- Please Help - How to select order by ticket by using CTrade library
- OrderSelect Problem
- How to get profit / loss of 0.01
I am trying to get the execution time of a pending order so my trade manager can check if it is the current M5 bar, but I get 0. I even found one article that suggested I use OrderSelect first, which I did and I still get 0. I'm also not sure why I need OrderSelect since the documentation code (that I use) gets the ticket number, type, magic, etc. without ever selecting the order. I really hope someone can help. Thanks!
Is the bool return value of your OrderSelect() true?
"I'm also not sure why I need OrderSelect since the documentation code (that I use) gets the ticket number, type, magic, etc. without ever selecting the order."
Not sure what you mean by this - please read this for an explanation: https://www.mql5.com/en/docs/trading/orderselect
Thank you for replying. Here is the code to answer the question and yes, OrderSelect = true, because the Print function show up in the Experts tab.
totalOrders=OrdersTotal(); // total number of placed pending orders //--- iterate over all placed pending orders for(int i=0; i<totalOrders; i++) { //--- parameters of the order ulong order_ticket=OrderGetTicket(i); // order ticket string order_symbol=Symbol(); // symbol ENUM_ORDER_TYPE type=(ENUM_ORDER_TYPE)OrderGetInteger(ORDER_TYPE); // type of the order double orderPrice=OrderGetDouble(ORDER_PRICE_OPEN); magic=OrderGetInteger(ORDER_MAGIC); // MagicNumber of the order if(OrderSelect(order_ticket)) { time_setup =(datetime)OrderGetInteger(ORDER_TIME_DONE); orderBarTime = iTime(Symbol(),PERIOD_M5,iBarShift(Symbol(),PERIOD_M5,time_setup)); Print("time_setup=",time_setup," orderBarTime=",orderBarTime); } else { Alert("error selecting order"); }
I have tried datetime, integer and ENUM_ORDER_PROPERTY_INTEGER, and I still get 0 for time_setup (ORDER_TIME_DONE). I have no trouble getting ticket time from a Position, but can't figure out what to do to get the Order time.
Thank you for replying. Here is the code to answer the question and yes, OrderSelect = true, because the Print function show up in the Experts tab.
I have tried datetime, integer and ENUM_ORDER_PROPERTY_INTEGER, and I still get 0 for time_setup (ORDER_TIME_DONE). I have no trouble getting ticket time from a Position, but can't figure out what to do to get the Order time.
I compiled in MQL5 - assuming you are trying to read a pending order which is yet to be executed, you need to use ORDER_TIME_SETUP - this returns the time.
Some other observations/recommendations:
1) Read the symbol from the order data
2) OrderSelect() should be earlier - before using any OrderGet function
3) Define all variables before the for loop - no need to keep re-instantiating on each iteration, plus the scope is wider
Here is an adjusted example
//+------------------------------------------------------------------+ //| 429183-OrderTimeDone.mq5 | //| Copyright 2022, MetaQuotes Ltd. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2022, MetaQuotes Ltd." #property link "https://www.mql5.com" #property version "1.00" //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ // https://www.mql5.com/en/forum/429183/ //+------------------------------------------------------------------+ //| | //+------------------------------------------------------------------+ void OnStart() { long magic = 0; datetime time_setup = 0; datetime orderBarTime = 0; string order_symbol = ""; double orderPrice = 0; ulong order_ticket = 0; ENUM_ORDER_TYPE type; int totalOrders = OrdersTotal(); // total number of placed pending orders //--- iterate over all placed pending orders for(int i = 0; i < totalOrders; i++) { //--- parameters of the order order_ticket = OrderGetTicket(i); // order ticket if(OrderSelect(order_ticket)) { order_symbol = OrderGetString(ORDER_SYMBOL); // symbol type = (ENUM_ORDER_TYPE)OrderGetInteger(ORDER_TYPE); // type of the order orderPrice = OrderGetDouble(ORDER_PRICE_OPEN); magic = OrderGetInteger(ORDER_MAGIC); // MagicNumber of the order time_setup = (datetime)OrderGetInteger(ORDER_TIME_SETUP); orderBarTime = iTime(Symbol(),PERIOD_M5,iBarShift(Symbol(),PERIOD_M5,time_setup)); Print("time_setup=",time_setup," orderBarTime=",orderBarTime); } else { Alert("error selecting order"); } } } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+
I am trying to get the execution time of a pending order so my trade manager can check if it is the current M5 bar, but I get 0. I even found one article that suggested I use OrderSelect first, which I did and I still get 0. I'm also not sure why I need OrderSelect since the documentation code (that I use) gets the ticket number, type, magic, etc. without ever selecting the order. I really hope someone can help. Thanks!
What do you mean by " execution time of a pending order"?
Is it the time for the order to enter the server queue successfully?
Or is it the time when an existing order on the server is triggered and a new position is created?
If you mean the latter, then the use of OrderSelect() is wrong in the first step.
You should use HistorySelect() because Order is no longer in the Order queue when triggered.
What do you mean by " execution time of a pending order"?
Is it the time for the order to enter the server queue successfully?
Or is it the time when an existing order on the server is triggered and a new position is created?
If you mean the latter, then the use of OrderSelect() is wrong in the first step.
You should use HistorySelect() because Order is no longer in the Order queue when triggered.
HistorySelect() uses from and to dates as input arguments so that is a different approach, which could bring back a list rather than reference a single pending order.
I suspect if you read " execution time" as "ORDER_TIME_SETUP" it will be the right interpretation of the query.
HistorySelect() uses from and to dates as input arguments so that is a different approach, which could bring back a list rather than reference a single pending order.
I suspect if you read " execution time" as "ORDER_TIME_SETUP" it will be the right interpretation of the query.
if ORDER_TIME_SETUP is for "execution" then ORDER_TIME_DONE is for ???
I really think the poster should clarify exactly what time he was trying to get?
if ORDER_TIME_SETUP is for "execution" then ORDER_TIME_DONE is for ???
I really think the poster should clarify exactly what time he was trying to get?
Have a read of the documentation to see the difference https://www.mql5.com/en/docs/constants/tradingconstants/orderproperties
Maybe the OP was wrong to select ORDER_TIME_DONE (which would not be valid when in pending status), but that is the crux of the issue I think.
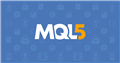
- www.mql5.com
Thank you, R4tna C. Your explanation is much clearer than the confusing MQ5 documentation. Working fine now! I guess Order Time Done is when the pending becomes a position, but that is not clear in the documentation.
Yes a little confusing at first, but makes sense once understood
//error time_setup = (datetime)OrderGetInteger(ORDER_TIME_SETUP); //correct time_setup = (datetime)OrderGetInteger(ORDER_TIME);

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use