You are making a gross mistake: you are creating an indicator handle on every tick ! Remember, according to the MQL5 style, the indicator handle should be created ONCE and it should be done in OnInit!
An example of working with the indicator:
Forum on trading, automated trading systems and testing trading strategies
Vladimir Karputov, 2021.06.05 06:59
Yes, Fractals can contain '0.0' or 'EMPTY_VALUE'. Example:
Code: 'iFractals.mq5'
//+------------------------------------------------------------------+ //| iFractals.mq5 | //| Copyright © 2021, Vladimir Karputov | //+------------------------------------------------------------------+ #property copyright "Copyright © 2021, Vladimir Karputov" #property version "1.000" //--- input parameters input int InputBars=9; //--- int handle_iFractals; // variable for storing the handle of the iFractals indicator bool m_init_error = false; // error on InInit //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- forced initialization of variables m_init_error = false; // error on InInit //--- create handle of the indicator iFractals handle_iFractals=iFractals(Symbol(),Period()); //--- if the handle is not created if(handle_iFractals==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iFractals indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early m_init_error=true; return(INIT_SUCCEEDED); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(m_init_error) return; //--- double upper[],lower[]; ArraySetAsSeries(upper,true); ArraySetAsSeries(lower,true); int start_pos=0,count=6; if(!iGetArray(handle_iFractals,UPPER_LINE,start_pos,count,upper) || !iGetArray(handle_iFractals,LOWER_LINE,start_pos,count,lower)) return; //--- string text=""; for(int i=0; i<count; i++) { //--- string text_upper=""; if(upper[i]==0.0 || upper[i]==EMPTY_VALUE) text_upper=""; else text_upper=DoubleToString(upper[i],Digits()); //--- string text_lower=""; if(lower[i]==0.0 || lower[i]==EMPTY_VALUE) text_lower=""; else text_lower=DoubleToString(lower[i],Digits()); //--- text=text+IntegerToString(i)+"#: "+"Upper "+text_upper+", Lower "+text_lower+"\n"; } Comment(text); } //+------------------------------------------------------------------+ //| Get value of buffers | //+------------------------------------------------------------------+ bool iGetArray(const int handle,const int buffer,const int start_pos, const int count,double &arr_buffer[]) { bool result=true; if(!ArrayIsDynamic(arr_buffer)) { PrintFormat("ERROR! EA: %s, FUNCTION: %s, this a no dynamic array!",__FILE__,__FUNCTION__); return(false); } ArrayFree(arr_buffer); //--- reset error code ResetLastError(); //--- fill a part of the iBands array with values from the indicator buffer int copied=CopyBuffer(handle,buffer,start_pos,count,arr_buffer); if(copied!=count) { //--- if the copying fails, tell the error code PrintFormat("ERROR! EA: %s, FUNCTION: %s, amount to copy: %d, copied: %d, error code %d", __FILE__,__FUNCTION__,count,copied,GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } return(result); } //+------------------------------------------------------------------+
Result:
You are making a gross mistake: you are creating an indicator handle on every tick ! Remember, according to the MQL5 style, the indicator handle should be created ONCE and it should be done in OnInit!
