You seem to missing "MQLInfoInteger( MQL_TRADE_ALLOWED )" ... Documentation on MQL5: MQL5 programs / Trade Permission
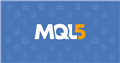
- www.mql5.com
Good day All!
I am trying to create a method to check if trading is allowed, if the trading context is busy, if the symbol has restrictions (long only/short only), etc.
Here is what I came up with, I am wondering if anyone would have some suggestions concerning other Account/Symbol related prechecks before sending an order.
I also have a method to check for market hours but I need to manually input the hours, is there another way that could automatically adapt to different markets without having to change the inputs myself?
Note: "MqlDateTime Time" is getting refreshed every tick in another method. Current hour inputs are for Forex on GMT TimeLocal().
Thank you in advance guys! I hope someone can give me tips to improve those prechecks!
Cheers,
Hello
You can automatically retrieve the Trading session for an asset and you will no longer have to worry about manually providing hours for that .
bool IsAssetInTradingSession(string asset,ENUM_DAY_OF_WEEK day_of_week){ bool result=false; datetime session_from=0,session_to=0; //find total sessions int sessions_total=0; for(int se=0;se<10;se++) { bool sessexist=SymbolInfoSessionTrade(asset,day_of_week,se,session_from,session_to); //if session exists if(sessexist){sessions_total=se+1;} else{break;} } //loop into all sessions and if we are not in any of them , return false for(int se=0;se<sessions_total;se++) { SymbolInfoSessionTrade(asset,day_of_week,se,session_from,session_to); //result is "For the day requested ,i.e , today , which server hours can you trade at //turn starting time in seconds , i think it is already //so , get the server time datetime servertime=TimeTradeServer(); //turn MqlDateTime mqdt_server; bool turn=TimeToStruct(servertime,mqdt_server); if(turn){ //zero minutes , hours and seconds mqdt_server.hour=0;mqdt_server.min=0;mqdt_server.sec=0; //back to datetime servertime=StructToTime(mqdt_server); //project start server time and end server time by adding the seconds from step one respectively session_from=(datetime)((int)(servertime)+(int)(session_from)); session_to=(datetime)((int)(servertime)+(int)(session_to)); //Print(asset+" server session from "+TimeToString(session_from,TIME_DATE|TIME_MINUTES|TIME_SECONDS)); //Print(asset+" server session to "+TimeToString(session_to,TIME_DATE|TIME_MINUTES|TIME_SECONDS)); //if we are within that time window then cool if(TimeTradeServer()>=session_from&&TimeTradeServer()<=session_to){return(true);} } }//loop into sessions ends here return(false); }
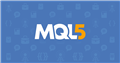
- www.mql5.com

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Good day All!
I am trying to create a method to check if trading is allowed, if the trading context is busy, if the symbol has restrictions (long only/short only), etc.
Here is what I came up with, I am wondering if anyone would have some suggestions concerning other Account/Symbol related prechecks before sending an order.
I also have a method to check for market hours but I need to manually input the hours, is there another way that could automatically adapt to different markets without having to change the inputs myself?
Note: "MqlDateTime Time" is getting refreshed every tick in another method. Current hour inputs are for Forex on GMT TimeLocal().
Thank you in advance guys! I hope someone can give me tips to improve those prechecks!
Cheers,