ok I just did
Rand=(MathRand()*100)/32767
That will give me a random number from 0 to 100. But an integer not a double.
So do it twice for the integer and fraction parts
Double=Int+Fraction/100
Here you go:
//+------------------------------------------------------------------+ //| Random value in the range [min, max] | //| Generates random numbers with a uniform distribution (bias-free).| //| Maximum granularity of generator is 32768 values. | //+------------------------------------------------------------------+ template <typename T> T random(const T min, const T max) { return((T)((double)min + (((double)MathRand() / 32767.0) * (double)(max - min)))); }
Best regards
Or just use (for 0 to 1 doubles)
double d=MathMod(MathRand(),100)/100;
Or just use (for 0 to 1 doubles)
You know this will limit your values to a max of 100.
So the granularity of your random number is 0.01. Starting at 0.00, going in steps of 0.01 to 0.99.
Just saying.
You know this will limit your values to a max of 100.
So the granularity of your random number is 0.01. Starting at 0.00, going in steps of 0.01 to 0.99.
Just saying.
It is exactly as the OP requested - double numbers 0.00 to 0.99
Actually - if 1 is included in the desired scope than should be changed to
double d=MathMod(MathRand(),101)/100;
It is exactly as the OP requested - double numbers 0.00 to 0.99
Actually - if 1 is included in the desired scope than should be changed to
Although your first answer is not >exactly< meeting the request, I like the idea of specifying the granularity.
Therefore I integrated it into the function as shown here:
//+------------------------------------------------------------------+ //| Random value in the range [min, max] | //| Generates random numbers with a uniform distribution (bias-free).| //| Granularity of generator is at max 32768 values. | //+------------------------------------------------------------------+ template <typename T> T random(const T min, const T max, const double granularity = 32768.0) { return((T)((double)min + ((MathMod(MathRand(), granularity) / (granularity - 1.0) * (double)(max - min)))); }
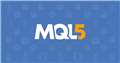
- www.mql5.com
int Zufallszahl() { int Zufall = -1; int BoS = MathRand() % 2; // Zufallszahl für Zufallsorder mit 0 und 1 auswählen if(BoS ==0) LongTrade(true,_Symbol,0.01); else ShortTrade(true,_Symbol,0.01); return(BoS); } //+----------------------------------------

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
I need a number from 0.00 to 1.00 completly random, is that too much to ask?
MathRand() is not working for me, please help.