1. Show your code.
2. No, you cannot.
Suminda Dharmasena :
If your account type is "Hedging Account", You have two options:
- OR close the position "BUY 0.01" and open the position "SELL 0.01"
- OR open "SELL 0.02" position and then back close
Vladimir Karputov #:
If your account type is "Hedging Account", You have two options:
- OR close the position "BUY 0.01" and open the position "SELL 0.01"
- OR open "SELL 0.02" position and then back close
How do I back close a position in option 2 in the above response?
A follow up question.
Say I have BUY 0.02 and I do a SELL 0.01.
- Can I have the same position ID?
- Can I partly back close the portion I sold?
- If I partly close will I get a new position ID?
Again thanks Vladimir and Dominik for the help.
Code:
//+------------------------------------------------------------------+ //| Buy using OrderSendAsync() asynchronous function | //+------------------------------------------------------------------+ bool BuyMarketAsync(const ulong position, const double volume, const double sl = 0, const double tp = 0, const ulong deviation = 10, const string comment = "Buy async", const ulong magic = 0) { if(position == 0 || !PositionSelectByTicket(position)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": cannot select ", position); return(false); } const string symbol = PositionGetString(POSITION_SYMBOL); //--- prepare the request MqlTradeRequest req = {}; req.action = TRADE_ACTION_DEAL; req.symbol = symbol; req.position = position; req.magic = magic; req.volume = volume; req.type = ORDER_TYPE_BUY; req.price = SymbolInfoDouble(req.symbol, SYMBOL_ASK); req.sl = sl; req.tp = tp; req.deviation = deviation; req.comment = comment; MqlTradeResult res = {}; if(!OrderSendAsync(req, res)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": error - ", ErrCode(GetLastError()), ", retcode = ", RetCode(res.retcode)); //--- then display the string description of the handled request Print("------------RequestDescription\r\n", RequestDescription(req, true), ":~"); //--- and show description of the request result Print("------------ ResultDescription\r\n", TradeResultDescription(res, true), ":~"); return(false); } //--- return(true); } //+------------------------------------------------------------------+ //| Buy using OrderSendAsync() asynchronous function | //+------------------------------------------------------------------+ bool BuyMarketAsync(const string symbol, const double volume, const double sl = 0, const double tp = 0, const ulong deviation = 10, const string comment = "Buy async", const ulong magic = 0) { ulong position; if(PositionSelect(symbol)) { position = PositionGetInteger(POSITION_TICKET); } else { position = 0; } //--- prepare the request MqlTradeRequest req = {}; req.action = TRADE_ACTION_DEAL; req.symbol = symbol; req.position = position; req.magic = magic; req.volume = volume; req.type = ORDER_TYPE_BUY; req.price = SymbolInfoDouble(req.symbol, SYMBOL_ASK); req.sl = sl; req.tp = tp; req.deviation = deviation; req.comment = comment; MqlTradeResult res = {}; if(!OrderSendAsync(req, res)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": error - ", ErrCode(GetLastError()), ", retcode = ", RetCode(res.retcode)); //--- then display the string description of the handled request Print("------------RequestDescription\r\n", RequestDescription(req, true), ":~"); //--- and show description of the request result Print("------------ ResultDescription\r\n", TradeResultDescription(res, true), ":~"); return(false); } //--- return(true); } //+------------------------------------------------------------------+ //| Sell using OrderSendAsync() asynchronous function | //+------------------------------------------------------------------+ bool SellMarketAsync(const ulong position, const double volume, const double sl = 0, const double tp = 0, const ulong deviation = 10, const string comment = "Sell async", const ulong magic = 0) { if(position == 0 || !PositionSelectByTicket(position)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": cannot select ", position); return(false); } const string symbol = PositionGetString(POSITION_SYMBOL); //--- prepare the request MqlTradeRequest req = {}; req.action = TRADE_ACTION_DEAL; req.symbol = symbol; req.position = position; req.magic = magic; req.volume = volume; req.type = ORDER_TYPE_SELL; req.price = SymbolInfoDouble(req.symbol, SYMBOL_BID); req.sl = sl; req.tp = tp; req.deviation = deviation; req.comment = comment; MqlTradeResult res = {}; if(!OrderSendAsync(req, res)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": error - ", ErrCode(GetLastError()), ", retcode = ", RetCode(res.retcode)); //--- then display the string description of the handled request Print("------------RequestDescription\r\n", RequestDescription(req, true), ":~"); //--- and show description of the request result Print("------------ ResultDescription\r\n", TradeResultDescription(res, true), ":~"); return(false); } //--- return(true); } //+------------------------------------------------------------------+ //| Sell using OrderSendAsync() asynchronous function | //+------------------------------------------------------------------+ bool SellMarketAsync(const string symbol, const double volume, const double sl = 0, const double tp = 0, const ulong deviation = 10, const string comment = "Sell async", const ulong magic = 0) { ulong position; if(PositionSelect(symbol)) { position = PositionGetInteger(POSITION_TICKET); } else { position = 0; } //--- prepare the request MqlTradeRequest req = {}; req.action = TRADE_ACTION_DEAL; req.symbol = symbol; req.position = position; req.magic = magic; req.volume = volume; req.type = ORDER_TYPE_SELL; req.price = SymbolInfoDouble(req.symbol, SYMBOL_BID); req.sl = sl; req.tp = tp; req.deviation = deviation; req.comment = comment; MqlTradeResult res = {}; if(!OrderSendAsync(req, res)) { Print(__FILE__, ":", __LINE__, " | ", __FUNCSIG__, ": error - ", ErrCode(GetLastError()), ", retcode = ", RetCode(res.retcode)); //--- then display the string description of the handled request Print("------------RequestDescription\r\n", RequestDescription(req, true), ":~"); //--- and show description of the request result Print("------------ ResultDescription\r\n", TradeResultDescription(res, true), ":~"); return(false); } //--- return(true); }
Suminda Dharmasena # :
How do I back close a position in option 2 in the above response?
A follow up question.
Say I have BUY 0.02 and I do a SELL 0.01.
- Can I have the same position ID?
- Can I partly back close the portion I sold?
- If I partly close will I get a new position ID?
I am using CTrade trading class, PositionCloseBy method:
Closes a position with the specified ticket by an opposite position.
bool PositionCloseBy( const ulong ticket, // position ticket const ulong ticket_by // opposite position ticket )
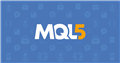
Documentation on MQL5: Standard Library / Trade Classes / CTrade / PositionCloseBy
- www.mql5.com
PositionCloseBy(const ulong,const ulong) - CTrade - Trade Classes - Standard Library - MQL5 Reference - Reference on algorithmic/automated trading language for MetaTrader 5

You are missing trading opportunities:
- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
Registration
Log in
You agree to website policy and terms of use
If you do not have an account, please register
Say I have a long position of 0.01 which I want to reverse hence I sell 0.02.
I sent the an opposite order with the position ID so as to have the reversed position also to have the same ID.
I get invalid volume error.