Hi, is there a way to check if the symbol was already selected for use with CSymbolInfo methods ?
The question is not entirely clear - if you are working with the CSymbolInfo class, then you have an object (usually m_symbol) that is initialized, which means that the symbol has already been selected.
Add:
Correct initialization:
//+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { *** //--- ResetLastError(); if(!m_symbol.Name(Symbol())) // sets symbol name { Print(__FILE__," ",__FUNCTION__,", ERROR: CSymbolInfo.Name"); return(INIT_FAILED); } *** //--- return(INIT_SUCCEEDED); }
an example is in the file 'Trading engine 4.mq5' from the article 'An attempt at developing an EA constructor'
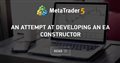
- www.mql5.com
The question is not entirely clear - if you are working with the CSymbolInfo class, then you have an object (usually m_symbol) that is initialized, which means that the symbol has already been selected.
Add:
Correct initialization:
an example is in the file 'Trading engine 4.mq5' from the article 'An attempt at developing an EA constructor'
Hi Vladimir, thanks for replying.
I know the correct initialization requires to select the Symbol but I want to programmatically ensure this has been done in the EA code in case it's been for any reason forgotten.
I don't think the documentation shows your example anywhere (thanks for sharing it here though) and it still doesn't resolve the human error where the programmer forgets to select the symbol... I've got a class that inherits from CSymbolInfo and I want to make sure that symbol has been selected and terminate the EA if not.
Hi Vladimir, thanks for replying.
I know the correct initialization requires to select the Symbol but I want to programmatically ensure this has been done in the EA code in case it's been for any reason forgotten.
I don't think the documentation shows your example anywhere (thanks for sharing it here though) and it still doesn't resolve the human error where the programmer forgets to select the symbol... I've got a class that inherits from CSymbolInfo and I want to make sure that symbol has been selected and terminate the EA if not.
You just need to check what the method returns
m_symbol.Name()
Just do an experiment. Write the simplest code. If you share the result - I will be grateful to you.
Hi Vladimir, yeah you are right... I haven't noticed Name() method is overloaded... I've run below code and it is working as expected (returns empty value before symbol is set.
Thanks for pointing me in the right direction.
#include <Trade\SymbolInfo.mqh> CSymbolInfo SymbolInfo; //--- void OnStart() { Print( SymbolInfo.Name() ); Print( SymbolInfo.Name(_Symbol) ); Print( SymbolInfo.Name() ); }
console: 2022.05.03 17:55:40.661 Test2 (AUDUSD.a,H1) 2022.05.03 17:55:40.661 Test2 (AUDUSD.a,H1) true 2022.05.03 17:55:40.661 Test2 (AUDUSD.a,H1) AUDUSD.a
By the way any ideas why SymbolInfo.Ask() and SymbolInfo.Bid() both return 0.0?
The SymbolInfoDouble( _Symbol, SYMBOL_BID ) and SymbolInfoDouble( _Symbol, SYMBOL_ASK ) return correct values
#include <Trade\SymbolInfo.mqh> CSymbolInfo SymbolInfo; //--- void OnStart() { Print( SymbolInfo.Name() ); Print( SymbolInfo.Name(_Symbol) ); Print( SymbolInfo.Name() ); Print( SymbolInfo.Ask() ); Print( SymbolInfo.Bid() ); Print( SymbolInfoDouble( _Symbol, SYMBOL_ASK ) ); Print( SymbolInfoDouble( _Symbol, SYMBOL_BID ) ); }
console: 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) true 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) AUDUSD.a 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) 0.0 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) 0.0 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) 0.71133 2022.05.03 18:12:12.679 Test2 (AUDUSD.a,H1) 0.71131
By the way any ideas why SymbolInfo.Ask() and SymbolInfo.Bid() both return 0.0?
The SymbolInfoDouble( _Symbol, SYMBOL_BID ) and SymbolInfoDouble( _Symbol, SYMBOL_ASK ) return correct values
Need to update prices. I am using this function:
//+------------------------------------------------------------------+ //| Refreshes the symbol quotes data | //+------------------------------------------------------------------+ bool RefreshRates() { //--- refresh rates if(!m_symbol.RefreshRates()) { Print(__FILE__," ",__FUNCTION__,", ERROR: ","RefreshRates error"); return(false); } //--- protection against the return value of "zero" if(m_symbol.Ask()==0 || m_symbol.Bid()==0) { Print(__FILE__," ",__FUNCTION__,", ERROR: ","Ask == 0.0 OR Bid == 0.0"); return(false); } //--- return(true); }
I recommend reading my article: An attempt at developing an EA constructor in the article there is a description of the trading engine ' Trading engine 4 '.
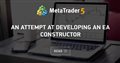
- www.mql5.com
I recommend reading my article: An attempt at developing an EA constructor in the article there is a description of the trading engine ' Trading engine 4 '.
It's already on my reading list :) looks like a great resource!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use