Hi everyone, how can I get current open position with specific comment? when I use positionGetstring(position_comment), it includes closed order which i dont want. please help me, thank you!
In MQL5 you can't compare strings by '==' you have to use; https://www.mql5.com/en/docs/strings/stringcompare
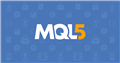
- www.mql5.com
I recommend learning the basics - so as not to confuse "order", "deal" and "position": Basic Principles
You didn't read the documentation well and you are using the functions for the NETTING account.
Here's how to do it:
//+------------------------------------------------------------------+ //| Test_en.mq5 | //| Copyright © 2022, Vladimir Karputov | //| https://www.mql5.com/en/users/barabashkakvn | //+------------------------------------------------------------------+ #property copyright "Copyright © 2022, Vladimir Karputov" #property link "https://www.mql5.com/en/users/barabashkakvn" #property version "1.000" #property script_show_inputs //--- #include <Trade\PositionInfo.mqh> CPositionInfo m_position; // trade position object //--- //+------------------------------------------------------------------+ //| Script program start function | //+------------------------------------------------------------------+ void OnStart() { } //+------------------------------------------------------------------+ //| Check Positions | //+------------------------------------------------------------------+ void CheckPositions() { for(int i=PositionsTotal()-1; i>=0; i--) if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties if(m_position.Symbol()==Symbol() /*&& m_position.Magic()==m_magic*/) { string comment=m_position.Commemt(); ulong ticket=m_position.Ticket(); if(m_position.PositionType()==POSITION_TYPE_BUY) { ... } else { ... } } } //+------------------------------------------------------------------+
In MQL5 you can't compare strings by '==' you have to use; https://www.mql5.com/en/docs/strings/stringcompare
thank you very much for your help
I recommend learning the basics - so as not to confuse "order", "deal" and "position": Basic Principles
You didn't read the documentation well and you are using the functions for the NETTING account.
Here's how to do it:
thank you very much for your help and information
ulong takeOrder=PositionGetTicket(i); if(PositionSelect(Symbol())==true)
You get a ticket from a random position (any chart) but then select a position on the current chart.

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi everyone, how can I get current open position with specific comment? when I use positionGetstring(position_comment), it includes closed order which i dont want. please help me, thank you!