Hello Everyone,
I am new to mql5 and i want to make a ticks counter
so that, each 30 pips i want to make an order (buy or stop buy )
can any one help ? i have searched alot and cannot find a good way of doing this.
Each 30 pips above and below price or every 30 ticks ?
For now, i just want to be able to make a pending order below and above the Ask price with 30 pips.
if the direction is down, i want to make another buy order at the 30 pips below pending order, and so on.
For now, i was able to write the following code :
void OnTick()
{
double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits);
MqlRates PriceInfo[];
ArraySetAsSeries(PriceInfo,true);
int PriceData = CopyRates(_Symbol,_Period,0,3,PriceInfo);
if(PriceInfo[1].close > PriceInfo[1].open)
if(PositionsTotal() == 0 )
{
trade.Buy(0.10,NULL,Ask,0,Ask + 300*_Point,"Buy is done");
}
}
In this way, i am making a pending buy order above 30 pips. is that right ?
currency used : EURUSD
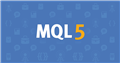
- www.mql5.com
For now, i just want to be able to make a pending order below and above the Ask price with 30 pips.
if the direction is down, i want to make another buy order at the 30 pips below pending order, and so on.
For now, i was able to write the following code :
void OnTick()
{
double Ask = NormalizeDouble(SymbolInfoDouble(_Symbol,SYMBOL_ASK),_Digits);
MqlRates PriceInfo[];
ArraySetAsSeries(PriceInfo,true);
int PriceData = CopyRates(_Symbol,_Period,0,3,PriceInfo);
if(PriceInfo[1].close > PriceInfo[1].open)
if(PositionsTotal() == 0 )
{
trade.Buy(0.10,NULL,Ask,0,Ask + 300*_Point,"Buy is done");
}
}
In this way, i am making a pending buy order above 30 pips. is that right ?
currency used : EURUSD
Great . What do you mean by if direction is down though?
try these structures
//+------------------------------------------------------------------+ //| CustomPending.mq5 | //| Copyright 2020, MetaQuotes Software Corp. | //| https://www.mql5.com | //+------------------------------------------------------------------+ #property copyright "Copyright 2020, MetaQuotes Software Corp." #property link "https://www.mql5.com" #property version "1.00" input int Distance=300;//distance of pendings in points input color buy_default_color=clrDarkBlue;//buy default not-armed color input color buy_armed_color=clrBlue;//buy armed color input color buy_hit_color=clrDodgerBlue;//buy armed and hit color input color sell_default_color=clrDarkRed;//sell default not-armed color input color sell_armed_color=clrRed;//sell armed color input color sell_hit_color=clrOrangeRed;//sell armed and hit color //+------------------------------------------------------------------+ //| Expert initialization function | //+------------------------------------------------------------------+ enum pending_type { pt_void=0,//Void pt_limit=1,//Limit pt_stop=2//Stop }; enum pending_direction { pd_none=0,//None pd_buy=1,//Buy pd_sell=2//Sell }; struct custom_pending { pending_direction Direction; pending_type Type; double Price; bool Armed,Set,Hit,Displayed; string object_name; custom_pending(void){Reset();} ~custom_pending(void){Reset();} void Reset() { if(Displayed){ObjectDelete(ChartID(),object_name);} Armed=false;Set=false;Hit=false;Displayed=false;Price=NULL;Type=pt_void;Direction=pd_none; } //setup void Setup(bool reset, pending_direction direction, pending_type type, double price, string name, bool arm_if_valid, double bid, double ask, datetime