Second if statement is not executed after the first if statement's conditions comes false. My code is attached below. Please can someone point me in the right direction.
Henihollar:
Second if statement is not executed after the first if statement's conditions comes false. Please can someone point me in the right direction.
what makes you think that? else if says it does get executed. most likely the conditions of the second if are also false.
use the debugger and check the values
Henihollar: Second if statement is not executed after the first if statement's conditions comes false. Please can someone point me in the right direction.
Be careful of the if-else construct you are using. It can be very misleading.
This is what you are doing ...
if ( condition1 ) { // Something if ... } else if (condition2 ) { // Something else if ... };
This is what it is actually doing ...
if ( condition1 ) { // Something if ... } else { if (condition2 ) { // Something else if ... }; // Something else ... };
You can write it like this for more control ...
if ( condition1 ) { // Something if ... } else { if (condition2 ) { // Something else if ... } else { // Something else else ... }; // Something else ... };
Henihollar :
You make a gross mistake: you create a new indicator handle on each tick. This is the biggest mistake!
According to the MQL5 style, the indicator handle is created ONCE!!! And this is done in OnInit.
Example:
An example of get values from the iRSI indicator
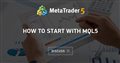
How to start with MQL5
- 2020.07.05
- www.mql5.com
This thread discusses MQL5 code examples. There will be examples of how to get data from indicators, how to program advisors...

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Second if statement is not executed after the first if statement's conditions comes false. Please can someone point me in the right direction.