 300} dictName['mt5.PERIOD_M6'] = {'mt5 value' : 6, 'int minutes' : 6, 'int seconds' : 360} dictName['mt5.PERIOD_M10'] = {'mt5 value' : 10, 'int minutes' : 10, 'int seconds' : 600} dictName['mt5.PERIOD_M12'] = {'mt5 value' : 12, 'int minutes' : 12, 'int seconds' : 720} dictName['mt5.PERIOD_M15'] = {'mt5 value' : 15, 'int minutes' : 15, 'int seconds' : 900} dictName['mt5.PERIOD_M20'] = {'mt5 value' : 20, 'int minutes' : 20, 'int seconds' : 1200} dictName['mt5.PERIOD_M30'] = {'mt5 value' : 30, 'int minutes' : 30, 'int seconds' : 1800} dictName['mt5.PERIOD_H1'] = {'mt5 value' : 16385, 'int minutes' : 60, 'int seconds' : 3600} dictName['mt5.PERIOD_H2'] = {'mt5 value' : 16386, 'int minutes' : 120, 'int seconds' : 7200} dictName['mt5.PERIOD_H3'] = {'mt5 value' : 16387, 'int minutes' : 180, 'int seconds' : 10800} dictName['mt5.PERIOD_H4'] = {'mt5 value' : 16388, 'int minutes' : 240, 'int seconds' : 14400} dictName['mt5.PERIOD_H6'] = {'mt5 value' : 16390, 'int minutes' : 360, 'int seconds' : 21600} dictName['mt5.PERIOD_H8'] = {'mt5 value' : 16392, 'int minutes' : 480, 'int seconds' : 28800} dictName['mt5.PERIOD_H12'] = {'mt5 value' : 16396, 'int minutes' : 720, 'int seconds' : 43200} dictName['mt5.PERIOD_D1'] = {'mt5 value' : 16408, 'int minutes' : 1440, 'int seconds' : 86400} dictName['mt5.PERIOD_W1'] = {'mt5 value' : 32769, 'int minutes' : 10080, 'int seconds' : 604800} dictName['mt5.PERIOD_MN1'] = {'mt5 value' : 49153, 'int minutes' : 43200, 'int seconds' : 2592000} return dictName
Useage
>>> import MetaTrader5 as mt5
>>> from getTimeframes import getTimeframes
>>> inp_path = "C:/Program Files/FTMO MetaTrader 5/terminal64.exe"
>>> mt5.initialize(inp_path)
True
>>> tfs = getTimeframes("tfs")
>>> for k, v in tfs.items():
... print(k,v)
mt5.PERIOD_M1 {'mt5 value': 1, 'int minutes': 1, 'int seconds': 60}
mt5.PERIOD_M2 {'mt5 value': 2, 'int minutes': 2, 'int seconds': 120}
mt5.PERIOD_M3 {'mt5 value': 3, 'int minutes': 3, 'int seconds': 180}
mt5.PERIOD_M4 {'mt5 value': 4, 'int minutes': 4, 'int seconds': 240}
mt5.PERIOD_M5 {'mt5 value': 5, 'int minutes': 5, 'int seconds': 300}
mt5.PERIOD_M6 {'mt5 value': 6, 'int minutes': 6, 'int seconds': 360}
mt5.PERIOD_M10 {'mt5 value': 10, 'int minutes': 10, 'int seconds': 600}
mt5.PERIOD_M12 {'mt5 value': 12, 'int minutes': 12, 'int seconds': 720}
mt5.PERIOD_M15 {'mt5 value': 15, 'int minutes': 15, 'int seconds': 900}
mt5.PERIOD_M20 {'mt5 value': 20, 'int minutes': 20, 'int seconds': 1200}
mt5.PERIOD_M30 {'mt5 value': 30, 'int minutes': 30, 'int seconds': 1800}
mt5.PERIOD_H1 {'mt5 value': 16385, 'int minutes': 60, 'int seconds': 3600}
mt5.PERIOD_H2 {'mt5 value': 16386, 'int minutes': 120, 'int seconds': 7200}
mt5.PERIOD_H3 {'mt5 value': 16387, 'int minutes': 180, 'int seconds': 10800}
mt5.PERIOD_H4 {'mt5 value': 16388, 'int minutes': 240, 'int seconds': 14400}
mt5.PERIOD_H6 {'mt5 value': 16390, 'int minutes': 360, 'int seconds': 21600}
mt5.PERIOD_H8 {'mt5 value': 16392, 'int minutes': 480, 'int seconds': 28800}
mt5.PERIOD_H12 {'mt5 value': 16396, 'int minutes': 720, 'int seconds': 43200}
mt5.PERIOD_D1 {'mt5 value': 16408, 'int minutes': 1440, 'int seconds': 86400}
mt5.PERIOD_W1 {'mt5 value': 32769, 'int minutes': 10080, 'int seconds': 604800}
mt5.PERIOD_MN1 {'mt5 value': 49153, 'int minutes': 43200, 'int seconds': 2592000}
Sweet...
