Basket=StringToDouble("InputBasket");
https://www.mql5.com/en/docs/convert/stringtodouble
String containing a symbol representation of a number.
"InputBasket"
NAN = Not a Number.
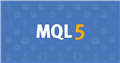
- www.mql5.com
https://www.mql5.com/en/docs/convert/stringtodouble
String containing a symbol representation of a number.
NAN = Not a Number.
Not sure what you are saying..
Basket is a double variable.
I assume that the number entered in the box is text, that is why i am putting stringtodouble.
If that is incorrect, what should I be putting in the code: and how do I pull it into the Basket variable?
thanks
ObjectSetDouble(0,Basket,OBJPROP_TEXT,"InputBasket"); ?
Please read the documentation.
Your string has to hold a double value for example "10.0" and not "InputBasket" that is not a number and can not be converted to a number.
Example:
string s = "10.0"; double d = StringToDouble(s); Print(d);
ObjectSetDouble(0,"InputBasket",OBJPROP_TEXT,Basket);
This is wrong because this function set's a double not a text so use
ObjectSetString( for OBJPROP_TEXT
ObjectSetString(0,"InputBasket",OBJPROP_TEXT,"12345");
Otherwise i would advice you to use the standard functions,
//+------------------------------------------------------------------+ //| Create Edit object | //+------------------------------------------------------------------+ bool EditCreate(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int sub_window=0, // subwindow index const int x=0, // X coordinate const int y=0, // Y coordinate const int width=50, // width const int height=18, // height const string text="Text", // text const string font="Arial", // font const int font_size=10, // font size const ENUM_ALIGN_MODE align=ALIGN_CENTER, // alignment type const bool read_only=false, // ability to edit const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER, // chart corner for anchoring const color clr=clrBlack, // text color const color back_clr=clrWhite, // background color const color border_clr=clrNONE, // border color const bool back=false, // in the background const bool selection=false, // highlight to move const bool hidden=true, // hidden in the object list const long z_order=0) // priority for mouse click { //--- reset the error value ResetLastError(); //--- create edit field if(!ObjectCreate(chart_ID,name,OBJ_EDIT,sub_window,0,0)) { Print(__FUNCTION__, ": failed to create \"Edit\" object! Error code = ",GetLastError()); return(false); } //--- set object coordinates ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x); ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y); //--- set object size ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width); ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height); //--- set the text ObjectSetString(chart_ID,name,OBJPROP_TEXT,text); //--- set text font ObjectSetString(chart_ID,name,OBJPROP_FONT,font); //--- set font size ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size); //--- set the type of text alignment in the object ObjectSetInteger(chart_ID,name,OBJPROP_ALIGN,align); //--- enable (true) or cancel (false) read-only mode ObjectSetInteger(chart_ID,name,OBJPROP_READONLY,read_only); //--- set the chart's corner, relative to which object coordinates are defined ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,corner); //--- set text color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set background color ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr); //--- set border color ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_COLOR,border_clr); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the label by mouse ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Move Edit object | //+------------------------------------------------------------------+ bool EditMove(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int x=0, // X coordinate const int y=0) // Y coordinate { //--- reset the error value ResetLastError(); //--- move the object if(!ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x)) { Print(__FUNCTION__, ": failed to move X coordinate of the object! Error code = ",GetLastError()); return(false); } if(!ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y)) { Print(__FUNCTION__, ": failed to move Y coordinate of the object! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Resize Edit object | //+------------------------------------------------------------------+ bool EditChangeSize(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int width=0, // width const int height=0) // height { //--- reset the error value ResetLastError(); //--- change the object size if(!ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width)) { Print(__FUNCTION__, ": failed to change the object width! Error code = ",GetLastError()); return(false); } if(!ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height)) { Print(__FUNCTION__, ": failed to change the object height! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Change Edit object's text | //+------------------------------------------------------------------+ bool EditTextChange(const long chart_ID=0, // chart's ID const string name="Edit", // object name const string text="Text") // text { //--- reset the error value ResetLastError(); //--- change object text if(!ObjectSetString(chart_ID,name,OBJPROP_TEXT,text)) { Print(__FUNCTION__, ": failed to change the text! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Return Edit object text | //+------------------------------------------------------------------+ bool EditTextGet(string &text, // text const long chart_ID=0, // chart's ID const string name="Edit") // object name { //--- reset the error value ResetLastError(); //--- get object text if(!ObjectGetString(chart_ID,name,OBJPROP_TEXT,0,text)) { Print(__FUNCTION__, ": failed to get the text! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Delete Edit object | //+------------------------------------------------------------------+ bool EditDelete(const long chart_ID=0, // chart's ID const string name="Edit") // object name { //--- reset the error value ResetLastError(); //--- delete the label if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete \"Edit\" object! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+
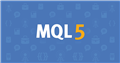
- www.mql5.com
Please read the documentation.