time_now) { if(reset) Reset(); Direction=direction; Type=type; Price=price; object_name=name; Set=true; string txt=""; color clr=buy_default_color; bool tag_below=false; if(Direction==pd_buy){txt="Buy ";if(Type==pt_limit){tag_below=true;}} if(Direction==pd_sell){txt="Sell ";clr=sell_default_color;if(Type==pt_stop){tag_below=true;}} if(Type==pt_limit){txt+="Limit ";} if(Type==pt_stop){txt+="Stop ";} txt+=" @["+DoubleToString(Price,Digits())+"]"; CreateHLine(ChartID(),0,object_name,txt,8,Price,clr,1,STYLE_DOT,false,tag_below,time_now); Displayed=true; if(arm_if_valid) { //Buy Stop if(Direction==pd_buy&&Type==pt_stop&&ask<Price) Armed=true; //Buy Limit if(Direction==pd_buy&&Type==pt_limit&&bid>Price) Armed=true; //Sell Stop if(Direction==pd_sell&&Type==pt_stop&&bid>Price) Armed=true; //Sell Limit if(Direction==pd_sell&&Type==pt_limit&&ask<Price) Armed=true; if(Armed) { if(Direction==pd_buy) { UpdateHline(ChartID(),object_name,Price,buy_armed_color,time_now); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,buy_armed_color); } if(Direction==pd_sell) { UpdateHline(ChartID(),object_name,Price,sell_armed_color,time_now); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,sell_armed_color); } } } } //maintain void Maintain(double bid,double ask,datetime time) { if(Set) { //not armed not hit - check for arming if(!Armed&&!Hit) { //Buy Stop if(Direction==pd_buy&&Type==pt_stop&&ask<Price) Armed=true; //Buy Limit if(Direction==pd_buy&&Type==pt_limit&&bid>Price) Armed=true; //Sell Stop if(Direction==pd_sell&&Type==pt_stop&&bid>Price) Armed=true; //Sell Limit if(Direction==pd_sell&&Type==pt_limit&&ask<Price) Armed=true; if(Armed) { if(Direction==pd_buy) { UpdateHline(ChartID(),object_name,Price,buy_armed_color,TimeCurrent()); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,buy_armed_color); } if(Direction==pd_sell) { UpdateHline(ChartID(),object_name,Price,sell_armed_color,TimeCurrent()); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,sell_armed_color); } ChartRedraw(ChartID()); } } //armed check for hit if(Armed&&!Hit) { //Buy Stop if(Direction==pd_buy&&Type==pt_stop&&ask>=Price) Hit=true; //Buy Limit if(Direction==pd_buy&&Type==pt_limit&&ask<=Price) Hit=true; //Sell Stop if(Direction==pd_sell&&Type==pt_stop&&bid<=Price) Hit=true; //Sell Limit if(Direction==pd_sell&&Type==pt_limit&&bid>=Price) Hit=true; if(Hit) { if(Direction==pd_buy) { UpdateHline(ChartID(),object_name,Price,buy_hit_color,TimeCurrent()); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,buy_hit_color); } if(Direction==pd_sell) { UpdateHline(ChartID(),object_name,Price,sell_hit_color,TimeCurrent()); ObjectSetInteger(ChartID(),object_name+"_LABEL",OBJPROP_COLOR,sell_hit_color); } ChartRedraw(ChartID()); } } } } }; custom_pending PendingBuy,PendingSell; bool SystemSet=false; double actual_distance=0,point=0; int OnInit() { //--- SystemSet=false; double bid=0,ask=0; datetime time=TimeCurrent(); bool gotAsk=SymbolInfoDouble(Symbol(),SYMBOL_ASK,ask); bool gotBid=SymbolInfoDouble(Symbol(),SYMBOL_BID,bid); bool gotPoint=SymbolInfoDouble(Symbol(),SYMBOL_POINT,point); if(gotAsk&&gotBid&&gotPoint) { actual_distance=point*((double)Distance); double buy_pending_price=bid+actual_distance; double sell_pending_price=ask-actual_distance; PendingBuy.Setup(true,pd_buy,pt_stop,buy_pending_price,"buy_pending",true,bid,ask,time); PendingSell.Setup(true,pd_sell,pt_stop,sell_pending_price,"sell_pending",true,bid,ask,time); SystemSet=true; } //--- return(INIT_SUCCEEDED); } //+------------------------------------------------------------------+ //| Expert deinitialization function | //+------------------------------------------------------------------+ void OnDeinit(const int reason) { //--- } //+------------------------------------------------------------------+ //| Expert tick function | //+------------------------------------------------------------------+ void OnTick() { //--- double bid=0,ask=0; datetime time=TimeCurrent(); bool gotAsk=SymbolInfoDouble(Symbol(),SYMBOL_ASK,ask); bool gotBid=SymbolInfoDouble(Symbol(),SYMBOL_BID,bid); //if system is set if(SystemSet) { if(gotAsk&&gotBid) { PendingBuy.Maintain(bid,ask,time); PendingSell.Maintain(bid,ask,time); } } //if system is not set if(!SystemSet) { bool gotPoint=SymbolInfoDouble(Symbol(),SYMBOL_POINT,point); if(gotAsk&&gotBid&&gotPoint) { actual_distance=point*((double)Distance); double buy_pending_price=bid+actual_distance; double sell_pending_price=ask-actual_distance; PendingBuy.Setup(true,pd_buy,pt_stop,buy_pending_price,"buy_pending",true,bid,ask,time); PendingSell.Setup(true,pd_sell,pt_stop,sell_pending_price,"sell_pending",true,bid,ask,time); SystemSet=true; } } //--- } //+------------------------------------------------------------------+ //| ChartEvent function | //+------------------------------------------------------------------+ void OnChartEvent(const int id, const long &lparam, const double &dparam, const string &sparam) { //--- } //+------------------------------------------------------------------+ void CreateHLine(long cid,int subw,string name,string label,int label_fs,double price,color col,int wid,ENUM_LINE_STYLE style,bool selectable,bool tag_below_line,datetime label_time) { bool obji=ObjectCreate(cid,name,OBJ_HLINE,subw,0,price); ObjectSetInteger(cid,name,OBJPROP_WIDTH,wid); ObjectSetInteger(cid,name,OBJPROP_COLOR,col); ObjectSetInteger(cid,name,OBJPROP_BACK,true); ObjectSetInteger(cid,name,OBJPROP_STYLE,style); ObjectSetInteger(cid,name,OBJPROP_SELECTABLE,selectable); obji=ObjectCreate(cid,name+"_LABEL",OBJ_TEXT,subw,label_time,price); ObjectSetString(cid,name+"_LABEL",OBJPROP_TEXT,label); ObjectSetInteger(cid,name+"_LABEL",OBJPROP_COLOR,col); ObjectSetInteger(cid,name+"_LABEL",OBJPROP_ALIGN,ALIGN_RIGHT); if(!tag_below_line) ObjectSetInteger(cid,name+"_LABEL",OBJPROP_ANCHOR,ANCHOR_RIGHT_LOWER); if(tag_below_line) ObjectSetInteger(cid,name+"_LABEL",OBJPROP_ANCHOR,ANCHOR_RIGHT_UPPER); ObjectSetInteger(cid,name+"_LABEL",OBJPROP_FONTSIZE,label_fs); ChartRedraw(ChartID()); } void UpdateHline(long cid,string name,double price,color col,datetime label_time) { ObjectSetDouble(cid,name,OBJPROP_PRICE,price); ObjectSetInteger(cid,name,OBJPROP_COLOR,col); ObjectSetDouble(cid,name+"_LABEL",OBJPROP_PRICE,price); ObjectSetInteger(cid,name,OBJPROP_TIME,label_time); ObjectSetInteger(cid,name+"_LABEL",OBJPROP_COLOR,col); }
I mean if price is descending (Direction down)
or ascending (Direction up)
Thank you very much !! This is very interesting .
Can you please explain somehow your code ? I would be very grateful.
Thanks in advance

- Free trading apps
- Over 8,000 signals for copying
- Economic news for exploring financial markets
You agree to website policy and terms of use
Hello Everyone,
I am new to mql5 and i want to make a ticks counter
so that, each 30 pips i want to make an order (buy or stop buy )
can any one help ? i have searched alot and cannot find a good way of doing this.