Mag.
You would have to approach it using object-oriented programming concepts. For example, you could use an array of a structure (or class) which includes a buffer array, with methods for initialisation and manipulation, so that you could then use a loop over this array to work with the multiple buffers.
Topics concerning MT4 and MQL4 have their own section.
In future please post in the correct section.
I have moved your topic to the MQL4 and Metatrader 4 section.
@Keith Watford thanks for correcting my mistake, Ill pay attention now onwards.
@Fernando Carreiro i appreciate your insight very much thank you. I have no idea how to go about the path you are explaining, practically.
I would have thought there must be a way to work with an "array of referenced arrays" in mql4.
I tried 'optimizing' the code a little and noticed how slow the indicator becomes when i run the loop per buffer as opposed to all buffer in one loop. So i really need to find out how to make an array made of other arrays. Preferably a & not a copy.
If anyone has any ideas, that would be fantastic. Thanks a lot for now
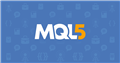
Here is an example using array of structure ...
#property strict #property indicator_chart_window #define MBufferCount 15 struct SBuffer { double m_dbBuffer[]; }; SBuffer oBuffers[ MBufferCount ]; int OnInit() { for( int i=0; i < MBufferCount; i++ ) SetIndexBuffer( i, oBuffers[ i ].m_dbBuffer ); return(INIT_SUCCEEDED); }; int OnCalculate( const int rates_total, const int prev_calculated, const datetime &time[], const double &open[], const double &high[], const double &low[], const double &close[], const long &tick_volume[], const long &volume[], const int &spread[] ) { double some_value = EMPTY_VALUE; for( int i = rates_total - ( ( prev_calculated < 1 ) ? 1 : prev_calculated ); i >= 0; i-- ) { for( int j = 0; j < MBufferCount; j++ ) { oBuffers[ j ].m_dbBuffer[ i ] = some_value; }; }; return rates_total; };
int i = rates_total - ( ( prev_calculated < 1 ) ? 1 : prev_calculated )Cheers!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello everyone, hope you are all doing great!
I would love to know if theres a method to deal with multiple buffers. My thought is to use iMA() function to pull data from any symbol and any timeframe. To produce something like the image bellow: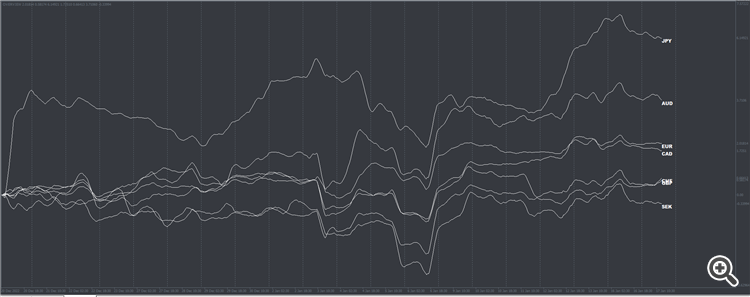
The problem becomes managing these buffers, from including, declaring, initializing, update etc..
Im missing the knowledge on how to create a Multi-dimensional array that would reference all 'ExtLineBuffers', so i can Loop onu it and access all buffers in on place, if this makes sense.
The code I prototyped is this:
The goal would be to eliminate the need to go thru all the code, checking all manual iterations for mistakes or inclusions ( i.e. Buffer1[0], Buffer2[0], ..., Buffer15[0] )
Thanks very much if you read this,
Mag.