Your string has to hold a double value for example "10.0" and not "InputBasket" that is not a number and can not be converted to a number.
Example:
This is wrong because this function set's a double not a text so use
Otherwise i would advice you to use the standard functions,
HI Marco,
thanks for your input, I used your example of the standard function, which helps,
But now I can't get the input to show..
I added the following under the first section after return(true)
EditCreate(0,"Edit",0,x,y,width,height,"Text",font,font_size,align,read_only,corner,clr,back_clr,border_clr,back,selection,hidden,z_order);
Nothing shows on the chart.
I did manage to get it up once, but now it doesn't work again, also It was stuck in the very corner, which I couldn't see it.. I changed the figures at the top eg x & y, but it wouldn't move.
I have looked at the links you sent me, but I can't find anything that looks like the above.
I don't get any errors, so I'm not sure what coding I have wrong..
Thanks in advance for all your help :)
First off you have to check the object list to see if it exists.
Also you have to remove it first if you want to recreate it.
In your case you could try the following.
if(ObjectFind("Edit") < 0) { EditCreate(0,"Edit",0,200,200,200,200,"Text"); }
Or for fast development.
ObjectDelete("Edit"); EditCreate(0,"Edit",0,200,200,200,200,"Text");
If you are trying to recreate an object that already exists it will throw 4200 Object already exists.
EditCreate(0,"Edit",0,200,200,200,200,"Text"); EditCreate(0,"Edit",0,200,200,200,200,"Text"); Print(GetLastError());
First off you have to check the object list to see if it exists.
Also you have to remove it first if you want to recreate it.
In your case you could try the following.
Or for fast development.
If you are trying to recreate an object that already exists it will throw 4200 Object already exists.
HI Marco,
I am going completely crazy,
I updated the EA as you suggested above. And the button shows up, but what I need it so be able to pull the number that I put in the box to my variable.
I have entered the following in the:
OnInit, OnChartEvent, & in the EditCreate, EditTextChange & EditTextGet (just above the return(true);
void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { if (id == CHARTEVENT_OBJECT_ENDEDIT && sparam==name_inputbutton) { { ObjectDelete("Edit"); EditCreate(0,"Edit",0,100,100,100,100,"Enter Basket"); string s = "Edit"; double d = StringToDouble(s); basket=d; }} }
I can change the number in the box, but it doesn't transfer to the variable basket.
I even tried the following, thinking that the EditTextGet would pull the figure that I entered in the box.
The number in the box gets updated and the object, but it doesn't change the basket variable
if (id == CHARTEVENT_OBJECT_ENDEDIT && sparam==name_inputbutton) { { ObjectDelete("Edit"); EditCreate(0,"Edit",0,100,100,100,100,"Enter Basket"); string s; EditTextGet(s,0,"Edit"); double d = StringToDouble(s); basket=d; }}
Any suggestions???
That is also in those standard calls that i posted above.