And finally:
>>> for k, v in tfs.items():
... print(k,":")
... ohlcv_data = mt5.copy_rates_from_pos("EURUSD", tfs[k]['mt5 value'], 0,2)
... print(ohlcv_data)
mt5.PERIOD_M1 :
[(1698450780, 1.05644, 1.0565 , 1.05638, 1.0565 , 26, 3, 0)
(1698450840, 1.05649, 1.05655, 1.05649, 1.05652, 29, 3, 0)]
mt5.PERIOD_M2 :
[(1698450720, 1.05646, 1.05652, 1.05638, 1.0565 , 54, 2, 0)
(1698450840, 1.05649, 1.05655, 1.05649, 1.05652, 29, 3, 0)]
mt5.PERIOD_M3 :
[(1698450660, 1.05648, 1.05652, 1.05638, 1.0565 , 80, 2, 0)
(1698450840, 1.05649, 1.05655, 1.05649, 1.05652, 29, 3, 0)]
mt5.PERIOD_M4 :
[(1698450480, 1.05646, 1.05656, 1.05645, 1.05646, 168, 2, 0)
(1698450720, 1.05646, 1.05655, 1.05638, 1.05652, 83, 2, 0)]
mt5.PERIOD_M5 :
[(1698450300, 1.05638, 1.05656, 1.05628, 1.05653, 204, 2, 0)
(1698450600, 1.05652, 1.05655, 1.05638, 1.05652, 153, 2, 0)]
mt5.PERIOD_M6 :
[(1698450480, 1.05646, 1.05656, 1.05638, 1.0565 , 222, 2, 0)
(1698450840, 1.05649, 1.05655, 1.05649, 1.05652, 29, 3, 0)]
mt5.PERIOD_M10 :
[(1698450000, 1.0564 , 1.05656, 1.05628, 1.05653, 332, 2, 0)
(1698450600, 1.05652, 1.05655, 1.05638, 1.05652, 153, 2, 0)]
mt5.PERIOD_M12 :
[(1698449760, 1.05655, 1.05657, 1.05628, 1.05646, 324, 2, 0)
(1698450480, 1.05646, 1.05656, 1.05638, 1.05652, 251, 2, 0)]
mt5.PERIOD_M15 :
[(1698449400, 1.05669, 1.05673, 1.05631, 1.05637, 328, 2, 0)
(1698450300, 1.05638, 1.05656, 1.05628, 1.05652, 357, 2, 0)]
mt5.PERIOD_M20 :
[(1698448800, 1.05673, 1.05678, 1.05638, 1.05639, 343, 2, 0)
(1698450000, 1.0564 , 1.05656, 1.05628, 1.05652, 485, 2, 0)]
mt5.PERIOD_M30 :
[(1698447600, 1.05708, 1.05708, 1.05664, 1.0567 , 671, 2, 0)
(1698449400, 1.05669, 1.05673, 1.05628, 1.05652, 685, 2, 0)]
mt5.PERIOD_H1 :
[(1698444000, 1.05675, 1.05708, 1.05619, 1.05704, 2486, 2, 0)
(1698447600, 1.05708, 1.05708, 1.05628, 1.05652, 1356, 2, 0)]
mt5.PERIOD_H2 :
[(1698436800, 1.05827, 1.0583 , 1.05572, 1.05676, 6869, 2, 0)
(1698444000, 1.05675, 1.05708, 1.05619, 1.05652, 3842, 2, 0)]
mt5.PERIOD_H3 :
[(1698429600, 1.05782, 1.05971, 1.05572, 1.05672, 10520, 2, 0)
(1698440400, 1.0567 , 1.05736, 1.05619, 1.05652, 7214, 2, 0)]
mt5.PERIOD_H4 :
[(1698422400, 1.05559, 1.05971, 1.05554, 1.05829, 20708, 2, 0)
(1698436800, 1.05827, 1.0583 , 1.05572, 1.05652, 10711, 2, 0)]
mt5.PERIOD_H6 :
[(1698408000, 1.05618, 1.05946, 1.05351, 1.05782, 25673, 2, 0)
(1698429600, 1.05782, 1.05971, 1.05572, 1.05652, 17734, 2, 0)]
mt5.PERIOD_H8 :
[(1698393600, 1.05671, 1.05699, 1.05351, 1.05559, 21972, 2, 0)
(1698422400, 1.05559, 1.05971, 1.05554, 1.05652, 31419, 2, 0)]
mt5.PERIOD_H12 :
[(1698364800, 1.05571, 1.05699, 1.05521, 1.05619, 19535, 2, 0)
(1698408000, 1.05618, 1.05971, 1.05351, 1.05652, 43407, 2, 0)]
mt5.PERIOD_D1 :
[(1698278400, 1.05675, 1.05691, 1.05216, 1.0561 , 74318, 2, 0)
(1698364800, 1.05571, 1.05971, 1.05351, 1.05652, 62942, 2, 0)]
mt5.PERIOD_W1 :
[(1697328000, 1.05101, 1.06166, 1.05062, 1.05949, 352970, 2, 0)
(1697932800, 1.05935, 1.0694 , 1.05216, 1.05652, 337848, 2, 0)]
mt5.PERIOD_MN1 :
[(1693526400, 1.08409, 1.08816, 1.0488 , 1.0573 , 1205586, 2, 0)
(1696118400, 1.05659, 1.0694 , 1.04482, 1.05652, 1486246, 2, 0)]
Of course this can be extended to have hour and day values, and can be used to parse other period representations such as "M15" to reverse lookup the mt5 value in the dictionary to pass to mt5 function calls in python.
Hope it is useful to some!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Where is the documentation for the different timeframes i can querry? Using python mt5 library
https://www.mql5.com/en/docs/python_metatrader5