StringConcatenate works differently in MQL5 than in MQL4.
In MQL4, it returns the string formed by concatenation.
In MQL5, the first string variable you insert is what is used to form the concatenation with the other two (or more) string values you put as arguments.
string a = "Hello "; string b = "World"; string c; StringConcatenate(c, a, b); Print(c); // Hello World
MQL5 Docs -> StringConcatenate: https://www.mql5.com/en/docs/strings/stringconcatenate
IMO it was a mistake to by the devs to change it because it breaks code and things like that are part of the reason why MQL5 wasn't received well by the community when it was first released.
One way to make your code compatible between MQL4 and MQL5 is to use an #ifdef statement
string sendInformation; #ifdef __MQL4__ sendInformation = StringConcatenate(textString,CurrentAccountBalance); #else StringConcatenate(sendInformation, textString, CurrentAccountBalance); #endif
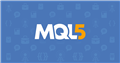
- www.mql5.com
Corrected version working with mt5 :
string CurrentAccountBalance = DoubleToString(AccountInfoDouble(ACCOUNT_BALANCE)); string textString = "Account balance = $"; string sendInformation; StringConcatenate(sendInformation,textString,CurrentAccountBalance); SendNotification(sendInformation);
StringConcatenate works differently in MQL5 than in MQL4.
In MQL4, it returns the string formed by concatenation.
In MQL5, the first string variable you insert is what is used to form the concatenation with the other two (or more) string values you put as arguments.
MQL5 Docs -> StringConcatenate: https://www.mql5.com/en/docs/strings/stringconcatenate
IMO it was a mistake to by the devs to change it because it breaks code and things like that are part of the reason why MQL5 wasn't received well by the community when it was first released.
One way to make your code compatible between MQL4 and MQL5 is to use an #ifdef statement
Hi Alexander
Thank you for your example - it now makes perfect sense. Your example (hello world) should be posted into the documentation - very clear.
And yes I do agree with you that the implementation of MQL5 is foreign to other operating system upgrades. Usually each revision builds on the previous versions - it does not replace or re-write methods and force the developer to re-learn.
Thanks again.
Hi
I'm having issues with using StringConcatenate within MT5. I am converting MT4 code to MT5 and have come across something that I would of thought would be simple - but after several attempts at solving it - seems to be a bit of an issue for me.
I have a bit of code that creates a string and then sends that off as a notification. SendNotification likes a straight string - nothing fancy.
MetaTrader 4 that works fine -
MetaTrader 5 that does not work
The error I get is -
'StringConcatenate' - wrong parameters count
implicit conversion from 'number' to 'string'
I'm not smart enough to know why a method designed to join two strings together returns an integer.
Does anyone have an example of the use of the StringConcatenate method within MT5 - or point me in the direction of some code. All the examples I can find are MT4.
Thanks
Put
(str)
In front any function working with number that you want to output as a string in the stringcon ... function
That is
string CurrentAccountBalance = (str)DoubleToString(AccountInfoDouble(ACCOUNT_BALANCE));
Hi Alexander
Thank you for your example - it now makes perfect sense. Your example (hello world) should be posted into the documentation - very clear.
And yes I do agree with you that the implementation of MQL5 is foreign to other operating system upgrades. Usually each revision builds on the previous versions - it does not replace or re-write methods and force the developer to re-learn.
Thanks again.
string a = "Hello "; string b = "World"; string c = a + b; // StringConcatenate(c, a, b); Print(c); // Hello World
Put
In front any function working with number that you want to output as a string in the stringcon ... function
That is
When I add this line into my MT5 -
string CurrentAccountBalance = (str)DoubleToString(AccountInfoDouble(ACCOUNT_BALANCE));
I get the following error
'str' - undeclared identifier
'DoubleToString' - some operator expected

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi
I'm having issues with using StringConcatenate within MT5. I am converting MT4 code to MT5 and have come across something that I would of thought would be simple - but after several attempts at solving it - seems to be a bit of an issue for me.
I have a bit of code that creates a string and then sends that off as a notification. SendNotification likes a straight string - nothing fancy.
MetaTrader 4 that works fine -
MetaTrader 5 that does not work
The error I get is -
'StringConcatenate' - wrong parameters count
implicit conversion from 'number' to 'string'
I'm not smart enough to know why a method designed to join two strings together returns an integer.
Does anyone have an example of the use of the StringConcatenate method within MT5 - or point me in the direction of some code. All the examples I can find are MT4.
Thanks