//+------------------------------------------------------------------+ //| Return Edit object text | //+------------------------------------------------------------------+ bool EditTextGet(string &text, // text const long chart_ID=0, // chart's ID const string name="Edit") // object name { //--- reset the error value ResetLastError(); //--- get object text if(!ObjectGetString(chart_ID,name,OBJPROP_TEXT,0,text)) { Print(__FUNCTION__, ": failed to get the text! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+
And then you have to set the string on the basket object.
Don't forget to call ChartRedraw(); when you are done.
And try to post all of the relevant code because i don't see any basket.
I don't like guessing games.
if (id == CHARTEVENT_OBJECT_ENDEDIT && sparam==name_inputbutton) { { ObjectDelete("Edit"); EditCreate(0,"Edit",0,100,100,100,100,"Enter Basket"); string s; EditTextGet(s,0,"Edit"); ObjectSetString(0,"Basket",OBJPROP_TEXT,s); ChartRedraw(); }}
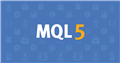
- www.mql5.com
Hi Marco,
thanks for your help.
I tried a few other option as per your suggestion. still no luck
I have attached a screen shot of the results, as you can see the object is created "Edit" and when I update the number in the box, it transfers to the Object list fine.
I have included the complete indicator coding.
(this is just the button.. I want to get this working before I try and bring the button into any other indicator..
Thanks again for you help. :)
//+------------------------------------------------------------------+ //| buttoninput.mq4 | //| Copyright 2019, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2019, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" #property strict #property indicator_chart_window double basket=10; string name_inputbutton = "basket"; string s="nul"; double d; //+------------------------------------------------------------------+ //| Custom indicator initialization function | //+------------------------------------------------------------------+ int OnInit() { ObjectDelete("Edit"); EditCreate(0,"Edit",0,100,100,100,100,"Enter Basket"); ChartRedraw(); text(); //--- return(INIT_SUCCEEDED); } double ObjectGetDouble( long chart_id, // chart identifier string name, // object name ENUM_OBJECT_PROPERTY_DOUBLE prop_id, // property identifier int prop_modifier=0 // property modifier, if required ); //+------------------------------------------------------------------+ //| Custom indicator Text | //+------------------------------------------------------------------+ void stats(string tname,string word,int fsize,string ftype,color tcolor,int posxy,int posx,int posy,int cnr) { // TextSetFont( tname,20, FW_BOLD,1 ); ObjectCreate(tname,OBJ_LABEL,0,0,0); ObjectSetText(tname,word,fsize,ftype,tcolor); ObjectSet(tname,OBJPROP_CORNER,1); ObjectSet(tname,OBJPROP_XDISTANCE,posx); ObjectSet(tname,OBJPROP_YDISTANCE,posy); } void text() { string Mode=" "; int ipos=200; int fontS=12; double vbid = MarketInfo(Symbol(), MODE_SPREAD); color InfoColor=clrWhite; int xpos=200;//Text Position int cnr=1; int row=30; string Font="Arial"; stats("TXT","Basket: "+DoubleToString(basket,0),fontS,Font,clrAqua,ipos,xpos-1,row,cnr); stats("TXT1","s="+s+" d="+DoubleToString(d,0),fontS,Font,clrAqua,ipos,xpos-1,row+20,cnr); } //+------------------------------------------------------------------+ //| Custom indicator iteration function | //+------------------------------------------------------------------+ int OnCalculate (const int rates_total, // size of input time series const int prev_calculated, // bars handled in previous call const datetime& time[], // Time const double& open[], // Open const double& high[], // High const double& low[], // Low const double& close[], // Close const long& tick_volume[], // Tick Volume const long& volume[], // Real Volume const int& spread[] // Spread ) { //--- //--- return value of prev_calculated for next call return(rates_total); } //+------------------------------------------------------------------+ //| Timer function | //+------------------------------------------------------------------+ void