An example of working with the indicator:
Thank you Vladimir Karputov i hav modfied it but wont take any trades see the code below
#include <Trade/Trade.mqh> //--- input parameters input int InputBars=9; //--- int handle_iFractals; // variable for storing the handle of the iFractals indicator bool m_init_error = false; // error on InInit ulong posTicket; CTrade trade; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- forced initialization of variables m_init_error = false; // error on InInit //--- create handle of the indicator iFractals handle_iFractals=iFractals(Symbol(),Period()); //--- if the handle is not created if(handle_iFractals==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iFractals indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early m_init_error=true; return(INIT_SUCCEEDED); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(m_init_error) return; //--- //we calculate ask price double Ask=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //we calculate Bid price double Bid=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID),_Digits); //We create empty string for the signal string signal=""; //create a price array MqlRates PriceArray[]; //Create fractals array double upper[],lower[]; ArraySetAsSeries(upper,true); ArraySetAsSeries(lower,true); // sort the array from the current candle downwards ArraySetAsSeries(PriceArray,true); //fill the array with price data //int Data=CopyRates(_Symbol,Period(),start_pos,count,PriceArray); int start_pos=0,count=6; //fill the array with price data int Data=CopyRates(_Symbol,Period(),start_pos,count,PriceArray); if(!iGetArray(handle_iFractals,UPPER_LINE,start_pos,count,upper) || !iGetArray(handle_iFractals,LOWER_LINE,start_pos,count,lower)) return; //Calculate the value for the Last candle double lcupper=upper[1]; double lclower=lower[1]; //Reset if value is empty if(lcupper==EMPTY_VALUE) lcupper=0; //Reset if value is empty if(lclower==EMPTY_VALUE) lclower=0; //Buy signal //if it going up if(lclower!=0) if(lclower>PriceArray[1].low) {signal="buy"; } //Sell signal //if it going down if(lcupper!=0) if(lcupper>PriceArray[1].high) {signal="sell"; } //sell 10 microlot if (signal=="sell"&&PositionsTotal()<1) trade.Buy(0.1,NULL,Bid,(Bid+200*_Point),(Bid-150*_Point),NULL); //BUY 10 microlot if (signal=="buy"&&PositionsTotal()<1) trade.Buy(0.1,NULL,Ask,(Ask-200*_Point),(Ask+150*_Point),NULL); //--- string text=""; for(int i=0; i<count; i++) { //--- string text_upper=""; if(upper[i]==0.0 || upper[i]==EMPTY_VALUE) text_upper=""; else text_upper=DoubleToString(upper[i],Digits()); //--- string text_lower=""; if(lower[i]==0.0 || lower[i]==EMPTY_VALUE) text_lower=""; else text_lower=DoubleToString(lower[i],Digits()); //--- text=text+IntegerToString(i)+"#: "+"Upper "+text_upper+", Lower "+text_lower+"\n"; } Comment(text); } //+------------------------------------------------------------------+ //| Get value of buffers | //+------------------------------------------------------------------+ bool iGetArray(const int handle,const int buffer,const int start_pos, const int count,double &arr_buffer[]) { bool result=true; if(!ArrayIsDynamic(arr_buffer)) { PrintFormat("ERROR! EA: %s, FUNCTION: %s, this a no dynamic array!",__FILE__,__FUNCTION__); return(false); } ArrayFree(arr_buffer); //--- reset error code ResetLastError(); //--- fill a part of the iBands array with values from the indicator buffer int copied=CopyBuffer(handle,buffer,start_pos,count,arr_buffer); if(copied!=count) { //--- if the copying fails, tell the error code PrintFormat("ERROR! EA: %s, FUNCTION: %s, amount to copy: %d, copied: %d, error code %d", __FILE__,__FUNCTION__,count,copied,GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } return(result); } //+------------------------------------------------------------------+
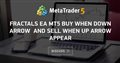
- 2022.06.03
- www.mql5.com
Don't forget: if there is no fractal, then the cell will be OR ' 0.0 ' OR ' EMPTY_VALUE '
thank you i have tried but the EA is not opening position when arrow appear
