Hi All,
I have been reading everything I can on MQL5 and how Trades are managed compared to MQL4 and have found that it's not a trivial thing to port from one to the other. In this link it states that 'For each account, for every financial instrument, there can be only one position. For each symbol, at any given time, there can be only one open position - long or short.'
However I am able to place multiple trades at the same time, both Long and Short in my EA. The problem comes when trying to select the open trades to ascertain the status. For example I created a simple function in MQL4 that counts all orders with the same Magic number to know how many open trades I have for a given EA at any given moment, it looks like this:
I have been trying combinations of selecting by position without much luck, what would a similar function look like in MQL5?
Once I understand this I should be able to figure out the rest myself, this I thought would be a simple place to start.
Thanks,
Noki0100
Counting positions on the current symbol and only those positions for which Magic Number matches the 'magic number' input parameter
//+------------------------------------------------------------------+ //| CalculateAllPositions.mq5 | //| Copyright © 2018, Vladimir Karputov | //| https://www.mql5.com/ru/market/product/43516 | //+------------------------------------------------------------------+ #property copyright "Copyright © 2020, Vladimir Karputov" #property link "https://www.mql5.com/ru/market/product/43516" #property version "1.001" #property description "Calculate all positions" #include <Trade\PositionInfo.mqh> CPositionInfo m_position; // trade position object //--- input parameters input ulong m_magic=15489; // magic number //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ int OnInit() { //--- //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- Comment(""); } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- int total_position=CalculateAllPositions(); Comment("All position: ",IntegerToString(total_position)); } //+------------------------------------------------------------------+ //| TradeTransaction function | //+------------------------------------------------------------------+ void OnTradeTransaction(const MqlTradeTransaction &trans, const MqlTradeRequest &request, const MqlTradeResult &result) { //--- } //+------------------------------------------------------------------+ //| Calculate all positions | //+------------------------------------------------------------------+ int CalculateAllPositions() { int total=0; for(int i=PositionsTotal()-1; i>=0; i--) if(m_position.SelectByIndex(i)) // selects the position by index for further access to its properties if(m_position.Symbol()==Symbol() && m_position.Magic()==m_magic) total++; //--- return(total); } //+------------------------------------------------------------------+
Thanks, that does indeed do what I ask for. However I am still a bit stuck. I am reading through and gaining more understanding but it's very different from MQL4.
Say I have 2 trades placed at different times.
The first order gets placed creating a deal, this is part of a new position.
The second order gets placed creating a new deal and this is added to my existing position.
So if there is only one position how do I (for example) adjust the stop loss on the first trade without changing the second (or visa versa).
It's how these link together in real time that I am struggling with.
Thanks,
Noki
Thanks, that does indeed do what I ask for. However I am still a bit stuck. I am reading through and gaining more understanding but it's very different from MQL4.
Say I have 2 trades placed at different times.
The first order gets placed creating a deal, this is part of a new position.
The second order gets placed creating a new deal and this is added to my existing position.
So if there is only one position how do I (for example) adjust the stop loss on the first trade without changing the second (or visa versa).
It's how these link together in real time that I am struggling with.
Thanks,
Noki
What type of account: netting or hedging?
OK, but when you say 'go around all positions' but thought there is only one position be market, what is it you mean by that?
I can see that MQLTradeResult returns 'deal' and 'order' id's, do I even need to retain those in that case? I currently keep each order ticket id in my own Struct to keep track of them, previously (in MT4) OrderSend would return the order number (ticket) which I would retain for later usage.
Thinking out loud here: I need to use PositionSelect() to work with my orders. Then use PositionSelectByTicket() to work with the individual items? If so where is I get the Ticket that this function requires? Is it part of the MQLTradeResult?
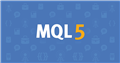
- www.mql5.com
OK, but when you say 'go around all positions' but thought there is only one position be market, what is it you mean by that?
I can see that MQLTradeResult returns 'deal' and 'order' id's, do I even need to retain those in that case? I currently keep each order ticket id in my own Struct to keep track of them, previously (in MT4) OrderSend would return the order number (ticket) which I would retain for later usage.
Thinking out loud here: I need to use PositionSelect() to work with my orders. Then use PositionSelectByTicket() to work with the individual items? If so where is I get the Ticket that this function requires? Is it part of the MQLTradeResult?
Example:
OK I think I have enough to experiment with it now. I always learn best by doing and a bit of trial and error.
Thanks for you help!
PS
I think the main confusion stems from the terms overlapping.
'For each account, for every financial instrument, there can be only one position. For each symbol, at any given time, there can be only one open position - long or short.'
Isn't quite true (at least in all circumstances) it seems.
OK I think I have enough to experiment with it now. I always learn best by doing and a bit of trial and error.
Thanks for you help!
PS
I think the main confusion stems from the terms overlapping.
'For each account, for every financial instrument, there can be only one position . For each symbol, at any given time, there can be only one open position - long or short.'
Isn't quite true (at least in all circumstances) it seems.
There is no mistake. The highlighted is true for NETTING.
Indeed, that's what I mean by overlapping. Context matters, in this case hedging vs netting.
Thanks again!

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hi All,
I have been reading everything I can on MQL5 and how Trades are managed compared to MQL4 and have found that it's not a trivial thing to port from one to the other. In this link it states that 'For each account, for every financial instrument, there can be only one position. For each symbol, at any given time, there can be only one open position - long or short.'
However I am able to place multiple trades at the same time, both Long and Short in my EA. The problem comes when trying to select the open trades to ascertain the status. For example I created a simple function in MQL4 that counts all orders with the same Magic number to know how many open trades I have for a given EA at any given moment, it looks like this:
I have been trying combinations of selecting by position without much luck, what would a similar function look like in MQL5?
Once I understand this I should be able to figure out the rest myself, this I thought would be a simple place to start.
Thanks,
Noki0100