OnTimer() { //--- //text(); } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { if (id == CHARTEVENT_OBJECT_ENDEDIT && sparam==name_inputbutton) { // ObjectDelete("Edit"); // EditCreate(0,"Edit",0,100,100,100,100,"Enter Basket"); EditTextGet(s,0,"Edit"); // ObjectGet("Edit",OBJ_EDIT); // ObjectSetString(0,"Basketinput",OBJPROP_NAME,"Edit"); ChartRedraw(); // text(); } } bool IsNumeric(string text) { int length=StringLen(text); for(int i=0;i<length;i++) { int char1=StringGetChar(text,i); if((char1>47 && char1<58) || char1==46) continue; else return(false); } return(true); } //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //+------------------------------------------------------------------+ //| Create Edit object | //+------------------------------------------------------------------+ bool EditCreate(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int sub_window=0, // subwindow index const int x=100, // X coordinate const int y=100, // Y coordinate const int width=50, // width const int height=25, // height const string text="Text", // text const string font="Arial", // font const int font_size=10, // font size const ENUM_ALIGN_MODE align=ALIGN_CENTER, // alignment type const bool read_only=false, // ability to edit const ENUM_BASE_CORNER corner=CORNER_LEFT_UPPER, // chart corner for anchoring const color clr=clrBlack, // text color const color back_clr=clrWhite, // background color const color border_clr=clrNONE, // border color const bool back=false, // in the background const bool selection=false, // highlight to move const bool hidden=false, // hidden in the object list const long z_order=0) // priority for mouse click { //--- reset the error value ResetLastError(); //--- create edit field if(!ObjectCreate(chart_ID,name,OBJ_EDIT,sub_window,0,0)) { Print(__FUNCTION__, ": failed to create \"Edit\" object! Error code = ",GetLastError()); return(false); } //--- set object coordinates ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x); ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y); //--- set object size ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width); ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height); //--- set the text ObjectSetString(chart_ID,name,OBJPROP_TEXT,text); //--- set text font ObjectSetString(chart_ID,name,OBJPROP_FONT,font); //--- set font size ObjectSetInteger(chart_ID,name,OBJPROP_FONTSIZE,font_size); //--- set the type of text alignment in the object ObjectSetInteger(chart_ID,name,OBJPROP_ALIGN,align); //--- enable (true) or cancel (false) read-only mode ObjectSetInteger(chart_ID,name,OBJPROP_READONLY,read_only); //--- set the chart's corner, relative to which object coordinates are defined ObjectSetInteger(chart_ID,name,OBJPROP_CORNER,corner); //--- set text color ObjectSetInteger(chart_ID,name,OBJPROP_COLOR,clr); //--- set background color ObjectSetInteger(chart_ID,name,OBJPROP_BGCOLOR,back_clr); //--- set border color ObjectSetInteger(chart_ID,name,OBJPROP_BORDER_COLOR,border_clr); //--- display in the foreground (false) or background (true) ObjectSetInteger(chart_ID,name,OBJPROP_BACK,back); //--- enable (true) or disable (false) the mode of moving the label by mouse ObjectSetInteger(chart_ID,name,OBJPROP_SELECTABLE,selection); ObjectSetInteger(chart_ID,name,OBJPROP_SELECTED,selection); //--- hide (true) or display (false) graphical object name in the object list ObjectSetInteger(chart_ID,name,OBJPROP_HIDDEN,hidden); //--- set the priority for receiving the event of a mouse click in the chart ObjectSetInteger(chart_ID,name,OBJPROP_ZORDER,z_order); //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Move Edit object | //+------------------------------------------------------------------+ bool EditMove(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int x=0, // X coordinate const int y=0) // Y coordinate { //--- reset the error value ResetLastError(); //--- move the object if(!ObjectSetInteger(chart_ID,name,OBJPROP_XDISTANCE,x)) { Print(__FUNCTION__, ": failed to move X coordinate of the object! Error code = ",GetLastError()); return(false); } if(!ObjectSetInteger(chart_ID,name,OBJPROP_YDISTANCE,y)) { Print(__FUNCTION__, ": failed to move Y coordinate of the object! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Resize Edit object | //+------------------------------------------------------------------+ bool EditChangeSize(const long chart_ID=0, // chart's ID const string name="Edit", // object name const int width=0, // width const int height=0) // height { //--- reset the error value ResetLastError(); //--- change the object size if(!ObjectSetInteger(chart_ID,name,OBJPROP_XSIZE,width)) { Print(__FUNCTION__, ": failed to change the object width! Error code = ",GetLastError()); return(false); } if(!ObjectSetInteger(chart_ID,name,OBJPROP_YSIZE,height)) { Print(__FUNCTION__, ": failed to change the object height! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); } //+------------------------------------------------------------------+ //| Change Edit object's text | //+------------------------------------------------------------------+ bool EditTextChange(const long chart_ID=0, // chart's ID const string name="Edit", // object name const string text="Text") // text { //--- reset the error value ResetLastError(); //--- change object text if(!ObjectSetString(chart_ID,name,OBJPROP_TEXT,text)) { Print(__FUNCTION__, ": failed to change the text! Error code = ",GetLastError()); return(false); } s=ObjectGetString(0,"Edit",OBJPROP_NAME,0);// I also tried OBJPROP_NAME d=StringToDouble(s); basket=d; return(true); } //+------------------------------------------------------------------+ //| Return Edit object text | //+------------------------------------------------------------------+ bool EditTextGet(string &text, // text const long chart_ID=0, // chart's ID const string name="Edit") // object name { //--- reset the error value ResetLastError(); //--- get object text if(!ObjectGetString(chart_ID,name,OBJPROP_TEXT,0,text)) { Print(__FUNCTION__, ": failed to get the text! Error code = ",GetLastError()); return(false); } s=ObjectGetString(0,name,OBJPROP_NAME,0);// I also tried OBJPROP_NAME & OBJPROP_TEXT d=StringToDouble(s); basket=d; return(true); } //+------------------------------------------------------------------+ //| Delete Edit object | //+------------------------------------------------------------------+ bool EditDelete(const long chart_ID=0, // chart's ID const string name="Edit") // object name { //--- reset the error value ResetLastError(); //--- delete the label if(!ObjectDelete(chart_ID,name)) { Print(__FUNCTION__, ": failed to delete \"Edit\" object! Error code = ",GetLastError()); return(false); } //--- successful execution return(true); }
Okay so you need multiple conversions if you want to use the numerical value.
if (id == CHARTEVENT_OBJECT_ENDEDIT && sparam==name_inputbutton) { double d = StringToDouble(ObjectGetString(0,"Edit",OBJPROP_TEXT)); ObjectSetString(0,"TXT1",OBJPROP_TEXT,(string)d); ChartRedraw(); }
Otherwise you could just use the string values directly.
Okay so you need multiple conversions if you want to use the numerical value.
Otherwise you could just use the string values directly.
Hi Marco,
I added the above to what you suggested, but it still doesn't work.
Can you please explain why I would be taking "TXT1" as the string, as the info in that field is "s=nul d=0"
I also tried by changing TXT1 to Edit, since that is the string that I am trying to pull into the variable basket. But that still did not work.
I have attached the mq4 file, so that you can test it on your side.
thanks for your help

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi,
I have created an indicator with displays and buttons that change variables, but now I want to create an edit button on the chart.
I have managed to get the button on the chart, but I can't seem to figure out how to get the entry (number) to transfer to my variable.
the button displays and I can enter the number, but it doesn't pass to the variable "Basket"
I enter 10, the Print comes out correctly, but the chart keeps showing 0 and doesn't update the variable.
What am i doing wrong..
I even have included (#include <edit.mqh>)
HELP>