Why do you have OnStart() AND OnTick()?
Is this supposed to be an EA or a script?
- You are mixing the concepts of a Script (OnStart) and that of an Expert Advisor (OnTick).
- You are mixing concepts from MQL4 and MQL5:
- MQL5 does not have the the predefined array variables Open[], High[], Low[], Close[]. Those are only available in MQL4. Use the methods as described in the MQL5 documentation.
- MQL5 does not have the the predefined variables Ask or Bid (see below).
- Indicator handles should first be obtained in the OnInit() event handler, not in the other event handlers.
- Price quotes returned by the terminal are already normalised. Don't normalise them again.
For calculated price quotes, normalise prices based on the tick size. Don't normalise based on Points or number of Digits (see below). - When scanning through the currently open positions, you need to Select the position first before obtaining its properties. So use the function PositionGetSymbol() to obtain it's symbol and select the position by index as well, for further processing. Or use the class CTrade functionality from the Trade Classes in the Standard Library if you prefer Object Oriented Programming.
Forum on trading, automated trading systems and testing trading strategies
Fernando Carreiro, 2022.04.23 14:09
Unlike MQL4, MQL5 does not have the predefined variables "Ask" and "Bid". So, you will have to assign them yourself, either by using the SymbolInfoDouble() function or by using the SymbolInfoTick() function.
Example using SymbolInfoDouble() function (sample code is untested/uncompiled):
double Ask = SymbolInfoDouble( _Symbol, SYMBOL_ASK ), // Get symbol's current Ask price Bid = SymbolInfoDouble( _Symbol, SYMBOL_BID ); // Get symbol's current Bid priceExample using SymbolInfoTick() function (sample code is untested/uncompiled):
MqlTick oTick; // Declare variable of MqlTick structure if( SymbolInfoTick( _Symbol, oTick ) ) { double Ask = oTick.ask, // Get symbol's current Ask price or just use "oTick.ask" directly in your code instead of "Ask" Bid = oTick.bid; // Get symbol's current Bid price or just use "oTick.bid" directly in your code instead of "Bid" // Rest of your code goes here ... };If you want to mimic the MQL4 style of predefined variables, then you can use one the following examples (sample code is untested/uncompiled):
double Ask, Bid; // Globally scoped Ask and Bid prices void OnTick() { Ask = SymbolInfoDouble( _Symbol, SYMBOL_ASK ), // Get symbol's current Ask price Bid = SymbolInfoDouble( _Symbol, SYMBOL_BID ); // Get symbol's current Bid price // Rest of your code goes here };double Ask, Bid; // Globally scoped Ask and Bid prices void OnTick() { MqlTick oTick; // Declare variable of MqlTick structure if( SymbolInfoTick( _Symbol, oTick ) ) { Ask = oTick.ask, // Get symbol's current Ask price Bid = oTick.bid; // Get symbol's current Bid price // Rest of your code goes here ... }; };Forum on trading, automated trading systems and testing trading strategies
Tick size vs Point(), can be a little tricky in Multicurrency EA
Fernando Carreiro, 2022.03.09 12:11
Tick Size and Point Size can be very different especially on stocks and other symbols besides forex.
Always use Tick Size to adjust and align your prices, not the point size. In essence, make sure that your price quotes, are properly aligned to the Tick size (see following examples).
... double tickSize = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_SIZE ); ... double normalised_price = round( price / tick_size ) * tick_size; ... // Or use a function double Round2Ticksize( double price ) { double tick_size = SymbolInfoDouble( _Symbol, SYMBOL_TRADE_TICK_SIZE ); return( round( price / tick_size ) * tick_size ); };
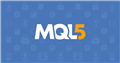
- www.mql5.com
- You are mixing the concepts of a Script (OnStart) and that of an Expert Advisor (OnTick).
- You are mixing concepts from MQL4 and MQL5:
- MQL5 does not have the the predefined array variables Open[], High[], Low[], Close[]. Those are only available in MQL4. Use the methods as described in the MQL5 documentation.
- MQL5 does not have the the predefined variables Ask or Bid (see below).
- Indicator handles should first be obtained in the OnInit() event handler, not in the other event handlers.
- Price quotes returned by the terminal are already normalised. Don't normalise them again.
For calculated price quotes, normalise prices based on the tick size. Don't normalise based on Points or number of Digits (see below). - When scanning through the currently open positions, you need to Select the position first before obtaining its properties. So use the function PositionGetSymbol() to obtain it's symbol and select the position by index as well, for further processing. Or use the class CTrade functionality from the Trade Classes in the Standard Library if you prefer Object Oriented Programming.
Thanks alot, I really appreciate your contribution. I will send the Code as soon as am done with
- You are mixing the concepts of a Script (OnStart) and that of an Expert Advisor (OnTick).
- You are mixing concepts from MQL4 and MQL5:
- MQL5 does not have the the predefined array variables Open[], High[], Low[], Close[]. Those are only available in MQL4. Use the methods as described in the MQL5 documentation.
- MQL5 does not have the the predefined variables Ask or Bid (see below).
- Indicator handles should first be obtained in the OnInit() event handler, not in the other event handlers.
- Price quotes returned by the terminal are already normalised. Don't normalise them again.
For calculated price quotes, normalise prices based on the tick size. Don't normalise based on Points or number of Digits (see below). - When scanning through the currently open positions, you need to Select the position first before obtaining its properties. So use the function PositionGetSymbol() to obtain it's symbol and select the position by index as well, for further processing. Or use the class CTrade functionality from the Trade Classes in the Standard Library if you prefer Object Oriented Programming.
Thanks to y'all am able to compile the code successfully, thanks alot. But I can't get to use the ticks instead

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use