#include <Trade/Trade.mqh> //--- input parameters input int InputBars=9; //--- int handle_iFractals; // variable for storing the handle of the iFractals indicator bool m_init_error = false; // error on InInit ulong posTicket; CTrade trade; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- forced initialization of variables m_init_error = false; // error on InInit //--- create handle of the indicator iFractals handle_iFractals=iFractals(Symbol(),Period()); //--- if the handle is not created if(handle_iFractals==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iFractals indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early m_init_error=true; return(INIT_SUCCEEDED); } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { if(m_init_error) return; //--- //we calculate ask price double Ask=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits); //we calculate Bid price double Bid=NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_BID),_Digits); //We create empty string for the signal string signal=""; //create a price array MqlRates PriceArray[]; //Create fractals array double upper[],lower[]; ArraySetAsSeries(upper,true); ArraySetAsSeries(lower,true); // sort the array from the current candle downwards ArraySetAsSeries(PriceArray,true); //fill the array with price data //int Data=CopyRates(_Symbol,Period(),start_pos,count,PriceArray); int start_pos=0,count=6; //fill the array with price data int Data=CopyRates(_Symbol,Period(),start_pos,count,PriceArray); if(!iGetArray(handle_iFractals,UPPER_LINE,start_pos,count,upper) || !iGetArray(handle_iFractals,LOWER_LINE,start_pos,count,lower)) return; for(int i=0; i<count; i++){ if(upper[i]!=0.0 || upper[i]!=EMPTY_VALUE) {signal="sell"; }} for(int i=0; i<count; i++){ if(lower[i]!=0.0 || lower[i]!=EMPTY_VALUE) {signal="buy"; }} //sell 10 microlot if (signal=="sell"&&PositionsTotal()<1) trade.Buy(0.1,NULL,Bid,(Bid+200*_Point),(Bid-150*_Point),NULL); //BUY 10 microlot if (signal=="buy"&&PositionsTotal()<1) trade.Buy(0.1,NULL,Ask,(Ask-200*_Point),(Ask+150*_Point),NULL); //--- string text=""; for(int i=0; i<count; i++) { //--- string text_upper=""; if(upper[i]==0.0 || upper[i]==EMPTY_VALUE) text_upper=""; else text_upper=DoubleToString(upper[i],Digits()); //--- string text_lower=""; if(lower[i]==0.0 || lower[i]==EMPTY_VALUE) text_lower=""; else text_lower=DoubleToString(lower[i],Digits()); //--- text=text+IntegerToString(i)+"#: "+"Upper "+text_upper+", Lower "+text_lower+"\n"; } Comment(text); } //+------------------------------------------------------------------+ //| Get value of buffers | //+------------------------------------------------------------------+ bool iGetArray(const int handle,const int buffer,const int start_pos, const int count,double &arr_buffer[]) { bool result=true; if(!ArrayIsDynamic(arr_buffer)) { PrintFormat("ERROR! EA: %s, FUNCTION: %s, this a no dynamic array!",__FILE__,__FUNCTION__); return(false); } ArrayFree(arr_buffer); //--- reset error code ResetLastError(); //--- fill a part of the iBands array with values from the indicator buffer int copied=CopyBuffer(handle,buffer,start_pos,count,arr_buffer); if(copied!=count) { //--- if the copying fails, tell the error code PrintFormat("ERROR! EA: %s, FUNCTION: %s, amount to copy: %d, copied: %d, error code %d", __FILE__,__FUNCTION__,count,copied,GetLastError()); //--- quit with zero result - it means that the indicator is considered as not calculated return(false); } return(result); } //+------------------------------------------------------------------+
If there is a price, then you need this:
if(upper[i]!=0.0 && upper[i]!=EMPTY_VALUE)
thank you i have tried but the EA is not opening position when arrow appear
the above piece of code given by @vladmirkaruptov seems to work fine on my tester
#include <Trade/Trade.mqh> //--- input parameters input int InputBars=9; //--- int handle_iFractals; // variable for storing the handle of the iFractals indicator bool m_init_error = false; // error on InInit ulong posTicket; CTrade trade; //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- create handle of the indicator iFractals handle_iFractals=iFractals(Symbol(),Period()); //--- if the handle is not created if(handle_iFractals==INVALID_HANDLE) { //--- tell about the failure and output the error code PrintFormat("Failed to create handle of the iFractals indicator for the symbol %s/%s, error code %d", Symbol(), EnumToString(Period()), GetLastError()); //--- the indicator is stopped early return(INIT_FAILED); } //--- ChartIndicatorAdd(0,0,handle_iFractals); return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+maybe try to add the indicator to the chart and try to debug it more yourself
hi Vladimir Karputov this ea work well but i want it to open one trade at a time if is buy when hit tp it wait for another condition and then open trade and i want it to open trade even if the previous position still running please help me

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I have created EA for fractals indicator but when i run on strategy tester wont run is any one can help me
below is mql5 code for EA